Mastering the Abstract Factory Pattern: Common Pitfalls to Avoid
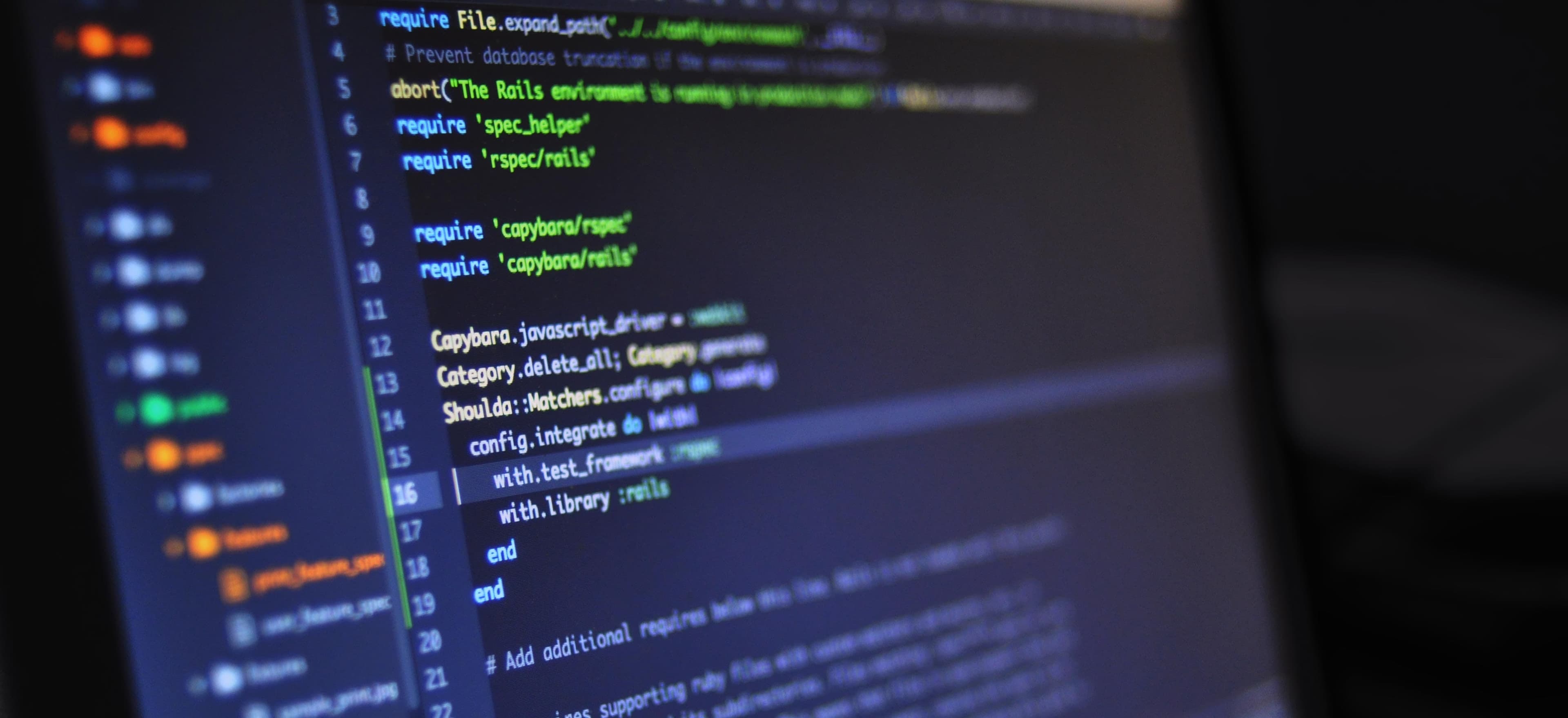
- Published on
Mastering the Abstract Factory Pattern: Common Pitfalls to Avoid
The Abstract Factory Pattern is one of the creational design patterns in software engineering that allows you to create families of related or dependent objects without specifying their concrete classes. It’s a powerful tool, but like any tool in a developer's toolkit, it has its pitfalls. This blog post will delve into the Abstract Factory Pattern, its usage, and, crucially, the common pitfalls you should avoid when implementing this pattern in your Java applications.
What is the Abstract Factory Pattern?
The Abstract Factory Pattern provides a way to encapsulate a group of individual factories that have a common theme. Each factory can produce a set of related products. The consumer code, which relies on these factories, does not need to know the specifics of the classes it is interacting with, promoting loose coupling.
Key Components
- Abstract Factory: An interface for creating abstract products.
- Concrete Factory: Implementations of the abstract factory for creating concrete products.
- Abstract Product: An interface for a type of product.
- Concrete Product: Implementations of various products.
Example Overview
Let’s imagine a scenario where we have two types of User Interfaces: Windows and Mac. We’ll create factories that are responsible for creating buttons and text fields for each UI type.
Example Implementation
Abstract Product Interfaces
// Abstract Product - Button
public interface Button {
void paint();
}
// Abstract Product - TextField
public interface TextField {
void render();
}
Concrete Products
// Concrete Product - Windows Button
public class WindowsButton implements Button {
@Override
public void paint() {
System.out.println("Rendering a button in Windows style.");
}
}
// Concrete Product - Mac Button
public class MacButton implements Button {
@Override
public void paint() {
System.out.println("Rendering a button in macOS style.");
}
}
// Concrete Product - Windows TextField
public class WindowsTextField implements TextField {
@Override
public void render() {
System.out.println("Rendering a text field in Windows style.");
}
}
// Concrete Product - Mac TextField
public class MacTextField implements TextField {
@Override
public void render() {
System.out.println("Rendering a text field in macOS style.");
}
}
Abstract Factory Interface
// Abstract Factory
public interface GUIFactory {
Button createButton();
TextField createTextField();
}
Concrete Factories
// Concrete Factory - Windows Factory
public class WindowsFactory implements GUIFactory {
@Override
public Button createButton() {
return new WindowsButton();
}
@Override
public TextField createTextField() {
return new WindowsTextField();
}
}
// Concrete Factory - Mac Factory
public class MacFactory implements GUIFactory {
@Override
public Button createButton() {
return new MacButton();
}
@Override
public TextField createTextField() {
return new MacTextField();
}
}
Client Code
public class Application {
private Button button;
private TextField textField;
public Application(GUIFactory factory) {
button = factory.createButton();
textField = factory.createTextField();
}
public void paint() {
button.paint();
textField.render();
}
}
Factory Selector
public class Main {
public static void main(String[] args) {
GUIFactory factory;
String osType = "WINDOWS"; // This can be dynamic based on the system.
if (osType.equals("WINDOWS")) {
factory = new WindowsFactory();
} else {
factory = new MacFactory();
}
Application app = new Application(factory);
app.paint();
}
}
Here's how this code works:
- The Application class consumes the GUIFactory which allows it to remain flexible and decoupled from the concrete implementations of the products.
- Depending on the detected operating system, a proper factory gets created, which in turn builds the appropriate button and text field.
Common Pitfalls to Avoid
While the Abstract Factory Pattern offers flexibility and encapsulation, there are several pitfalls developers should be wary of.
1. Overuse of the Pattern
The Abstract Factory Pattern can add unnecessary complexity if used in situations that do not require it. Some developers might apply it just for the sake of using design patterns or when simpler solutions exist.
When to Avoid: If there are not multiple families of related objects in your project, consider using simpler patterns like the Factory Method.
2. Not Following the Open/Closed Principle
The Open/Closed Principle states that classes should be open for extension but closed for modification. If your factories or products require modifying existing code for new implementations, you may not be adhering to this principle.
Solution: Use interfaces and follow the factory pattern's structure to introduce new products without editing existing factory classes.
3. Ignoring Dependency Injection
Implementing the Abstract Factory Pattern without properly using dependency injection can lead to tightly coupled classes. Always aim to inject the factory into your classes that need it, instead of instantiating the factory directly.
Example:
public Application(GUIFactory factory) {
// This keeps the Application class decoupled from the factory's implementations.
...
}
4. Lack of Cohesion
Each factory should be coherent and responsible for creating a specific set of products. Mixing products from different families in the same factory can lead to confusion and violate the intent of the Abstract Factory Pattern.
Recommendation: Keep each concrete factory focused on creating products for a specific theme or family.
5. Poor Naming Conventions
Names are crucial in maintaining readability. If factory or product names do not convey the specific design purpose, it can lead to confusion down the line.
Best Practice: Use descriptive names that reflect the product's purpose, such as WindowsButton
instead of Button1
.
Final Thoughts
When applied correctly, the Abstract Factory Pattern can significantly enhance the adaptability and modularity of a Java application. However, as we’ve discussed, careful consideration needs to be taken to avoid the pitfalls that come with it, such as overengineering, violating principles of OOP, and compromising clarity.
Always remember: a tool is only as good as the person wielding it. By recognizing both the strengths and the weaknesses of the Abstract Factory Pattern, you can master its usage and avoid common missteps.
Further Reading
- Design Patterns in Java
- The Factory Pattern Explained
By diving deeper into these resources, you can continue expanding your understanding and application of design patterns in software development. Mastering the Abstract Factory Pattern is just a step towards becoming an adept designer and architect of robust systems. Happy coding!