Understanding Side Effects in Lambda Functions
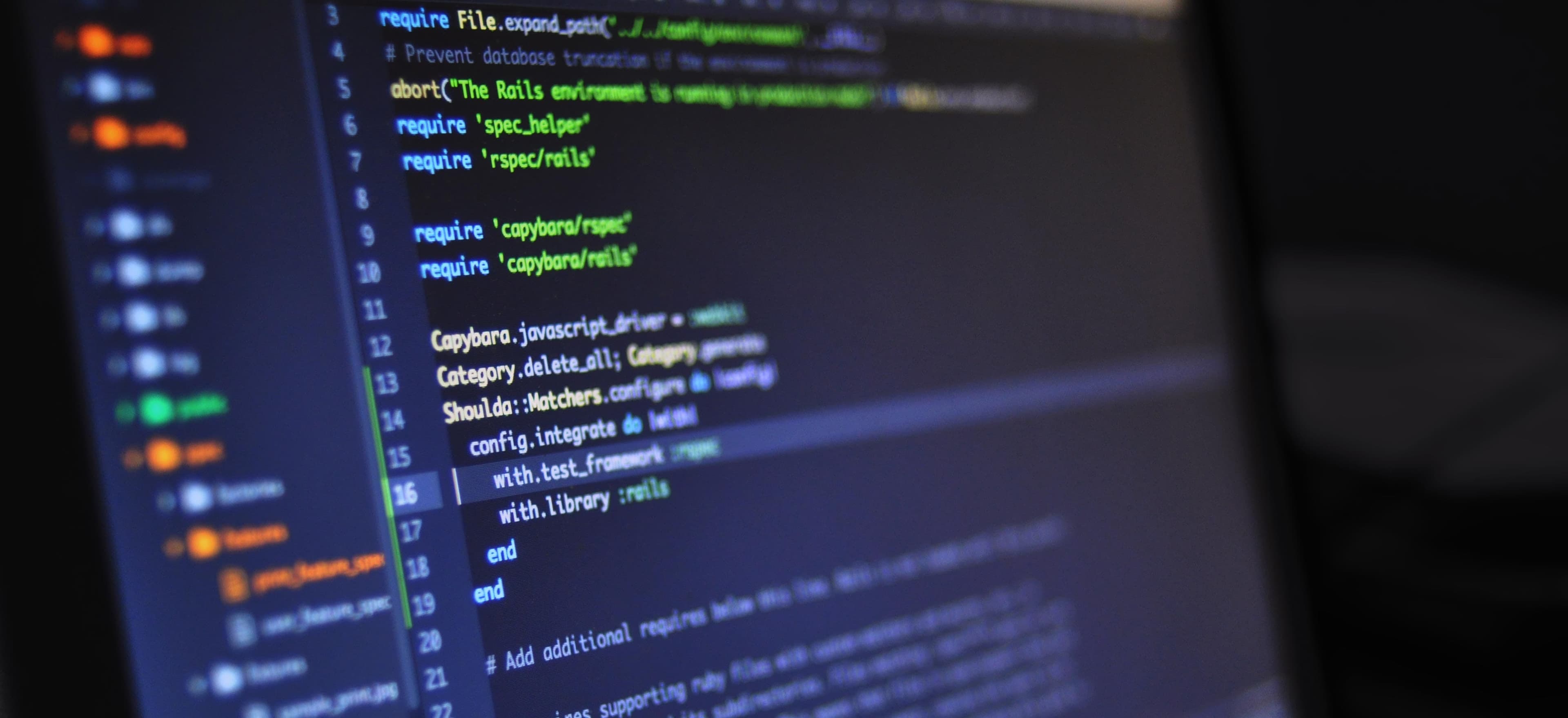
- Published on
Understanding Side Effects in Lambda Functions
In functional programming, the concept of side effects is a pivotal topic. It becomes especially relevant when discussing aspects of programming languages like Java, where lambda functions are prevalent. This blog post will unravel the nuances of side effects in lambda functions, offering insights into why they are significant and how to manage them effectively.
What are Lambda Functions?
Lambda functions in Java were introduced in Java 8, allowing developers to write concise, anonymous functions. These functions can be used primarily to define the behavior of instances of functional interfaces. A functional interface is an interface that contains a single abstract method.
Here's a simple example of a lambda function:
import java.util.Arrays;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using a lambda function to print each name
names.forEach(name -> System.out.println(name));
}
}
In this example, the lambda expression name -> System.out.println(name)
serves to iterate through the list of names and print them.
Understanding Side Effects
In programming, a side effect refers to any operation that affects the state of the system outside the function being executed. Modifying a variable, writing to a file, or making a network request can all be categorized as side effects.
Why are Side Effects Important?
Side effects can impact code readability, maintainability, and predictability. When a function has side effects, its behavior can depend on the external state, making it harder to reason through the code. Understanding when side effects occur—and managing them correctly—allows developers to create cleaner and more maintainable code.
Side Effects in Lambda Functions
When using lambda functions in Java, side effects can become a point of contention, particularly in concurrent programming and functional paradigms. While lambda expressions promote immutability and statelessness, allowing developers to produce cleaner code, they also present challenges when you inadvertently introduce side effects.
Example of a Side Effect in a Lambda
Consider the following code snippet, which introduces a side effect by modifying an external variable:
import java.util.Arrays;
import java.util.List;
public class SideEffectExample {
static int counter = 0; // External state
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Modifying external state within the lambda
names.forEach(name -> {
counter++; // Side effect: Updating external state
System.out.println(name);
});
System.out.println("Total names processed: " + counter);
}
}
In this code, we maintain a static variable counter
, which keeps track of the number of names processed. The lambda function modifies this external state, which constitutes a side effect.
Why Avoid Side Effects?
-
Readability: When side effects are prevalent in your code, understanding what a function does becomes more complex. It can make extensive debugging sessions necessary to figure out why your code isn't working as expected.
-
Testing: Functions with side effects are harder to test since their behavior may depend on and affect external states. This can lead to flaky tests, where inconsistent results are produced based on the variable states.
-
Concurrency and Multithreading: In a concurrent environment, side effects can lead to unpredictable behavior when multiple threads try to modify shared states. This can result in race conditions, deadlocks, and other issues.
To read more about functional programming principles and best practices, visit Effective Java.
Managing Side Effects
While it may not be entirely possible to eliminate side effects, managing them is crucial. Here are some strategies to effectively deal with side effects in lambda functions:
1. Use Local Variables
Limit the use of external variables within lambda expressions. Instead, prefer local, immutable variables whenever possible. This promotes statelessness and reduces the likelihood of unintended side effects.
import java.util.Arrays;
import java.util.List;
public class ManageSideEffectExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
int[] counter = {0}; // Using an array to simulate immutability
names.forEach(name -> {
counter[0]++; // Localized counter to manage side effect
System.out.println(name);
});
System.out.println("Total names processed: " + counter[0]);
}
}
2. Functional Interfaces and Pure Functions
Utilizing functional interfaces and aiming for pure functions (where the output is determined solely by the input, without causing side effects) can simplify code. If your lambda functions are pure, they will enhance trust and clarity in your codebase.
3. Pure Methods
Always prefer writing methods that do not alter the states of external variables. Return new states rather than mutating existing ones. For instance, the Stream API in Java encourages this approach:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> uppercaseNames = names.stream()
.map(String::toUpperCase) // Pure function
.collect(Collectors.toList());
System.out.println(uppercaseNames);
}
}
To Wrap Things Up
Understanding side effects in lambda functions is crucial for any developer working in Java today. Although it may seem tempting to leverage side effects for occasional convenience, the long-term costs can outweigh the short-term gains. By adhering to best practices—like using local variables, creating pure functions, and leveraging functional interfaces—you can craft cleaner, more maintainable code that is easier to test and debug.
Would you like to delve deeper into functional programming principles? Check out Java 8 in Action for further insights. As we explore more about lambda expressions and their impact, remember that mastering the nuances of side effects will empower you to write better, more reliable Java code.