Common TDD Pitfalls That Delay App Releases
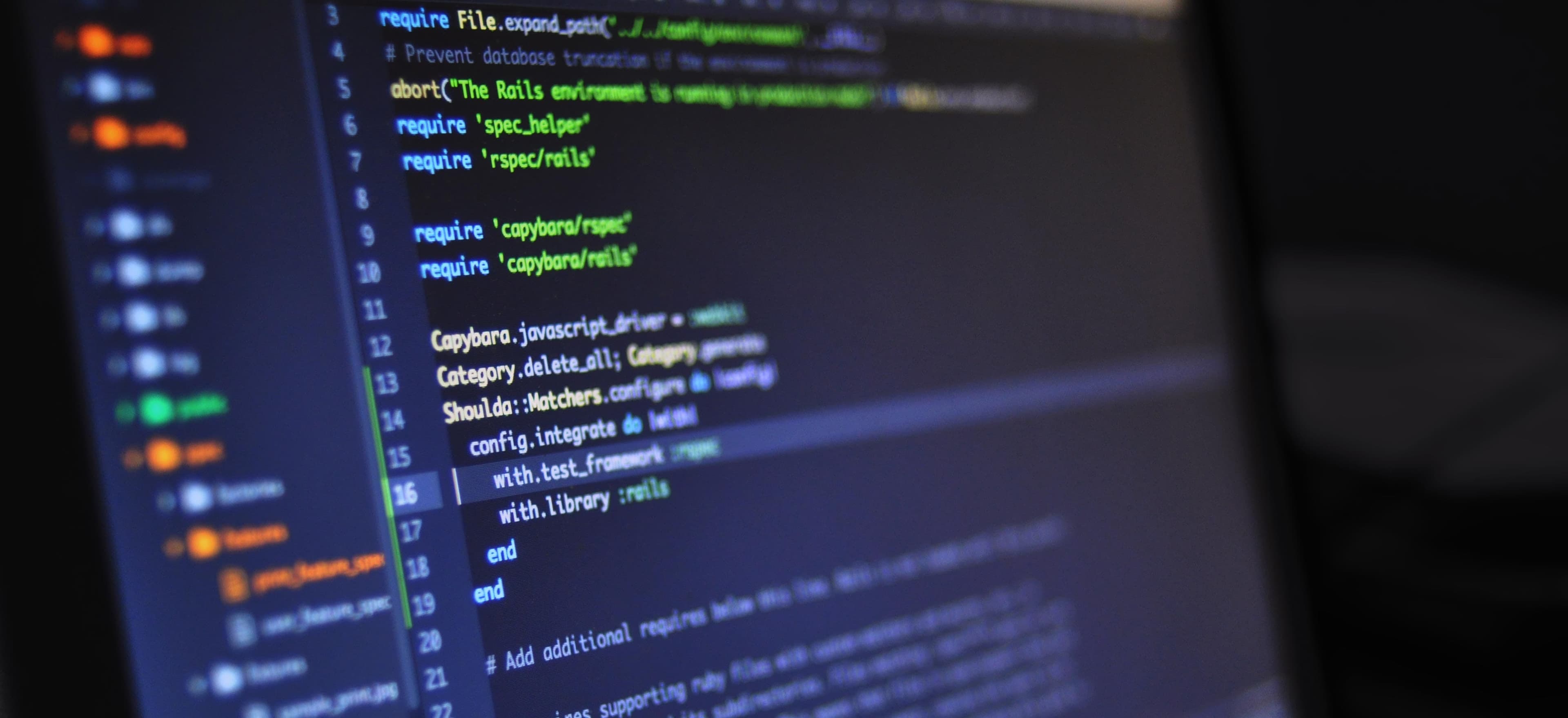
- Published on
Common TDD Pitfalls That Delay App Releases
Test-Driven Development (TDD) is a software development process emphasizing the creation of tests before writing the actual application code. While TDD promotes high-quality, maintainable code, developers can sometimes fall into common pitfalls that slow down the release cycle. This blog post will explore these pitfalls in detail, offering insights and practical examples to help you navigate away from them effectively.
Understanding TDD
Before diving into common pitfalls, let's briefly clarify what TDD entails. The TDD cycle typically follows three steps:
- Write a Test: Before writing the actual code, developers write a test that defines a function or improvements.
- Run the Test: At this stage, the newly written test should fail because the functionality has not been implemented yet.
- Write the Code: Only enough code to pass the test is added.
- Refactor: The code is restructured to meet standards without changing its external behavior.
Example TDD Cycle
Let's consider a simple example where we want to implement a method that calculates the factorial of a number.
// The test case that defines our expected behavior
@Test
public void testFactorial() {
FactorialCalculator calculator = new FactorialCalculator();
assertEquals(120, calculator.factorial(5));
}
In this code snippet, we write a test first, which defines that calculating the factorial of 5 should return 120. This test will fail until we implement the factorial
method.
public class FactorialCalculator {
public int factorial(int n) {
if (n == 0) return 1;
return n * factorial(n - 1);
}
}
Once the method is written, we can rerun the test, and it should pass successfully, confirming our implementation fulfills the defined behavior.
Common TDD Pitfalls
While TDD provides a structured approach to development, certain pitfalls can cause delays in app releases. Here are some of the most common ones:
1. Incomplete Test Coverage
One of the best practices in TDD is ensuring all functionalities are covered by tests. However, developers often skip writing tests for edge cases or specific scenarios due to time constraints. This incomplete test coverage can lead to bugs surfacing post-release, causing emergency fixes and delays.
How to Avoid It
- Make it a rule to cover both typical scenarios and edge cases.
- Utilize code coverage tools like JaCoCo or Clover to gauge your test coverage.
2. Writing Tests After Implementation
An obvious trap is the intention to implement functionality first and write tests later. This will defeat the TDD approach, leading to potential bugs and a lack of comprehensive testing.
Example Modification
Suppose our original example was modified post-implementation:
// Implemented without TDD
public int factorial(int n) {
// Logic goes here
}
Adopting TDD encourages structured development, making the code easier to test and maintain.
3. Over-engineering Tests
Another common issue is writing overly complex or verbose tests that are difficult to maintain. While it’s essential to practice good testing strategies, there's no need to complicate what should be straightforward.
To Align Your Tests
- Keep your tests simple. Each test should focus on a single aspect of functionality.
- Stick to clear naming conventions for methods to enhance readability.
4. Ignoring Refactoring
Refactoring is an integral part of TDD. Some developers might view refactoring as a nuisance and ignore it completely or do it poorly. However, failure to refactor can lead to technical debt that complicates future implementations or adjustments.
Continuous Refactoring Strategy
- Make refactoring part of your development cycle. Re-evaluate your tests and code regularly.
- Always ensure tests are passing after refactoring.
5. Not Practicing Incremental Development
A common pitfall of TDD is the tendency to tackle large pressure-driven tasks. Developers often ignore incremental development, leading to feature bloat and missed deadlines.
Incremental Approach Benefits
- Break down features into manageable tasks.
- Immediately write the necessary tests for each task.
6. Poorly Written Tests
Writing effective tests can significantly prevent delays during app releases. Tests that are not well-thought-out can yield incorrect results or are overly complicated to follow, which can derail the TDD process.
Tips for Writing Effective Tests
- Aim for clarity. Your tests should communicate what they intend to verify clearly.
- Utilize mocking frameworks like Mockito to isolate tests from external dependencies effectively.
The Closing Argument
Understanding and navigating common TDD pitfalls is essential for speeding up app releases and ensuring software quality. By recognizing incomplete test coverage, avoiding implementation-first strategies, and implementing simple and effective tests, developers can benefit from a streamlined development process.
As developers, it’s crucial to maintain discipline in the TDD process. Always remember: a well-structured approach saves time and reduces stress in the long run.
Useful Resources
If you're looking to dive deeper into TDD and its best practices, check out these excellent resources:
- Test-Driven Development by Example by Kent Beck
- JUnit 5 User Guide for testing in Java
- Mockito Documentation for effective Java testing
With a clear understanding of these common pitfalls, developers can develop more efficiently and lead their teams to faster release cycles. Happy coding and testing!