Top 5 Core Java Interview Questions That Stump Candidates
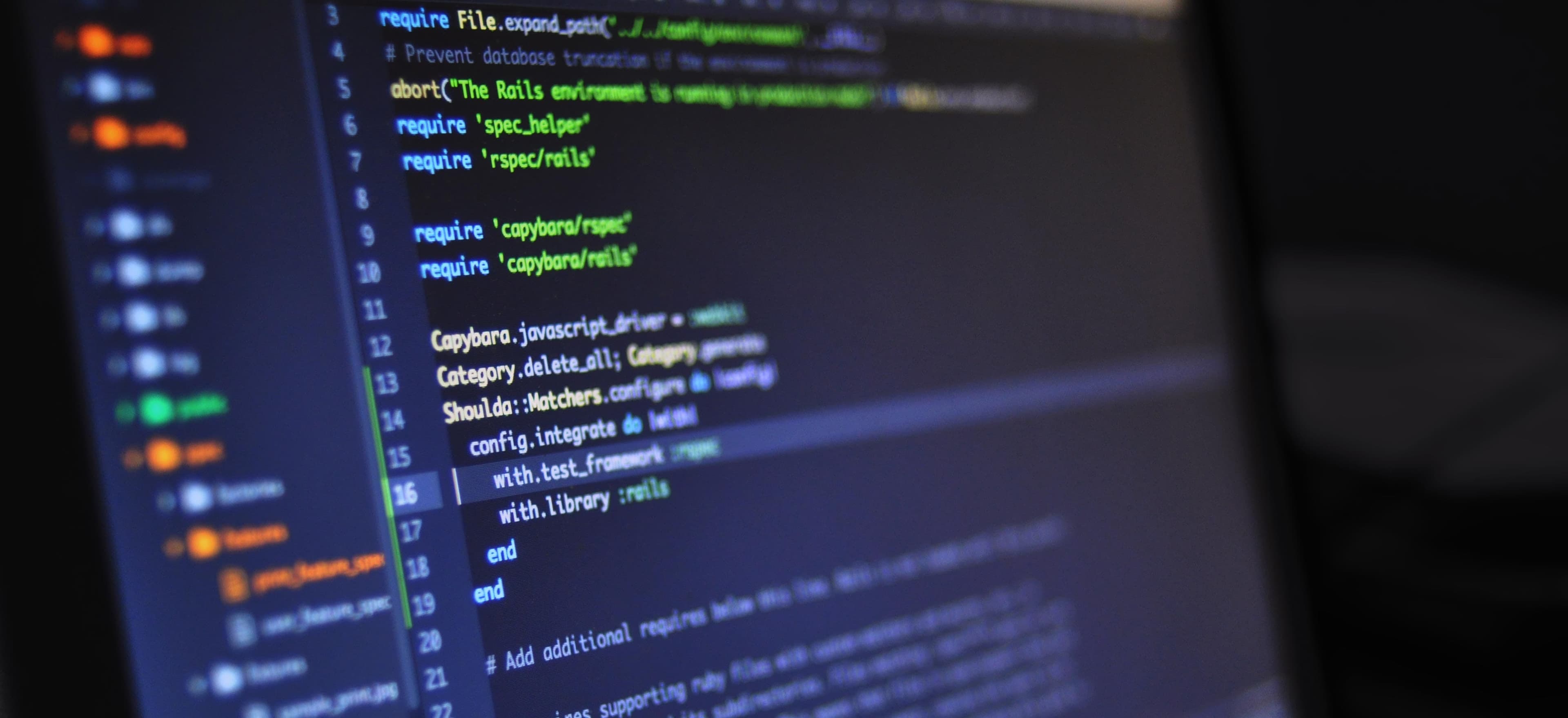
- Published on
Top 5 Core Java Interview Questions That Stump Candidates
Java continues to be one of the most widely used programming languages in the world. As developers pursue jobs that require proficiency in this language, preparing for interviews becomes crucial. However, many candidates find themselves stumped by certain core Java interview questions. In this blog post, we'll delve into the top five questions often encountered during interviews, providing you not only with the answers but also the reasoning behind them. This comprehensive approach will help you gain a deeper understanding of Java and enhance your preparation strategy.
1. Explain the concept of Java Virtual Machine (JVM)
Why It's Important
Understanding the JVM is foundational for any Java developer. It plays a crucial role in executing Java applications and managing system resources.
Answer
The Java Virtual Machine (JVM) is an abstract machine that enables Java bytecode to run on any system. Its primary role is to translate bytecode into machine code, allowing Java applications to be platform-independent—this characteristic is encapsulated in the phrase "Write Once, Run Anywhere" (WORA).
Core Responsibilities of JVM:
- Loading code: The JVM loads class files.
- Verifying code: It checks the code for security and integrity before execution.
- Executing code: The execution of the bytecode takes place here.
- Memory management: The JVM includes a garbage collector that helps manage memory.
Example Code Snippet: A simple Java program to illustrate the usage of the JVM.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This program generates bytecode that the JVM executes.
Lessons Learned
Familiarizing yourself with JVM concepts not only prepares you for interviews, but it also enhances your programming efficiency by making you aware of how Java operates under the hood.
2. What is the difference between ==
and equals()
in Java?
Why It's Important
This question is a common pitfall, as many candidates confuse the two concepts. Understanding the distinction is critical for comparing objects meaningfully.
Answer
In Java, ==
and equals()
serve different purposes:
-
==
Operator: It checks for reference equality, meaning it verifies if two references point to the same object in memory. -
equals()
Method: It is used for content equality. By default, it also checks for reference equality unless overridden.
Example Code Snippet:
String str1 = new String("Hello");
String str2 = new String("Hello");
// Using == to compare references
if (str1 == str2) {
System.out.println("str1 and str2 are the same reference.");
} else {
System.out.println("str1 and str2 are different references.");
}
// Using equals() to compare content
if (str1.equals(str2)) {
System.out.println("str1 and str2 have the same content.");
} else {
System.out.println("str1 and str2 have different content.");
}
Lessons Learned
The difference between ==
and equals()
is pivotal for ensuring that Java developers can accurately compare object instances. Knowing when to use each is vital for avoiding subtle bugs.
3. What are the main principles of Object-Oriented Programming (OOP) in Java?
Why It's Important
With Java being inherently object-oriented, understanding OOP principles is essential for effective software design.
Answer
The four main principles of OOP in Java are:
-
Encapsulation: This principle restricts direct access to certain components of an object. It enhances security, making it difficult to misuse class properties.
Example:
public class Person { private String name; // private field // Public setter public void setName(String name) { this.name = name; } // Public getter public String getName() { return this.name; } }
-
Inheritance: This allows one class to inherit properties and methods from another, promoting code reuse.
Example:
public class Animal { public void eat() { System.out.println("Eating..."); } } public class Dog extends Animal { public void bark() { System.out.println("Barking..."); } }
-
Polymorphism: This allows methods to do different things based on the object that it is acting upon, often through method overriding.
Example:
public class Animal { void sound() { System.out.println("Animal makes a sound"); } } public class Cat extends Animal { void sound() { System.out.println("Cat meows"); } }
-
Abstraction: This conceals complex implementation details and exposes only the necessary features. Abstract classes and interfaces help achieve this.
Example:
abstract class Shape { abstract void draw(); // Abstract method } class Circle extends Shape { void draw() { System.out.println("Drawing Circle"); } }
Lessons Learned
Grasping the principles of OOP is essential not only to solve interview questions but also to design scalable and maintainable software.
4. What is a Java interface and how is it different from an abstract class?
Why It's Important
Interfaces and abstract classes are essential building blocks of Java programming. Understanding the distinction can enhance your object-oriented design skills.
Answer
An interface in Java defines a contract that implementing classes must follow. It can contain abstract methods (without a body) and default methods. All methods in an interface are implicitly public.
Key Differences Between Interface and Abstract Class:
-
Abstract Class:
- Can contain both abstract and concrete methods (with implementation).
- It can have state (fields and constructors).
- A class can extend only one abstract class (single inheritance).
-
Interface:
- Can only contain abstract methods until Java 8, after which default and static methods can also be included.
- Cannot have constructors or any field other than static final fields.
- A class can implement multiple interfaces (multiple inheritance).
Example Code Snippet:
interface Animal {
void makeSound(); // Abstract method
}
abstract class Mammal {
abstract void displayInfo(); // Abstract method
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Mammal implements Animal {
void makeSound() {
System.out.println("Dog barks!");
}
void displayInfo() {
System.out.println("This is a mammal.");
}
}
Lessons Learned
Understanding the differences between interfaces and abstract classes is pivotal for effective design patterns in Java.
5. What is exception handling, and how does it work in Java?
Why It's Important
Exception handling is a core part of Java programming that ensures robustness and error management in applications.
Answer
Java uses a robust exception-handling mechanism that helps handle runtime errors, allowing the program to continue executing.
Key Components of Exception Handling:
- Try Block: Code that might throw an exception is placed in a try block.
- Catch Block: This block catches and handles the exception.
- Finally Block (Optional): This block executes regardless of whether an exception was caught.
Example Code Snippet:
public class ExceptionDemo {
public static void main(String[] args) {
try {
int result = 10 / 0; // This will throw ArithmeticException
System.out.println(result);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero: " + e.getMessage());
} finally {
System.out.println("Executed regardless of exception.");
}
}
}
Lessons Learned
Mastering Java's exception handling helps build robust applications that can effectively manage unexpected scenarios.
Lessons Learned
Java interviews can be intimidating, especially when faced with complex core topics. However, with the right preparation and understanding of fundamental concepts, candidates can significantly improve their chances of success.
The five interview questions we discussed are designed not only to challenge candidates but also to explore their understanding of Java's foundations. Knowing how to explain concepts like JVM, OOP principles, and exception handling articulately will greatly enhance your confidence in interviews.
For more guidance on mastering Java and preparing for interviews, consider checking resources like Java Documentation and websites such as GeeksforGeeks for extensive tutorials and sample questions. Happy coding and good luck with your interviews!
Checkout our other articles