Mastering Microservices Testing: Common Pitfalls to Avoid
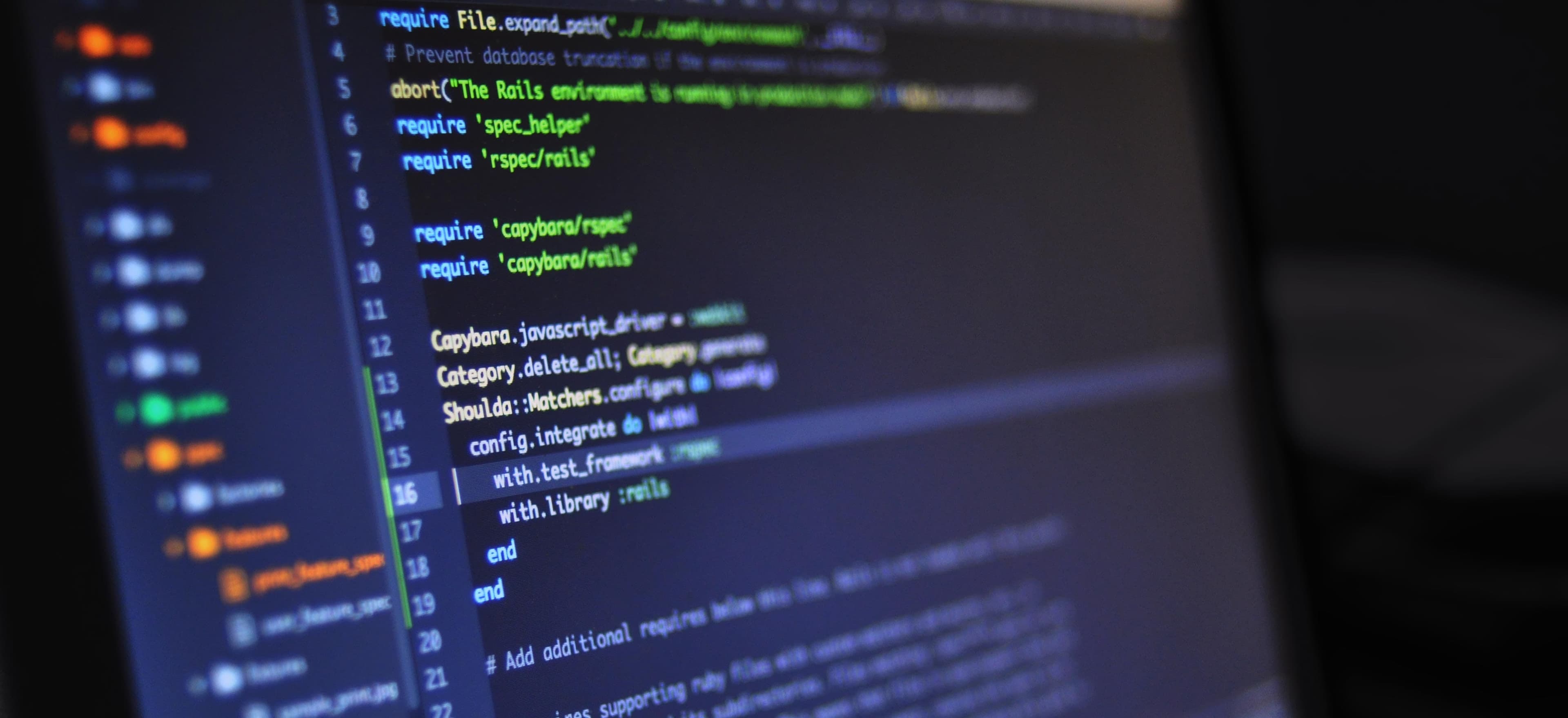
- Published on
Mastering Microservices Testing: Common Pitfalls to Avoid
In the realm of modern software development, microservices have emerged as a favored architectural pattern. But as organizations embrace microservices, they encounter unique challenges, especially in testing. Reliable testing is critical to ensure that each microservice operates independently and interacts seamlessly with others. In this post, we will explore common pitfalls in microservices testing and how to master them effectively.
Understanding Microservices
Before diving into testing, it’s crucial to grasp what microservices entail. Microservices architecture breaks down an application into smaller, self-contained services that can communicate over networks. Each service handles a specific task, having its own codebase and database, enabling teams to work autonomously.
However, this complexity introduces specific testing challenges that traditional testing methods may not address.
Common Pitfalls in Microservices Testing
1. Lack of a Testing Strategy
One major pitfall is entering the testing phase without a well-defined strategy. Ad-hoc testing often leads to an inconsistent quality assurance process.
Why It Matters: A documented testing strategy provides clarity on what to test, how to test, and when to test. This ensures all teams are aligned and reduces redundancy.
Solution: Develop a comprehensive testing strategy outlining the types of tests needed such as unit tests, integration tests, and end-to-end tests. Additionally, employ the test pyramid concept to prioritize different testing types.
2. Ignoring Service Dependencies
Microservices rarely operate in isolation. Every service often has dependencies on others. Ignoring these relationships may cause testing to miss identifying critical issues.
Why It Matters: When dependencies are not considered, you might get false positives during testing, leading to unreliable systems in production.
Solution:
Implement contract testing using tools like Pact. Contract testing verifies that each service adheres to its agreed-upon API contracts. This way, changes in one service don't break others unexpectedly.
Here’s a basic example of how to set up a consumer-driven contract in Pact:
// Consumer side contract
public class ConsumerTest {
PactProviderRule pactProviderRule = new PactProviderRule("Provider",
"localhost",
8080,
this);
@Test
@Pact(consumer = "Consumer", provider = "Provider")
public void runTest(PactDslWithProvider builder) {
builder
.given("data exists")
.uponReceiving("a request for data")
.path("/data")
.method("GET")
.willRespondWith()
.status(200)
.body("{ \"message\": \"Hello World\" }");
}
}
3. Underestimating Unit Testing
Perhaps, the most crucial aspect of building reliable microservices is robust unit testing. Some teams fail to prioritize unit testing, assuming integration and end-to-end tests are sufficient.
Why It Matters: Unit tests isolate the smallest components, ensuring each piece behaves correctly. Skipping this step can lead to undetected bugs cascading through your deployment pipeline.
Solution:
Adopt a Test-Driven Development (TDD) approach, where writing tests precedes writing functional code. Consider the following simplistic unit test with JUnit:
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void addPositiveNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 3);
assertEquals(8, result); // Test case
}
}
4. Not Using Automated Testing Tools
In a world of continuous integration and deployment, manual testing becomes impractical. Many teams still rely on manual testing which can result in inconsistent outcomes.
Why It Matters: Automated testing allows for consistent execution frequency and can assist in catching bugs earlier in the development cycle.
Solution: Utilize automation frameworks such as Selenium for web services, JUnit for Java applications, or Postman for API testing.
Employ CI/CD (Continuous Integration/Continuous Deployment) tools like Jenkins or GitHub Actions to automatically run your tests on each code commit.
5. Failing to Test in an Integrated Environment
Testing microservices in isolation often leads to flaws not showing up until it reaches production. This issue arises when integration testing environments do not mirror the production settings.
Why It Matters: The production environment typically reflects comprehensive data flows, making it critical to replicate as closely as possible during testing.
Solution:
Use Docker containers to create isolated testing environments closely resembling production. Deploy each service in its container, allowing accurate simulations of real-world interactions.
Here is a simplified example of a Dockerfile:
# Base image
FROM openjdk:11
# Setting working directory
WORKDIR /app
# Copy and build app
COPY target/myapp.jar myapp.jar
ENTRYPOINT ["java", "-jar", "myapp.jar"]
6. Overlooking Performance Testing
Microservices allow for quick development, but the performance impact of multiple services is often overlooked.
Why It Matters: As services scale, it’s essential to ensure that each performs under the expected load.
Solution: Implement performance testing using tools like JMeter or Gatling. It can help simulate various loads and gauge how the microservices perform.
When creating performance tests, consider the following code snippet:
import org.apache.jmeter.junit.JMeter;
public class MyServiceLoadTest {
@Test
public void performanceTest() {
// Simulate load
JMeter.performLoadTest(myServiceEndpoint, 1000, 60);
// Assertions
JMeter.assertThroughputAboveThreshold(100);
}
}
Final Thoughts
Mastering microservices testing requires avoiding the pitfalls that can diminish software quality. By implementing strategies like comprehensive testing plans, contract testing, automated tools, containerization, and performance tests, organizations can build robust microservice applications.
Always remember, testing is not a final step in the development process but an integral part of it. Make testing an essential practice throughout the software development lifecycle to ensure the reliability and performance of microservices.
For further reading on software testing best practices, check out Martin Fowler’s resources or dive deeper into microservices at Microservices.io. The more knowledge and tools you leverage, the more effective your testing strategy will be.
With persistence and continuous learning, mastering microservices testing is within your reach. Happy testing!