Troubleshooting Data Sync Issues in Lambda Architecture
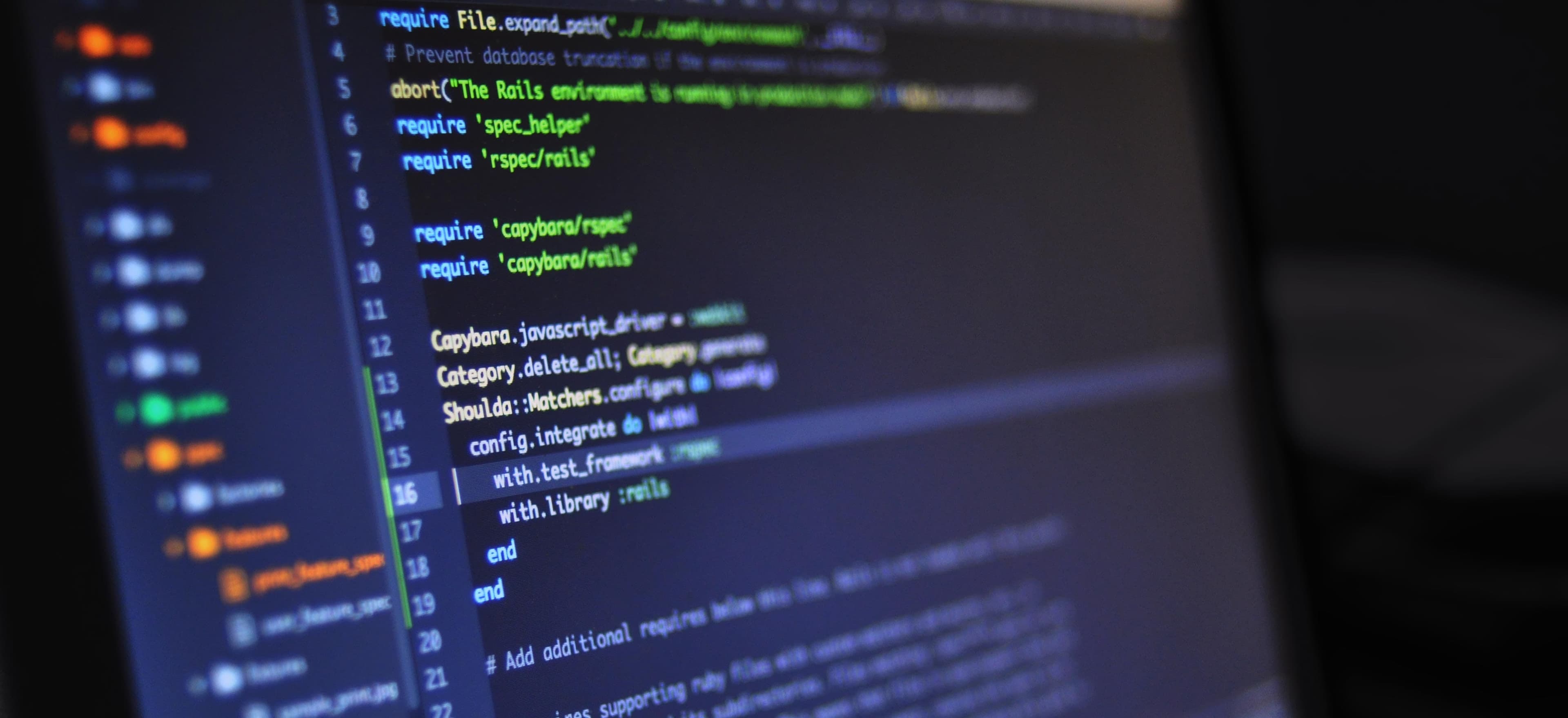
- Published on
Troubleshooting Data Sync Issues in Lambda Architecture
In modern data-intensive applications, Lambda Architecture has emerged as a robust design pattern that allows for real-time data processing along with batch processing, ensuring high throughput and low latency. However, like any system, it is not exempt from issues. One of the most prominent challenges is data sync issues. In this blog post, we will delve into these challenges, troubleshooting techniques, and the underlying principles that govern effective data synchronization.
Understanding Lambda Architecture
Before diving into data sync problems, it's essential to have a grasp of what Lambda Architecture entails. Lambda Architecture is typically comprised of three layers:
- Batch Layer: This layer manages the master data set and pre-computes the batch views.
- Speed Layer: This component deals with real-time data processing and ensures that minimal latency is achieved.
- Serving Layer: This layer indexes the data from both the batch and speed layers to serve queries.
Why Data Sync Issues Occur
Data syncing issues can arise for several reasons, including:
- Latency between Batch and Speed Layer: Discrepancies in the timing of data ingestion can lead to mismatched views.
- Faulty Mapping Strategies: Inconsistent data mapping can obscure how data is interpreted across layers.
- Data Quality: Garbage data or schema mismatches can cause sync disruptions.
- System Configuration: Improper configurations in data pipelines can exacerbate sync issues.
Understanding these causes can illuminate troubleshooting approaches.
Identifying Data Sync Problems
Symptoms of Data Sync Issues
Identifying a data sync issue often begins with recognizing the symptoms:
- Data does not match between batch and speed views.
- Real-time metrics deviate significantly after batch jobs run.
- Increased latency observed during data queries.
If you notice any of these symptoms, it’s time to drill down and identify the root causes.
Comprehensive Troubleshooting Steps
1. Validate Data Ingestion Processes
Data ingestion is the first point where synchronization can falter. Examine the following:
- Ensure data is being ingested in the correct format.
- Confirm the data pipeline is successfully capturing all events.
- Utilize monitoring tools to track data commands in real-time.
Example Code Snippet for Data Ingestion Validation
Here's a simple Java example using Apache Kafka to validate your data ingestion process:
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import org.apache.kafka.clients.consumer.ConsumerRecord;
import java.time.Duration;
import java.util.Collections;
import java.util.Properties;
public class DataIngestionValidator {
public static void main(String[] args) {
Properties props = new Properties();
props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(ConsumerConfig.GROUP_ID_CONFIG, "data-sync-validator");
props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringDeserializer");
props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringDeserializer");
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Collections.singleton("data-topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("Received message: key = %s, value = %s%n", record.key(), record.value());
// Validation logic here
}
}
}
}
Why This Matters: By consuming messages from your data pipeline in real-time, you can confirm that data is ingested correctly, which is the first step in ensuring synchronization.
2. Scrutinize Data Processing Logic
Once you've validated that data is ingested properly, assess the logic that processes this data for both batch and speed layers:
- Check if both layers use the same transformation logic.
- Ensure consistent schema definitions across layers.
3. Implement a Monitoring Solution
A robust monitoring solution can help pinpoint issues before they escalate. Here are a few key metrics to observe:
- Throughput: Measure the rate of data processing.
- Latency: Compare the time taken for batch and speed processing.
- Error Rates: Track the number of ingestion or transformation errors.
Consider utilizing tools such as Apache Kafka’s JMX metrics or third-party solutions like Prometheus for deeper insights.
4. Use Data Comparison Techniques
This step involves directly comparing the outputs of the batch and speed layers:
- Develop scripts to compare datasets produced by both layers.
- Utilize checksum or hashing algorithms to verify that data is consistent.
Example Code Snippet for Data Comparison
Below is an example of a simple Java application that performs data comparison:
import java.util.HashMap;
import java.util.Map;
public class DataComparer {
public static void main(String[] args) {
// Dummy data simulating batch layer records
Map<String, Integer> batchData = new HashMap<>();
batchData.put("record1", 100);
batchData.put("record2", 200);
// Simulating speed layer records
Map<String, Integer> speedData = new HashMap<>();
speedData.put("record1", 100);
speedData.put("record2", 250); // Intentional difference
compareData(batchData, speedData);
}
private static void compareData(Map<String, Integer> batchData, Map<String, Integer> speedData) {
for(String key : batchData.keySet()) {
if (!batchData.get(key).equals(speedData.get(key))) {
System.out.printf("Data mismatch for key: %s, Batch: %d, Speed: %d%n", key, batchData.get(key), speedData.get(key));
}
}
}
}
Why This Matters: Comparing datasets helps reveal discrepancies, providing insight into whether your data synchronization is functioning correctly.
5. Review System Configurations and Architecture Design
Check your architecture for potential configuration errors:
- Ensure that both batch and speed layers are correctly wired to the same data sources.
- Verify that the services are scaled to handle peak loads efficiently.
Closing the Chapter
Troubleshooting data sync issues in Lambda Architecture requires a structured approach to examine points of failure. By validating data ingestion processes, scrutinizing processing logic, implementing effective monitoring, and utilizing comparison techniques, you can enhance your system’s reliability.
For more in-depth knowledge about Lambda Architecture, take a look at this comprehensive guide on Lambda Architecture.
Remember, ensuring data synchronization is not an end goal; it's a continuous process of monitoring, validating, and refining your architecture. By diligently following these steps, you can mitigate data sync issues and bolster the integrity of your data-driven applications.
For more discussions on data architecture and engineering principles, check out Towards Data Science for valuable insights and community-driven content. Happy coding!