Top Tips to Spot Talented Java Developers for Your Project
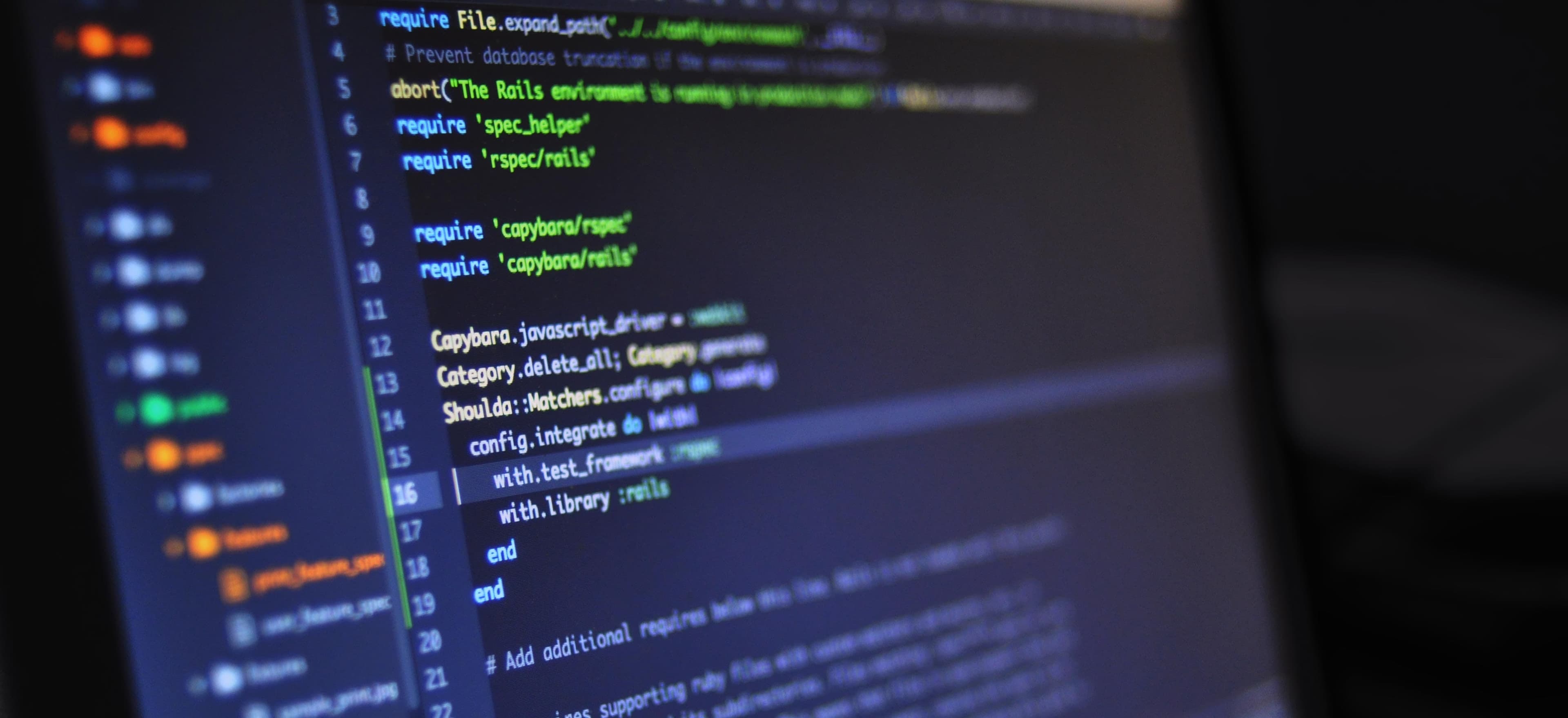
- Published on
Top Tips to Spot Talented Java Developers for Your Project
In today’s digital landscape, finding the right Java developer can significantly impact your project's success. From enterprise solutions to mobile applications, Java remains a dominant programming language due to its versatility and robust ecosystem. However, with countless developers claiming expertise, how can you discern the truly talented ones? In this blog post, we’ll explore key strategies and criteria for identifying top-notch Java developers for your project.
Understanding Java Expertise
Before we dive into the specific tips, it is important to understand what a talented Java developer entails. Talented developers do not only write code; they create maintainable, scalable, and efficient systems. They have a strong grasp of Java fundamentals, frameworks, tools, and the software development lifecycle.
1. Assessing Core Java Skills
A fundamental understanding of core Java concepts is non-negotiable. When interviewing candidates, focus on these critical areas:
-
OOP Principles: Candidates should demonstrate proficiency in Object-Oriented Programming (OOP) concepts, namely encapsulation, inheritance, and polymorphism. These principles form the backbone of Java application design.
-
Java APIs and Libraries: A good developer should be familiar with standard Java libraries such as Java Collections Framework and Java Streams, among others.
Example Code Snippet: Understanding OOP
class Animal {
String name;
Animal(String name) {
this.name = name;
}
void speak() {
System.out.println(name + " makes a sound.");
}
}
class Dog extends Animal {
Dog(String name) {
super(name);
}
@Override
void speak() {
System.out.println(name + " barks.");
}
}
In this code, we define two classes: Animal
and Dog
. The Dog
class extends Animal
, showcasing the principle of inheritance. Candidates should be able to explain the purpose of super
and method overriding.
2. In-Depth Knowledge of Java Frameworks
Most Java applications today are built using frameworks like Spring, Hibernate, or JavaServer Faces (JSF). A talented developer should have demonstrable experience with at least one of these frameworks.
Why Frameworks Matter
Frameworks simplify complicated tasks, promote code reusability, and enhance productivity. For instance, Spring provides vital features such as Dependency Injection and Aspect-Oriented Programming.
3. Problem-Solving Skills
Not all developers are adept at problem-solving, which is crucial for a project’s success. Use coding challenges or take-home assignments to assess candidates’ capabilities.
Turn to platforms like HackerRank to set up coding tests that mimic real-world scenarios. Look for:
- Algorithmic Thinking: Can they develop efficient algorithms?
- Debugging Skills: How quickly can they spot and fix bugs?
Sample Problem
Ask the candidate to solve for the factorial of a number using recursion. The candidate's solution could look like this:
public static int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
The recursive solution leverages the concept of breaking down a problem into smaller, more manageable parts, showcasing their understanding of recursion.
4. Code Quality and Best Practices
Evaluate whether developers adhere to coding standards and best practices. Talented developers should understand concepts like:
- Code Reviews: They should appreciate the importance of peer reviews and feedback.
- Testing: Familiarity with JUnit or Mockito for test-driven development.
Example Code Snippet: Unit Testing with JUnit
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class MathUtilsTest {
@Test
void testFactorial() {
assertEquals(120, MathUtils.factorial(5));
}
}
This unit test checks the integrity of the factorial
method. A committed developer embraces testing as part of their workflow and is comfortable using testing frameworks.
5. Communication Skills
Technical prowess is essential, but soft skills are equally important. A talented Java developer should effectively articulate ideas and explain their coding decisions to team members and stakeholders.
Behavioral Interview Questions:
- “Describe a time you faced a technical challenge and how you resolved it.”
- “How do you approach code reviews?”
6. Cultural Fit
Finally, consider the importance of cultural fit. A skilled developer may excel technically, but they must align with your team’s values and working style. Engage in conversations about their previous team experiences and project management methodologies they are accustomed to, such as Agile or Scrum.
Closing Remarks
Spotting talented Java developers involves a multifaceted evaluation process. It’s about more than technical skills; you need a developer who embodies quality, communicates efficiently, and fits well into your team culture.
Incorporate these tips into your hiring strategy, and you’ll be better equipped to select the best talent for your Java projects. If you are keen to deepen your understanding of Java and its frameworks, consider exploring Official Java Documentation and engaging with communities like Stack Overflow.
By prioritizing these areas, you ensure that your next project not only meets technical requirements but also thrives with a dedicated and talented team behind it. Happy hiring!