Overcoming Service Mesh Complexity in Microservices
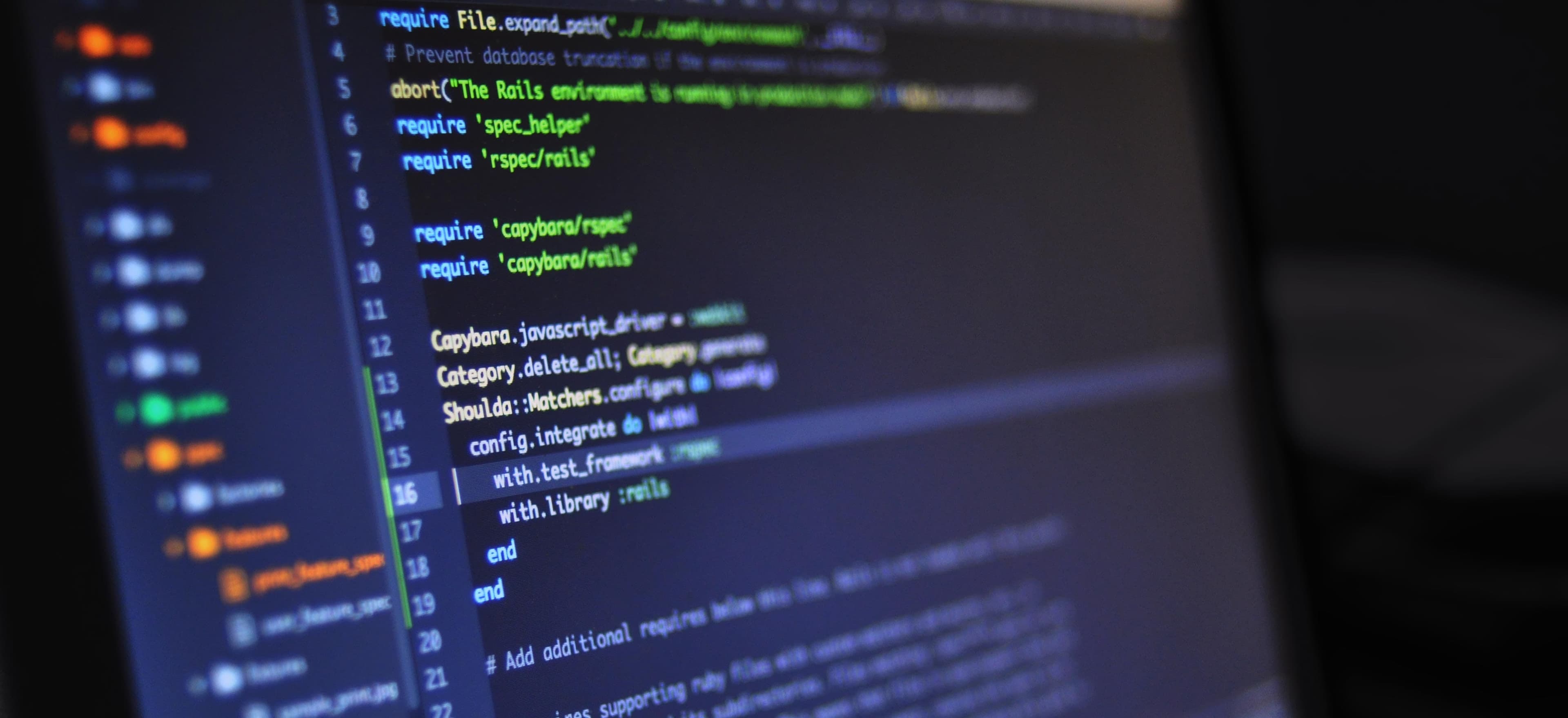
- Published on
Overcoming Service Mesh Complexity in Microservices
In modern software architecture, microservices provide a way to build complex applications as a suite of small, independently deployable services. However, as systems scale, managing service-to-service communications becomes increasingly complex. This is where a Service Mesh enters the scene.
A Service Mesh is an infrastructure layer that facilitates service-to-service communications, providing capabilities like traffic management, security, and observability. While these features can immensely simplify microservices architecture, they also introduce their own complexities.
In this blog post, we will explore how to overcome these challenges, making your Service Mesh implementation more efficient and straightforward.
What Is a Service Mesh?
Before diving into complexity, let’s clarify what a Service Mesh is.
A Service Mesh provides a set of functionalities to manage microservices interactions. Think of it as a dedicated infrastructure layer that helps you manage how different services communicate with each other. It achieves this by leveraging lightweight network proxies deployed alongside your services (often called a sidecar pattern).
Key Features of a Service Mesh
- Traffic Management: Control routing and load balancing between services.
- Service Discovery: Automatically finds services without manual configuration.
- Security: Provides features like mutual TLS (mTLS) for encrypting service traffic.
- Observability: Metrics, logs, and traces that help you understand how services interact.
For more information, visit Service Mesh 101.
Understanding Service Mesh Complexity
Implementing a Service Mesh can have several complexities, such as:
- Configuration Overhead: Managing configurations for each service.
- Operational Burden: Increased demand for monitoring and maintaining mesh infrastructure.
- Steep Learning Curve: Skills needed to understand and utilize mesh features effectively.
While these complexities might seem daunting, several strategies can help you manage them effectively.
Strategies to Overcome Service Mesh Complexity
1. Start Small
Starting small is crucial for any large-scale implementation. Identifying a few microservices that would benefit significantly from a Service Mesh can yield immediate results.
# Example of a basic service mesh configuration snippet using Istio
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service
http:
- route:
- destination:
host: my-service
port:
number: 80
In this YAML definition, we define a VirtualService
for managing traffic routing to my-service
, allowing you to focus on microservices one step at a time.
2. Utilize Documentation and Community Resources
Modern Service Mesh implementations (like Istio, Linkerd, and Consul) come with extensive documentation. Take advantage of these resources, tutorials, and community support.
For example, Istio Documentation offers numerous guides for various use cases, helping you navigate complexities effectively.
3. Automation is Key
When configuring the Service Mesh, do not underestimate the power of automation. Use tools or scripts to manage configurations instead of doing it manually. Automation minimizes human error and ensures consistency in deployments.
For example, you could automate configuration using Terraform:
resource "kubernetes_manifest" "virtual_service" {
manifest = {
apiVersion = "networking.istio.io/v1alpha3"
kind = "VirtualService"
metadata = {
name = "my-service"
}
spec = {
hosts = ["my-service"]
http = [
{
route = [
{
destination = {
host = "my-service"
port = { number = 80 }
}
}
]
}
]
}
}
}
Automating your deployments and configurations using Infrastructure as Code (IaC) maximizes efficiency and reduces overhead.
4. Monitor and Observe
Implementing observability tools will help mitigate issues before they escalate. Tools like Prometheus and Grafana can be integrated into your Service Mesh to gather metrics on service performance.
Example code using Prometheus metrics in a Spring Boot service:
@SpringBootApplication
public class MyServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MyServiceApplication.class, args);
}
@Bean
public MeterRegistryCustomizer<MeterRegistry> metricsCommonTags() {
return registry -> registry.config().commonTags("application", "my-service");
}
}
To learn more about observability tools, check out Prometheus Monitoring and Grafana.
5. Embrace Service Discovery
Service discovery can help ease the configuration overhead that comes with managing service endpoints. Most Service Mesh solutions offer built-in service discovery.
This feature automatically registers services, allowing you to reference them by logical names instead of physical locations, reducing the complexity of dynamic environments.
6. Focus on Security Early
With ease of connectivity comes the necessity for security. Security must be prioritized in the early stages of your Service Mesh implementation. Utilizing mTLS for service-to-service communication can prevent unauthorized access and data leaks.
Sample Istio Configuration for mTLS:
apiVersion: security.istio.io/v1beta1
kind: PeerAuthentication
metadata:
name: my-service
spec:
mtls:
mode: STRICT
Implementing strict mTLS between services ensures that all service communications are secure, greatly reducing your security risks.
Closing Remarks
While implementing a Service Mesh can seem complex, breaking down the process and focusing on key strategies can simplify your journey. By starting small, leveraging documentation, automating configurations, implementing observability, adopting service discovery, and focusing on security, you can turn the complex into manageable pieces.
Embracing these principles will aid you in achieving a robust microservices architecture, offering not just reduced complexity but also enhanced performance and security.
If you're interested in diving deeper into the world of Service Mesh, consider reading A Comprehensive Guide to Service Mesh and enhance your knowledge further.
Let's embrace the future of microservices, equipped with the tools and strategies to overcome any complexity that comes our way. Happy coding!
Checkout our other articles