Boost Minecraft Performance with Off-Heap Memory Tips!
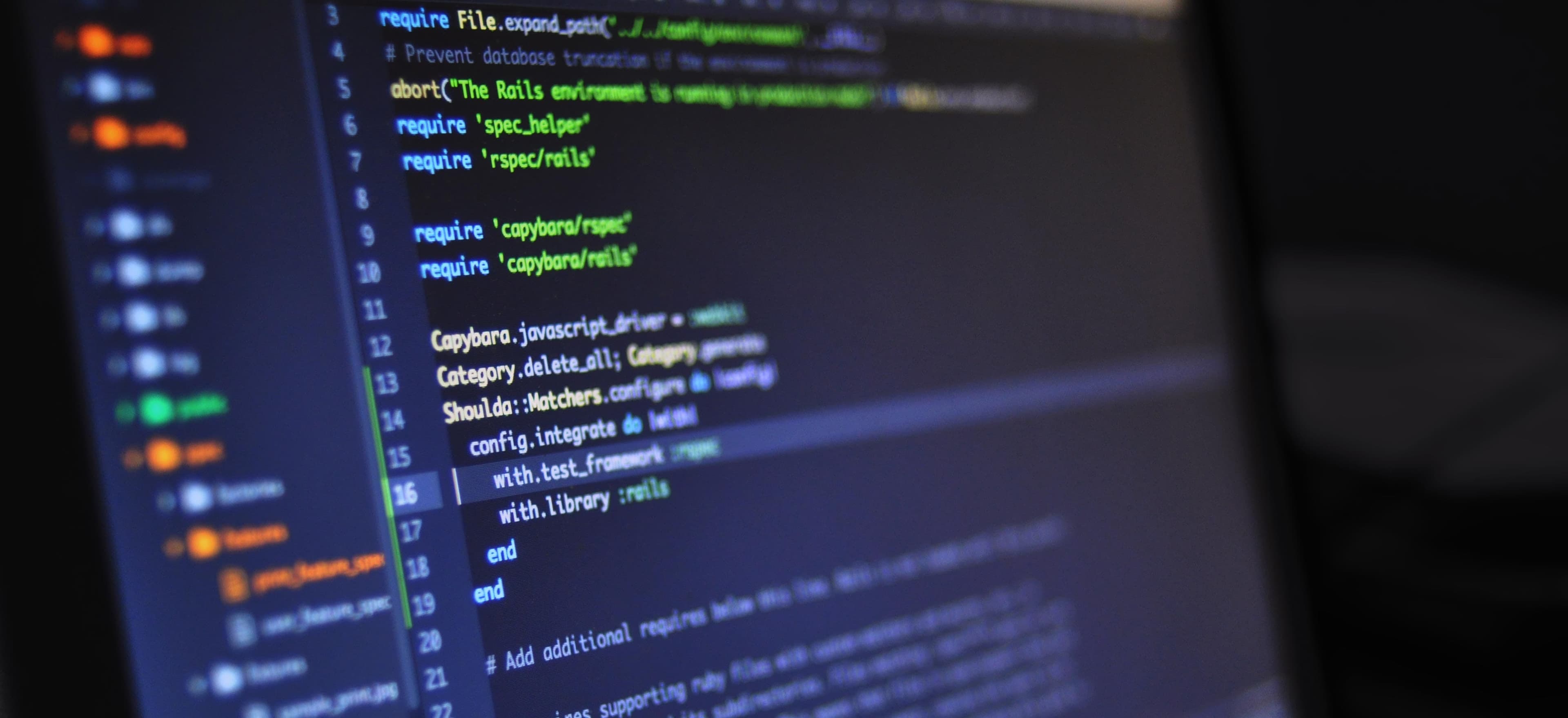
- Published on
Boost Minecraft Performance with Off-Heap Memory Tips
Minecraft, a game that has captured the hearts of millions, often faces performance issues as worlds grow larger and more players join. With increasing demands on system resources, optimizing game performance becomes essential. One such optimization technique is the utilization of off-heap memory. In this blog post, we’ll explore what off-heap memory is, how it works in the context of Minecraft, and provide actionable tips to enhance your gaming experience.
What is Off-Heap Memory?
Off-heap memory refers to memory that is managed outside of the Java Virtual Machine (JVM) heap. Unlike heap memory, which is managed automatically by the JVM's garbage collector, off-heap memory is allocated directly and can offer significant performance benefits, especially for applications with large datasets.
In the case of Minecraft, players often utilize mods, plugins, and world data that can lead to increased memory usage. Here’s where off-heap memory becomes crucial. It allows developers to allocate memory for heavy data structures more efficiently, reducing the overhead of garbage collection and improving performance.
Why Use Off-Heap Memory in Minecraft
- Reduced Garbage Collection Overhead: Off-heap memory doesn't go through the JVM's garbage collector, resulting in fewer pauses and smoother gameplay.
- Increased Performance: By managing memory explicitly, developers can optimize custom memory usage patterns, resulting in better performance during peak loads.
- Enhanced Memory Efficiency: As mods and plugins continue to add data to Minecraft, using off-heap memory can help juggle the memory needs of these additions without crashing the server.
Key Concepts
Before diving into implementation, let’s understand some crucial concepts:
- Direct Byte Buffers: Java provides
java.nio.ByteBuffer
for working with off-heap memory. This class allows for the allocation of memory outside the JVM’s heap and can be more efficient for game development. - Memory Mapped Files: Utilized for accessing large files, memory-mapped files can also store game data, allowing faster access than traditional file I/O.
- Native Memory Management: Implementing manual allocation and deallocation of memory can be riskier due to potential memory leaks but can yield performance gains when done correctly.
Implementing Off-Heap Memory in Minecraft
Now that we understand the theory, let's jump to the practical side. Below is a simple implementation using Java’s ByteBuffer
to allocate off-heap memory for storing player-related data.
Step 1: Allocating Off-Heap Memory
Let’s start by allocating a ByteBuffer
as off-heap memory.
import java.nio.ByteBuffer;
public class OffHeapMemoryExample {
private static final int BUFFER_SIZE = 1024;
private ByteBuffer offHeapBuffer;
public OffHeapMemoryExample() {
// Allocate direct off-heap memory
offHeapBuffer = ByteBuffer.allocateDirect(BUFFER_SIZE);
}
public void setPlayerPosition(int playerId, float x, float y, float z) {
// Calculate offset for playerId
int offset = playerId * 12; // 3 float values (x, y, z)
offHeapBuffer.putFloat(offset, x);
offHeapBuffer.putFloat(offset + 4, y);
offHeapBuffer.putFloat(offset + 8, z);
}
public float[] getPlayerPosition(int playerId) {
int offset = playerId * 12;
float x = offHeapBuffer.getFloat(offset);
float y = offHeapBuffer.getFloat(offset + 4);
float z = offHeapBuffer.getFloat(offset + 8);
return new float[]{x, y, z};
}
}
Explanation
- ByteBuffer.allocateDirect(...): This method allocates memory outside the JVM heap. It's faster for high-throughput applications.
- putFloat(...) and getFloat(...): These methods are used for writing and reading float values efficiently.
You can expand this example by creating more complex data structures for other player attributes or game elements to utilize the advantages of off-heap memory fully.
Step 2: Use Memory Mapped Files
If you deal with large datasets exceeding the memory capacity, consider using memory-mapped files. Here’s a brief example:
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
public class MemoryMappedFileExample {
private static final int FILE_SIZE = 1024 * 1024; // 1MB
public static void main(String[] args) {
try (RandomAccessFile memoryMappedFile = new RandomAccessFile("players.dat", "rw")) {
FileChannel channel = memoryMappedFile.getChannel();
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_WRITE, 0, FILE_SIZE);
// Write data
buffer.putInt(0, 42); // example data
// Read data
int playerScore = buffer.getInt(0);
System.out.println("Player Score: " + playerScore);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- MappedByteBuffer: It allows you to treat a slice of the file as an array in memory, providing fast read/write access.
- channel.map(...): This opens a file channel and maps it for read-write operations.
Best Practices for Off-Heap Memory Management
- Monitor Memory Usage: Regularly monitor the allocated off-heap memory to avoid memory leaks.
- Deallocate Resources: When no longer needed, release allocated memory (if manually controlled) to prevent wastage.
- Tune Performance: Experiment with various sizes of off-heap allocations to find the balance that fits your server load.
Closing Remarks
Using off-heap memory in Minecraft can significantly improve performance, especially as your worlds expand or with the inclusion of numerous mods and plugins. By understanding and implementing strategies like direct byte buffers and memory-mapped files, you can enhance your gaming experience while ensuring a smoother, more reliable performance.
For more in-depth reading on memory management and Java performance optimization, check out the following resources:
- Java Performance Tuning
- Understanding Off-Heap Memory
- Java NIO Documentation
Making targeted improvements to your Minecraft server's memory management will not only allow for optimal gameplay but also prepare it for future expansion. Happy crafting!