Resolving Consistency Issues in Split-Brain Scenarios
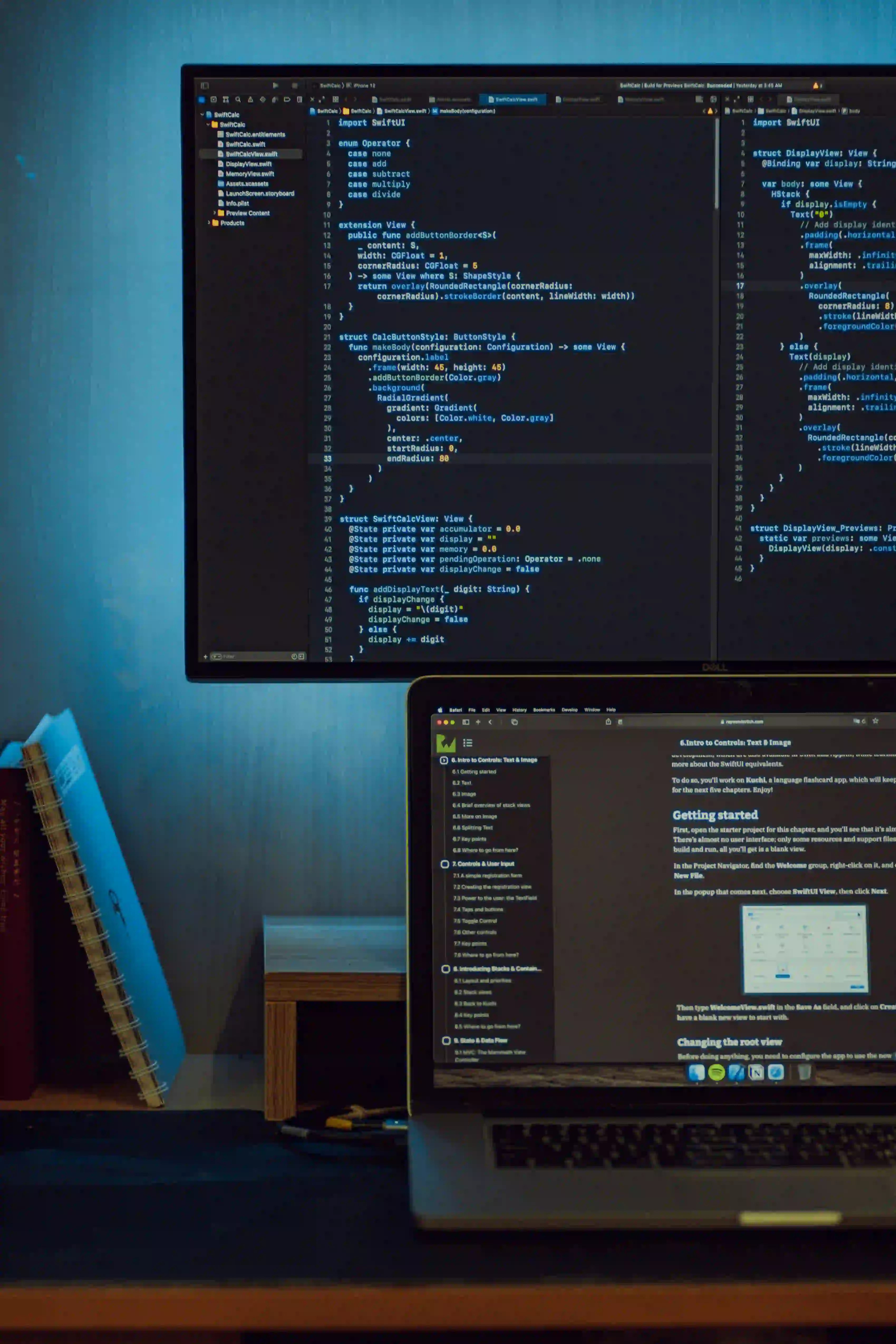
Resolving Consistency Issues in Split-Brain Scenarios
In the world of distributed systems, the term "split-brain" refers to a situation where a network partitioning occurs, causing different parts of a system to operate independently. This can lead to consistency issues, as different nodes may perceive differing states—and in extreme cases, different values for the same data. Addressing these issues is essential to maintaining reliability and ensuring data integrity across your application.
In this blog post, we will explore key strategies for resolving consistency issues in split-brain scenarios, focusing specifically on Java-based solutions. We’ll delve into important concepts, provide illustrative code snippets, and offer practical insights.
Understanding Split-Brain Scenarios
What Causes Split-Brain?
Split-brain typically occurs in distributed systems due to network failures, hardware malfunctions, or unforeseen events that disrupt communication between nodes. When this happens, each partition of the network can continue to operate in isolation, leading to conflicting data states.
Consequences of Split-Brain
The main consequences of split-brain scenarios include:
- Data Inconsistency: Different nodes may record different states for the same piece of data.
- Compromised Availability: Portions of your system may become unreachable, affecting user experience and system functionality.
- Complicated Recovery: Merging the different states after resolving the partition can be challenging.
Strategies for Mitigating Consistency Issues
To resolve consistency issues, numerous strategies can be applied. We will focus on the following three main approaches: Quorum-based systems, Leader Election, and Conflict-free Replicated Data Types (CRDTs).
1. Quorum-Based Systems
In a quorum-based system, you can achieve high availability while ensuring consistency by requiring a majority of nodes to agree on a given value before it is accepted. For example, in a group of five nodes, you’d need at least three to form a consensus.
Here's an illustrative Java code snippet demonstrating a simple quorum system:
import java.util.ArrayList;
import java.util.List;
public class QuorumSystem {
private List<String> nodes;
private static final int QUORUM_SIZE;
static {
QUORUM_SIZE = 3; // For simplicity, we need a majority.
}
public QuorumSystem() {
nodes = new ArrayList<>();
// Initialize the nodes (this is usually set up through networking)
}
public boolean writeData(String data) {
int votes = 0;
for (String node : nodes) {
if (sendWriteRequest(node, data)) {
votes++;
}
if (votes >= QUORUM_SIZE) {
return true; // Consensus reached
}
}
return false; // Failed to reach quorum
}
private boolean sendWriteRequest(String node, String data) {
// Simulate sending request to node and returning success or failure
return true; // Assume success for demonstration purposes
}
}
Why Quorum?
The reason for using a quorum system is rooted in the consensus algorithm itself. By deriving majority agreement from multiple nodes, the system effectively mitigates the risk of errors due to individual node failures and ensures only consistent data is written.
For a deeper understanding of quorum systems, check out CAP Theorem, which discusses the trade-offs between Consistency, Availability, and Partition Tolerance.
2. Leader Election
Another effective approach to managing split-brain situations is by implementing a leader election algorithm. In this model, one node is elected as the leader responsible for directing operations, while other nodes remain followers. If the leader fails or a network partition occurs, a new leader can be elected.
Below is a simplified implementation using an arbitrary leader election algorithm:
import java.util.Random;
public class LeaderElection {
private boolean isLeader;
private static final Random rand = new Random();
public LeaderElection() {
this.isLeader = electLeader();
}
private boolean electLeader() {
// Simulating leader election based on random votes
return rand.nextBoolean();
}
public void performTask() {
if (!isLeader) {
System.out.println("Forwarding to Leader...");
// Logic to forward task to the leader
} else {
System.out.println("Performing task as Leader...");
// Logic for the leader to perform the task
}
}
}
Why Leader Election?
Leader election is critical in maintaining a single source of truth. By funneling requests through the leader, conflicts are avoided, and data consistency is achieved. Although there may be a delay during elections, they play a pivotal role in ensuring a cohesive operation amidst disruptions.
You can refer to Apache ZooKeeper for real-world leader election implementations used in various distributed systems.
3. Conflict-free Replicated Data Types (CRDTs)
CRDTs are a class of data structures designed to allow for distributed data to be updated independently and still merge changes without conflicts. They are particularly useful in scenarios where multiple nodes might perform actions simultaneously.
A Java implementation of a simple counter using CRDT could look something like this:
import java.util.concurrent.atomic.AtomicInteger;
public class CRDTCounter {
private AtomicInteger value;
public CRDTCounter() {
this.value = new AtomicInteger(0);
}
public void increment() {
value.incrementAndGet();
}
public void merge(CRDTCounter other) {
this.value.addAndGet(other.value.get());
}
public int getValue() {
return value.get();
}
}
Why CRDTs?
CRDTs excel in scenarios where nodes may independently update a dataset, yet still need to reconcile differences later. By ensuring all operations are commutative and associative, CRDTs allow systems to recover to the correct state even after partitions are resolved.
For an in-depth analysis of CRDTs, visit this resource.
The Closing Argument
In a distributed system, encountering split-brain situations is unavoidable. Understanding and implementing robust strategies is fundamental to maintaining data consistency and overall system reliability.
- Quorum-Based Systems provide a structured way to reach consensus, minimizing conflicts.
- Leader Election centralizes operations, ensuring that decisions are made cohesively.
- CRDTs allow for independent updates, reducing the chances of conflicts across distributed nodes.
By leveraging these strategies, developers can create resilient systems capable of gracefully handling split-brain scenarios, ultimately enhancing user experience and system integrity. It’s crucial to understand your system’s specific requirements and apply the appropriate method while always keeping scalability and reliability in mind.
As distributed systems continue to evolve, so too will the methods for managing them. Embrace these concepts and stay ahead of the curve. Happy coding!