TreeMap vs HashMap: Choosing the Right Java Collection
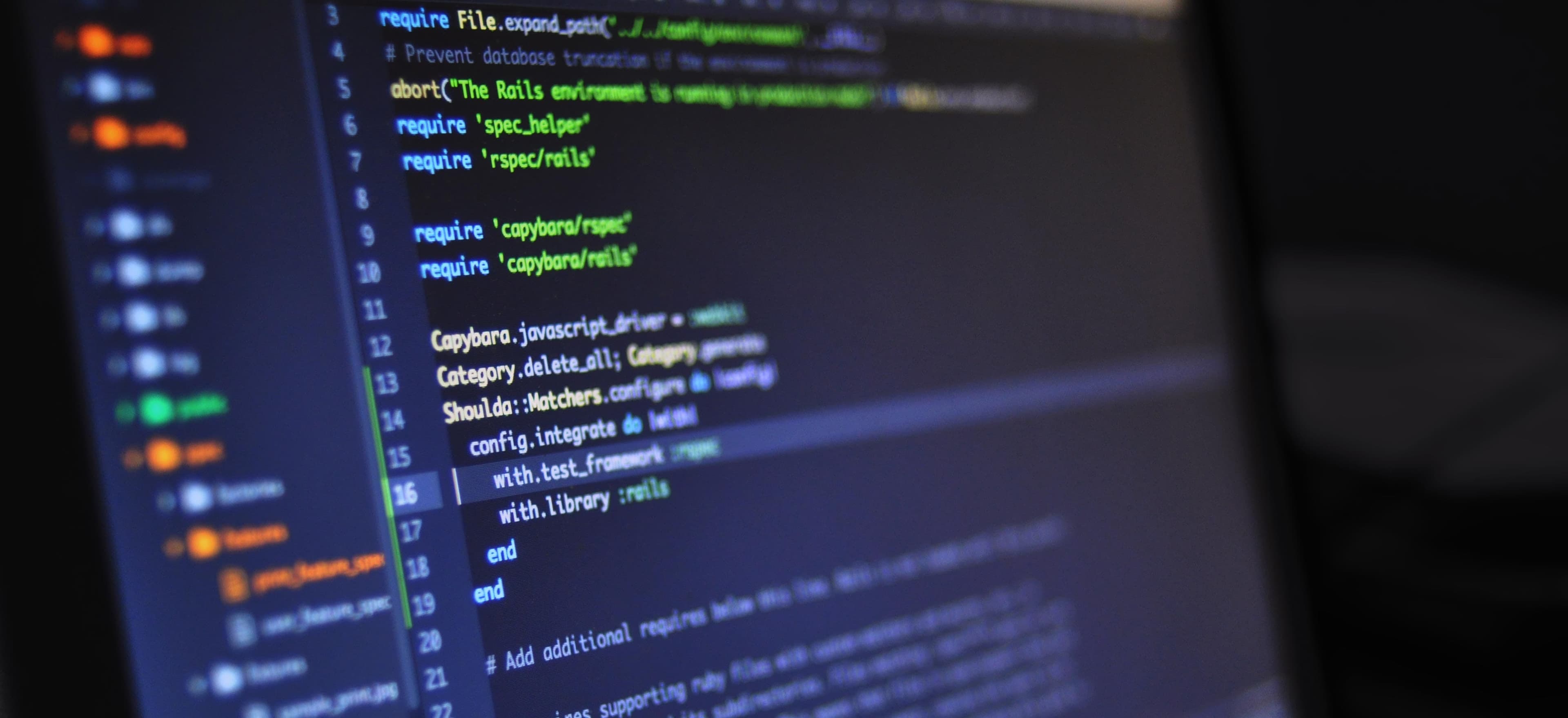
- Published on
TreeMap vs HashMap: Choosing the Right Java Collection
When working with Java collections, deciding between TreeMap
and HashMap
can significantly affect the performance and behavior of your application. Both classes implement the Map
interface, but they have different characteristics that make them suitable for different use cases. This blog post will delve into the key differences between TreeMap
and HashMap
, and guide you in choosing the right collection for your needs. Let's look at this through the framework of performance, order, and use cases.
Overview of HashMap
HashMap
is part of the java.util
package and is a commonly used collection for storing key-value pairs. It leverages a hash table for implementation.
Key Features of HashMap:
- Performance: Offers O(1) average time complexity for basic operations like adding, removing, and accessing elements.
- Ordering: The order of keys is unpredictable. This means elements can seem random when iterated over.
- Null Keys and Values: Allows one null key and multiple null values.
Basic Example of HashMap
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
// Adding key-value pairs
map.put("Apple", 1);
map.put("Banana", 2);
map.put("Mango", 3);
// Retrieving a value
System.out.println("Value for 'Apple': " + map.get("Apple")); // Outputs: 1
// Iterating through the map
for (String key : map.keySet()) {
System.out.println(key + ": " + map.get(key));
}
}
}
Why Use HashMap?
Choosing HashMap
is particularly beneficial when you prioritize speed and your key ordering does not matter. This makes it ideal for applications that require fast lookups and updates.
Overview of TreeMap
TreeMap
, also part of the java.util
package, implements the Map
interface and uses a Red-Black Tree structure to store elements.
Key Features of TreeMap:
- Performance: Offers O(log n) time complexity for basic operations, including adding, removing, and accessing elements.
- Ordering: Maintains a sorted order of keys based on their natural ordering or a specified comparator.
- Null Keys and Values: Does not allow null keys, but multiple null values are permitted.
Basic Example of TreeMap
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String[] args) {
TreeMap<String, Integer> treeMap = new TreeMap<>();
// Adding key-value pairs
treeMap.put("Apple", 1);
treeMap.put("Banana", 2);
treeMap.put("Mango", 3);
// Retrieving a value
System.out.println("Value for 'Apple': " + treeMap.get("Apple")); // Outputs: 1
// Iterating through the map in sorted order
for (String key : treeMap.keySet()) {
System.out.println(key + ": " + treeMap.get(key));
}
}
}
Why Use TreeMap?
Opt for TreeMap
when you require a sorted map or need to perform range queries or number of total elements in a specific range.
Key Differences between HashMap and TreeMap
| Feature | HashMap | TreeMap | |---------------|-------------------------------|-------------------------------| | Performance | O(1) average time complexity | O(log n) time complexity | | Order | No ordering | Sorted order based on keys | | Null Keys | Allows one null key | No null keys allowed | | Null Values | Allows multiple null values | Allows multiple null values |
When to Use Each
-
Use HashMap when:
- You need fast access, insertion, and deletion.
- The order of elements doesn't matter.
- You are okay with a potential overhead in terms of memory due to hashing.
-
Use TreeMap when:
- You need sorted keys.
- You are performing range operations or need to maintain a natural order.
- You can trade off performance for sorted guarantees.
Performance Considerations
When multitasking with large data sets, performance can dramatically impact the user experience. Understanding how hash codes work in HashMap
can help diagnose bottlenecks. A poor hash function can lead to performance issues due to excessive collisions.
Although both collections provide respective qualities, the performance of HashMap
tends to be superior for simple lookups. It’s crucial to consider the collection size. For smaller sizes, the cost difference may not be significant.
The Closing Argument
Choosing between HashMap
and TreeMap
boils down to your application’s needs. If speed is your priority and order is irrelevant, go for HashMap
. On the other hand, if you need a sorted representation of data, TreeMap
is the way to go.
For in-depth reading and advanced topics regarding Java Collections Framework, you can check out Oracle's Java Documentation and Java Tutorials on Collections.
In the evolving landscape of Java, being informed can guide you to make judicious decisions that will enhance code efficiency and readability.
Checkout our other articles