QueryDSL vs JPA Criteria: Choosing the Right Query Approach
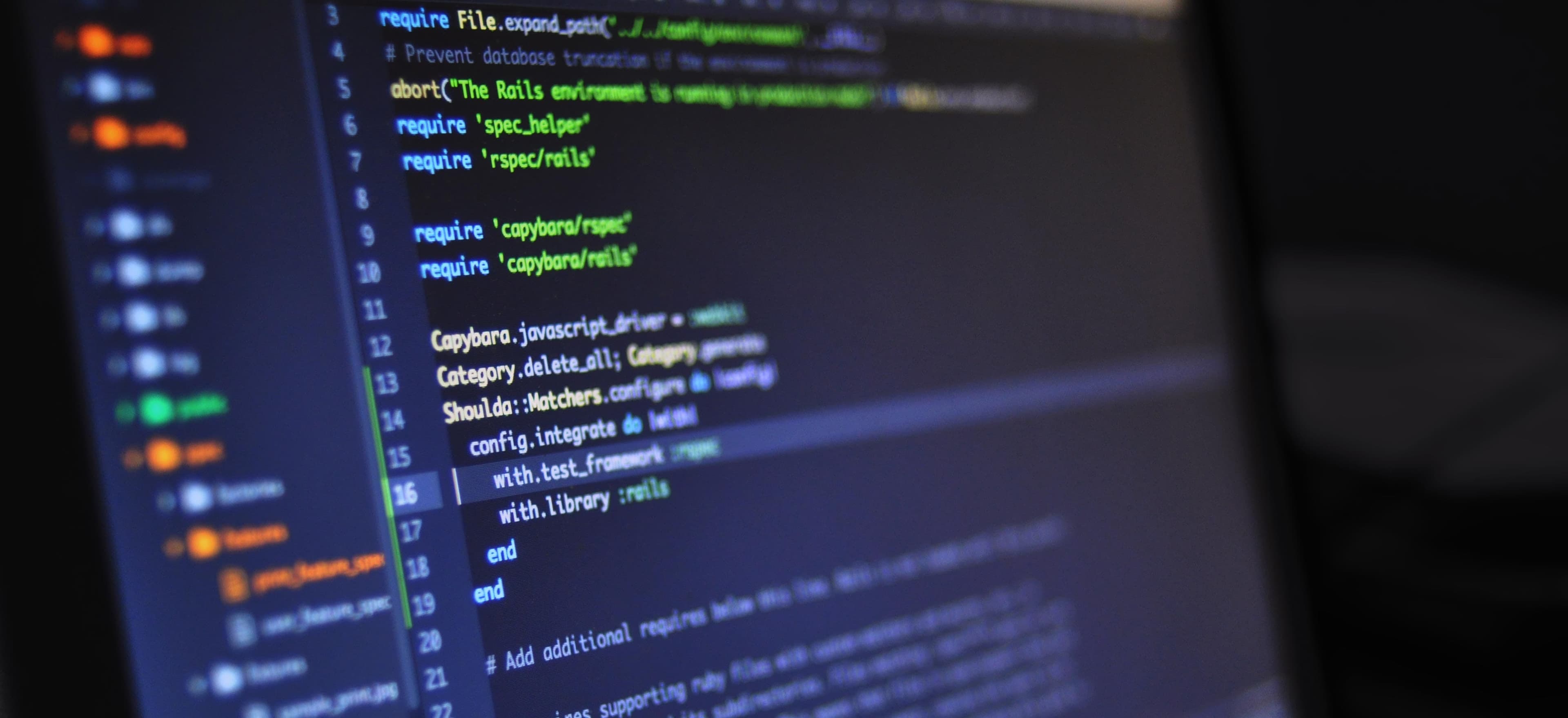
- Published on
QueryDSL vs JPA Criteria: Choosing the Right Query Approach
When developing enterprise applications in Java, choosing the right tool for querying data is crucial. Two popular options, QueryDSL and JPA Criteria, each offer unique advantages and cater to different programming preferences. This blog post dives into the core differences between these two approaches and helps you navigate the decision-making process.
Understanding JPA Criteria
Java Persistence API (JPA) provides a standard way to access data using Java objects. The Criteria API allows for constructing type-safe queries in a programmatic way. This significantly increases the capability to build dynamic queries based on various input conditions.
Code Example of JPA Criteria
Here’s a simple example that demonstrates how to use JPA Criteria to fetch users from a database.
import javax.persistence.*;
import javax.persistence.criteria.*;
import java.util.List;
public List<User> getActiveUsers(EntityManager entityManager) {
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<User> criteriaQuery = criteriaBuilder.createQuery(User.class);
Root<User> userRoot = criteriaQuery.from(User.class);
criteriaQuery.select(userRoot)
.where(criteriaBuilder.equal(userRoot.get("status"), "ACTIVE"));
TypedQuery<User> query = entityManager.createQuery(criteriaQuery);
return query.getResultList();
}
Why This Approach?
- Type Safety: The JPA Criteria API utilizes Java's type system to prevent incorrect queries at compile time.
- Dynamic Query Building: You can easily construct queries based on specific criteria, without hardcoding any SQL.
- Integration: Since it's integrated within JPA, there’s no need for additional libraries.
However, JPA Criteria can become verbose and hard to read, especially with complex queries.
Understanding QueryDSL
QueryDSL, unlike JPA Criteria, is a separate framework that brings a more fluent API for constructing type-safe queries. It is geared towards simplicity and usability, and it supports various backends, such as JPA, SQL, and others.
Code Example of QueryDSL
Below is an example of querying active users using QueryDSL.
import com.querydsl.jpa.impl.JPAQuery;
import com.querydsl.core.types.dsl.BooleanExpression;
public List<User> getActiveUsers(EntityManager entityManager) {
QUser user = QUser.user; // QType generated from the User entity
JPAQuery<User> query = new JPAQuery<>(entityManager);
BooleanExpression isActive = user.status.eq("ACTIVE");
return query.select(user)
.from(user)
.where(isActive)
.fetch();
}
Why This Approach?
- Conciseness: QueryDSL provides a more concise and readable syntax than JPA Criteria, which can enhance maintainability.
- Fluid API: The fluent style allows you to chain methods in a way that feels like a natural syntax for Java developers.
- Better Error Checking: As you write your queries, the IDE can provide better auto-completion and type-checking because QueryDSL is based on generated Q-types.
Despite its advantages, QueryDSL requires an additional setup for code generation, which can be seen as overhead for small projects.
Key Differences
While both JPA Criteria and QueryDSL offer type-safe querying, there are fundamental differences that can guide your choice:
1. Verbosity vs. Readability
- JPA Criteria can become verbose, especially with complex queries.
- QueryDSL offers a more readable and less verbose syntax.
2. Learning Curve
- JPA Criteria is part of JPA, so if you're already familiar with it, there's less to learn.
- QueryDSL has its nuances and you'll need to understand its code generation concept.
3. Flexibility
- JPA Criteria is tightly integrated with JPA specifications, which may limit its use outside JPA contexts.
- QueryDSL supports multiple backends and query types, giving you flexibility in your choice of technology stack.
4. IDE Support
- QueryDSL has better auto-completion and type-checking capabilities in IDEs due to its generated Q-types.
- While JPA Criteria is type-safe, it doesn't leverage IDE capabilities as effectively as QueryDSL.
When to Choose JPA Criteria
You might choose JPA Criteria if:
- You are heavily invested in JPA and need simple query capabilities.
- Your application has relatively straightforward querying needs.
- You prefer keeping external dependencies to a minimum.
When to Choose QueryDSL
On the other hand, consider QueryDSL if:
- You are building complex applications that require a lot of dynamic queries.
- You value code readability and maintainability.
- You are already using or plan to use multiple querying paradigms (like SQL or NoSQL).
Key Takeaways
Both QueryDSL and JPA Criteria have their pros and cons, and the right choice depends on your project requirements and team preference. If clarity and flexibility are your top priorities, QueryDSL might be the way to go. However, if you are looking for modest querying capabilities tied closely with JPA, then the JPA Criteria API stands tall.
To sum it up, understanding your project’s needs is essential for making an informed choice. We encourage you to explore QueryDSL Documentation and JPA Criteria API Overview for further information.
By carefully weighing these factors, you’ll be better positioned to select the most appropriate query approach for your Java applications. Happy coding!