Common Pitfalls When Building a Java Message System
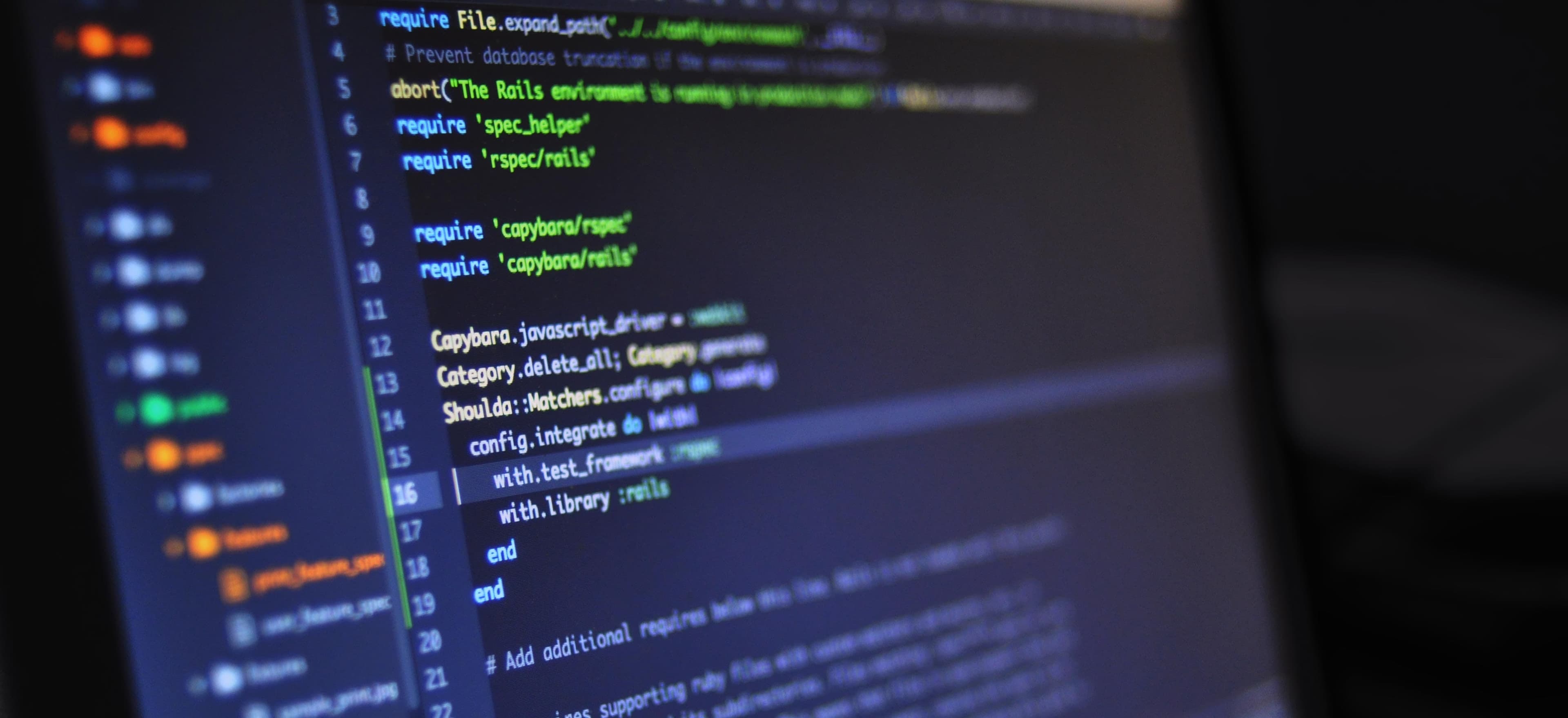
- Published on
Common Pitfalls When Building a Java Message System
Java is a powerful language that enables developers to build robust and scalable applications, including message systems. However, when constructing a message system, there are common pitfalls that developers often encounter. In this blog post, we will explore these pitfalls, how to avoid them, and provide sample code snippets along the way.
Understanding the Basics of a Java Message System
A Java message system facilitates communication between software components. Commonly used in distributed systems, it allows for asynchronous message passing through various messaging protocols. Technologies like JMS (Java Message Service) and libraries such as Apache Kafka or RabbitMQ are typically employed to implement such systems.
Before we delve into the pitfalls, it's essential to grasp the foundational concepts that govern a Java message system.
Core Functionalities of a Message System
- Send/Receive Messages: Message systems allow applications or services to send and receive messages.
- Asynchronous Communication: Components can communicate without waiting for each other’s response.
- Decoupling: They separate the sender's and receiver's concerns, allowing scalability.
- Message Persistence: Ensures that messages are not lost even in case of system failures.
Common Pitfalls in Building a Java Message System
1. Configuring Message Broker Incorrectly
One of the first steps when setting up a message system is configuring the message broker. An improper configuration can have severe implications on performance and reliability.
Pitfall Explanation
For instance, not setting up the correct persistence mechanisms can lead to message loss, especially if the application crashes. Some brokers may require specific configurations to handle message durability and acknowledgment properly.
Solution
Refer to the documentation of the specific message broker you are using. Implementing a basic configuration using Apache Kafka might look like this:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("acks", "all"); // Ensures that the leader acknowledges receipt of the record
props.put("retries", 3); // Defines how many times a send should be retried if it fails
2. Ignoring Message Formats
When constructing a message system, the format of the messages often leads to misunderstandings among developers.
Pitfall Explanation
If the format of the message is not well-defined and agreed upon, it can lead to serialization and deserialization issues that result in data corruption or misunderstandings.
Solution
Utilizing a widely accepted format such as JSON or Protocol Buffers can mitigate these issues. Here’s an example of sending a JSON message using the Jackson library:
ObjectMapper objectMapper = new ObjectMapper();
String jsonMessage = objectMapper.writeValueAsString(new MyMessage("Hello, World!"));
producer.send(new ProducerRecord<>("my-topic", jsonMessage));
3. Overlooking Message Size Limitations
Messaging systems often have restrictions on the size of messages.
Pitfall Explanation
If you attempt to send very large messages, you may encounter performance issues, or your messages may simply be rejected by the broker due to size constraints.
Solution
Keep your messages lightweight. For example, if your data is too large, consider breaking it into smaller messages or leveraging references instead of sending large payloads.
4. Not Implementing Retry Logic
Message systems can experience temporary failures. What happens if a message cannot be immediately processed?
Pitfall Explanation
If you don’t build in a retry mechanism, you risk losing messages or leaving them in a failed state.
Solution
Implement exponential backoff or simple retry logic. Here’s an example illustrating a retry mechanism using basic logging:
int maxRetries = 5;
int attempt = 0;
while (attempt < maxRetries) {
try {
producer.send(new ProducerRecord<>("my-topic", jsonMessage)).get();
break; // Break if message sending is successful
} catch (Exception e) {
attempt++;
if (attempt == maxRetries) {
System.err.println("Failed to send message after " + maxRetries + " attempts");
} else {
Thread.sleep((long) Math.pow(2, attempt) * 100); // Exponential backoff
}
}
}
5. Not Handling Message Ordering Issues
For many applications, maintaining the order of messages is crucial.
Pitfall Explanation
If you’re not careful, you may find that your messages arrive out of order, which can disrupt business logic or user experience.
Solution
To preserve order, use partitioning in Kafka, where messages within the same partition will preserve their order.
6. Neglecting Monitoring and Logging
Monitoring and logging provide visibility into your system’s health and behavior.
Pitfall Explanation
Ignoring these aspects can make it challenging to diagnose issues or optimize performance down the line.
Solution
Integrate established monitoring tools to track message throughput, latencies, and error rates. Here's a small snippet to log message sending:
producer.send(new ProducerRecord<>("my-topic", jsonMessage), (metadata, exception) -> {
if (exception == null) {
System.out.printf("Sent message with key: %s to partition: %d with offset: %d%n", key, metadata.partition(), metadata.offset());
} else {
System.err.println("Error sending message: " + exception.getMessage());
}
});
7. Ignoring Security Considerations
Security is paramount in any application; your message system should be no different.
Pitfall Explanation
Messages can contain sensitive information. Failing to encrypt these messages puts data at risk of unauthorized access.
Solution
Use transport-level security, like TLS/SSL, to encrypt the data in transit. For maximum security, consider end-to-end encryption of messages.
Closing Remarks
Building a Java message system can lead to successful, scalable applications. However, understanding the common pitfalls and their remedies significantly enhances your chance of success. From setting up your message broker correctly to implementing security best practices, each step is critical to the overall design.
Further Reading
By being mindful of these pitfalls, you can build a more reliable, efficient, and robust messaging system in Java. Happy coding!
Checkout our other articles