Common Mistakes When Mapping Data with MapStruct
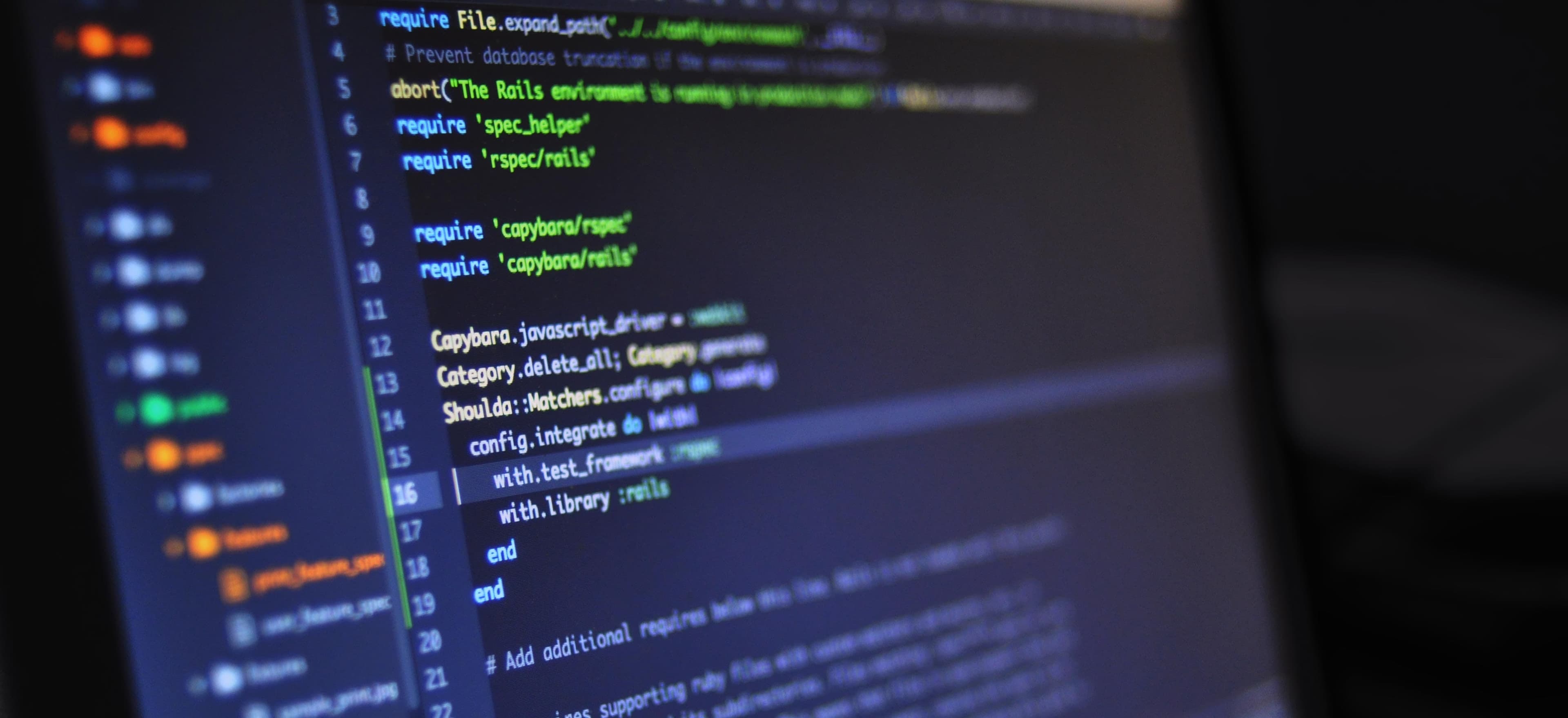
- Published on
Common Mistakes When Mapping Data with MapStruct
Data mapping is a vital aspect of software development, especially in Java applications. One of the most powerful tools for mapping data between different object models is MapStruct. It offers a code-generation approach, allowing developers to create efficient and clean mappers that transform data between different layers of an application. However, despite its benefits, developers can fall into several common pitfalls when using MapStruct. In this post, we will explore these mistakes in-depth and how to avoid them.
Understanding MapStruct
Before we dive into the common mistakes, let's outline what MapStruct does:
- Simple Annotation-Based Mapping: MapStruct uses simple annotations to define how Java objects map to one another.
- Compile-Time Checks: It generates the mapping code at compile time, leading to fewer runtime errors and improved performance.
- Highly Customizable: Developers can customize the mappings, including complex scenarios involving nested objects and collection handling.
A basic example of MapStruct is as follows:
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
UserDTO userToUserDTO(User user);
}
In the example above, a mapper interface defines a method to convert a User
object into a UserDTO
. MapStruct handles the implementation automatically during the build process.
Common Mistakes
1. Ignoring Null Safety
One of the most common mistakes developers make is not handling null values. When mapping objects, properties may be null, leading to NullPointerExceptions
.
Solution: Use the @Mapping
annotation to define what happens when values are null:
@Mapper
public interface UserMapper {
@Mapping(target = "age", defaultValue = "0") // Avoids NullPointerException
UserDTO userToUserDTO(User user);
}
In this example, providing a defaultValue
for the age
field ensures that if the user age is null, it defaults to 0 instead of causing an exception.
2. Failing to Use @Mapping
for Property Name Mismatches
When the source and target property names are not identical, developers often forget to use @Mapping
to specify how they relate. This oversight can lead to incomplete or incorrect mappings.
Example:
public class User {
private String firstName;
private String lastName;
}
public class UserDTO {
private String name; // Should be mapped from firstName and lastName
}
Solution: Explicitly define the mapping:
@Mapper
public interface UserMapper {
@Mapping(target = "name", expression = "java(user.getFirstName() + \" \" + user.getLastName())")
UserDTO userToUserDTO(User user);
}
By using an expression, we ensure that the first and last name are properly concatenated to form the full name in the DTO.
3. Not Handling Nested Mappings
Another mistake occurs during the mapping of nested objects. Developers often forget to create separate mapping methods for the nested fields, leading to data loss or incomplete DTOs.
Example:
public class Order {
private User user;
}
public class OrderDTO {
private UserDTO user; // Need a separate mapper for User
}
Solution: Create a dedicated mapping for the nested user:
@Mapper(uses = UserMapper.class)
public interface OrderMapper {
OrderDTO orderToOrderDTO(Order order);
}
By defining uses = UserMapper.class
, MapStruct knows to utilize the existing UserMapper
when mapping nested objects.
4. Overlooking Collection Mappings
When mapping collections, developers often overlook how MapStruct handles it. If not set up correctly, it might not map lists to lists or sets to sets, resulting in data inconsistencies.
Solution: Ensure you define collection mappings correctly:
public class User {
private List<Order> orders;
}
public class UserDTO {
private List<OrderDTO> orders;
}
@Mapper
public interface UserMapper {
List<OrderDTO> ordersToOrdersDTO(List<Order> orders);
}
In this case, ensure proper mapping functions are detailed for collections to avoid runtime issues.
5. Mismatched Types
Type mismatches between source and target objects can lead to mapping failures. For example, if you have a numeric type in the source and a string type in the target, MapStruct cannot automatically convert this.
Solution: Explicitly handle the conversion using mapping methods:
@Mapping(target = "age", source = "ageInt")
int convertAge(String ageInt);
In this example, a method to convert age from a string to an integer can be defined separately, or a custom converter class can be utilized.
6. Neglecting Custom Mappings for Complex Objects
Complex object structures often require custom logic in their mapping. Developers might resort to using default behaviors or ignore the need for mapping altogether.
Solution: Use custom methods for complex mappings.
@Mapper
public interface UserMapper {
@Mapping(target = "address", source = "address", qualifiedByName = "mapAddress")
UserDTO userToUserDTO(User user);
@Named("mapAddress")
static String mapAddress(Address address) {
if (address == null) {
return null;
}
return address.getStreet() + ", " + address.getCity();
}
}
Here, a custom mapping method mapAddress
is defined, ensuring address formatting is handled correctly.
7. Skipping the Use of Components
MapStruct allows for component-based mapping by specifying multiple mappers through the uses
attribute. Often, developers fail to leverage this feature, resulting in redundant code.
Solution: Create clear and reusable mappers.
@Mapper(uses = {UserMapper.class, AddressMapper.class})
public interface SystemMapper {
UserDTO mapUser(User user);
}
In this solution, using multiple mappers centralizes your mapping logic, allowing for cleaner code and reusability.
Closing the Chapter
While MapStruct is a powerful tool for Java developers, its effective use requires an understanding of its features and potential pitfalls. By avoiding common mistakes such as neglecting null safety, failing to manage nested mappings, and overlooking collection behavior, you can harness the full capabilities of MapStruct for efficient data mapping.
Make sure to leverage the options available, such as default values, custom methods for complex mappings, and collection handling. Doing so will lead to clearer code, improved performance, and seamless integration between different data models in your Java application.
For further reading on MapStruct, consider visiting the official documentation or exploring tutorials and sample projects that highlight common use cases.
Happy mapping!