Avoid These Common Mistakes in Spring MVC Development
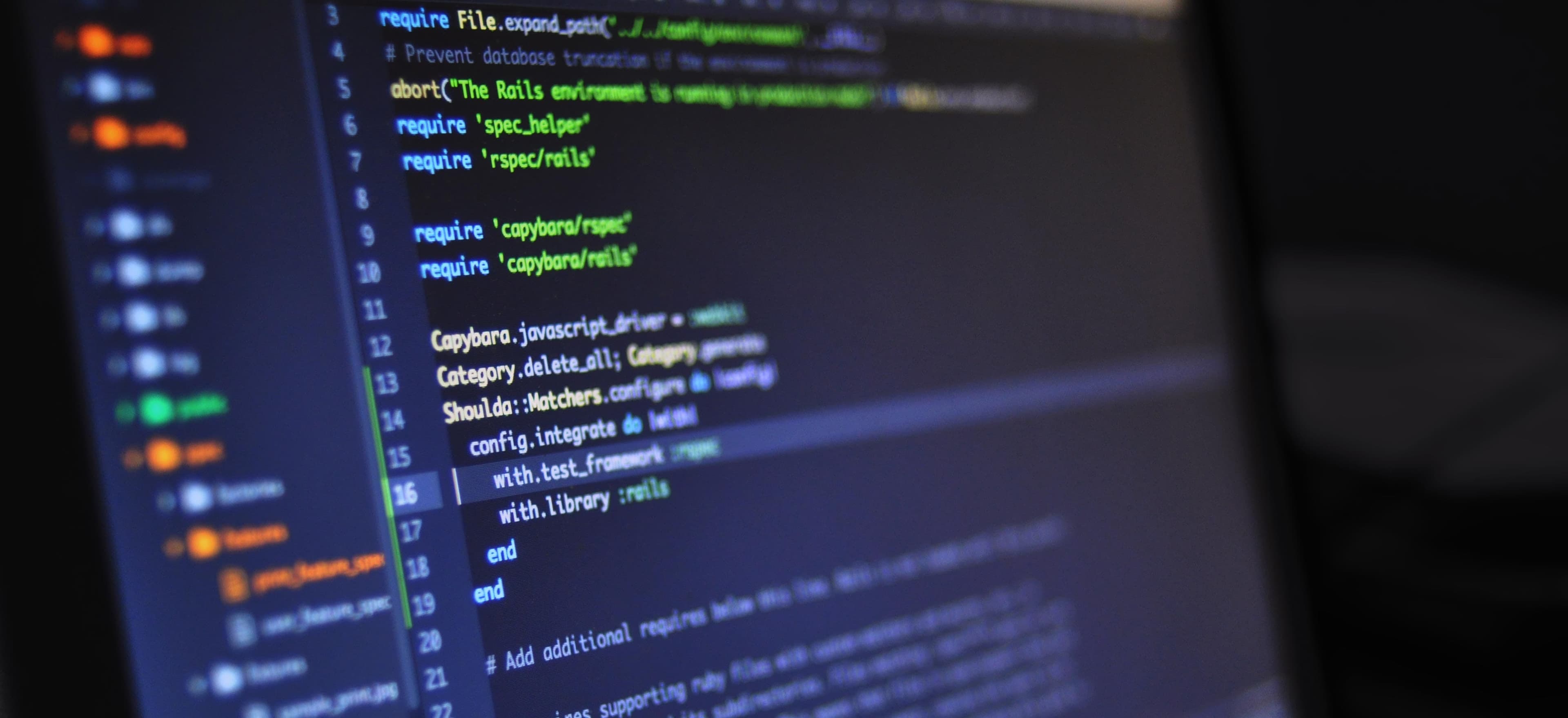
- Published on
Avoid These Common Mistakes in Spring MVC Development
Spring MVC is one of the most popular frameworks for building robust and scalable web applications in Java. Its powerful features, flexibility, and support for RESTful web services make it an essential tool for developers. However, despite its many advantages, developers can often make critical mistakes that can hinder application performance and security. In this guide, we'll outline some common pitfalls in Spring MVC development and how to avoid them.
1. Ignoring Exception Handling
Why It Matters
One of the most overlooked aspects of web application development is how to handle exceptions properly. In Spring MVC, failing to manage exceptions can lead to uninformative error messages or, worse, a system crash.
Solutions
Leverage Spring's built-in exception handling capabilities by using the @ControllerAdvice
annotation. This allows you to handle exceptions across your entire application in a centralized manner.
Example
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.servlet.ModelAndView;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public ModelAndView handleResourceNotFound(ResourceNotFoundException ex) {
ModelAndView mav = new ModelAndView("error");
mav.addObject("errorMessage", ex.getMessage());
return mav;
}
// Other exception handlers
}
In this example, when a ResourceNotFoundException
is thrown, a specific error page will be shown to the user. This results in a much better user experience, rather than exposing them to stack traces or predefined error messages.
2. Not Utilizing Spring's Built-In Features
Why It Matters
Spring MVC comes with a plethora of built-in features that can save you time and make your application more efficient. Failing to utilize these features often leads to redundant or inefficient code.
Solutions
Make use of features such as @RequestMapping
, data binding, validation, and model attributes effectively. Additionally, Spring's RestTemplate can be used for making HTTP requests rather than writing custom logic.
Example
Simply using the @RequestMapping
annotation allows you to map URLs effortlessly to your controller methods:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class MyController {
@GetMapping("/greet")
@ResponseBody
public String greet(@RequestParam String name) {
return "Hello, " + name + "!";
}
}
In this illustration, a simple endpoint is created to greet users without much overhead. This is not only cleaner but also ensures maintainability.
3. Violating the Single Responsibility Principle
Why It Matters
Having controllers that handle multiple concerns can complicate testing, maintenance, and readability. Each controller should focus on a single business function.
Solutions
Keep each controller dedicated to a particular aspect of your application, and use services to handle business logic.
Example
Consider separating your controller and service logic:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.save(user);
}
}
By isolating the controller from business logic, the code becomes cleaner and adheres to the Single Responsibility Principle.
4. Hardcoding Configuration Values
Why It Matters
Hardcoding configuration values makes your system less flexible and maintainable. If you need to change a value, you often have to go through multiple files to make the necessary updates.
Solutions
Use Spring's external property files. This method allows for easy change management without requiring code modifications.
Example
Place your properties in an application.properties file:
# application.properties
server.port=8080
app.name=My Application
Then, you can easily access them in your Java code using @Value
.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class ApplicationConfig {
@Value("${app.name}")
private String appName;
public String getAppName() {
return appName;
}
}
This approach allows for easier deployment in different environments without requiring code changes.
5. Overlooking Security
Why It Matters
Spring MVC applications are susceptible to various security vulnerabilities, including cross-site scripting (XSS) and cross-site request forgery (CSRF). Ignoring security best practices can lead to serious exploits.
Solutions
Always validate user inputs, use Spring Security for securing endpoints, and enable CSRF protection.
Example
Enable CSRF protection in your configuration:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.csrf().and()
.formLogin();
}
}
This code configures basic security settings and should always be a component of your application design. For more on security, see Spring Security Documentation.
The Last Word
Developing applications with Spring MVC can be incredibly rewarding, but avoiding common pitfalls is essential. By focusing on exception handling, leveraging built-in features, adhering to design principles, externalizing configuration, and prioritizing security, you can build more robust, efficient, and maintainable applications.
Remember, the key to successful Spring MVC development lies in understanding and practicing the right principles and making the best use of the tools at your disposal. Always keep learning and refactoring your code to adapt to new challenges, and you'll find that Spring MVC can take your Java applications to superb heights.
For further mastery, consider exploring the official Spring Guides that provide hands-on examples and best practices for various use cases.
Happy coding!
Checkout our other articles