Are Integration Tests Really Worth the Effort?
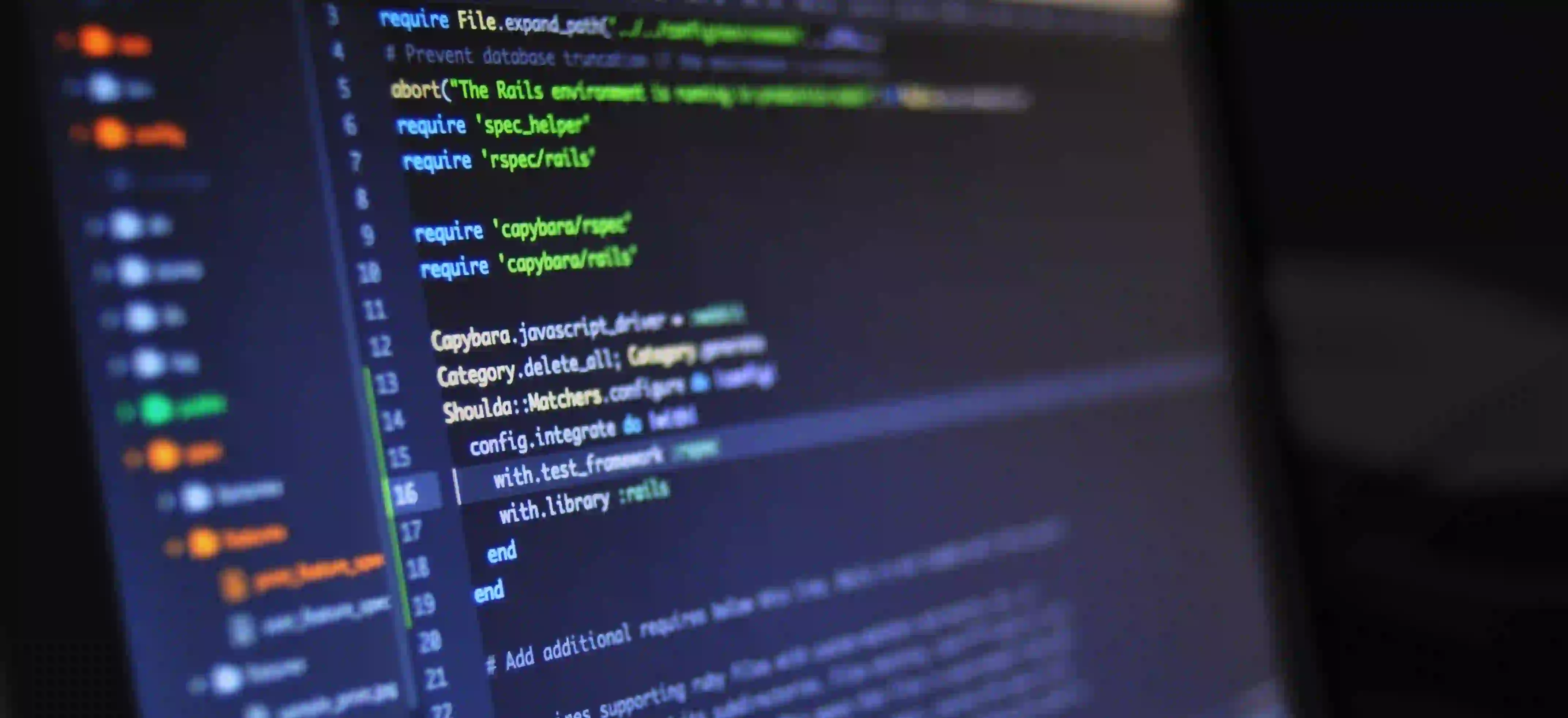
Are Integration Tests Really Worth the Effort?
In the world of software development, particularly in Java, testing is a fundamental component of creating robust applications. Among the myriad of testing strategies, integration testing stands out for its unique ability to validate the interaction between various components of an application. But are integration tests really worth the effort? Let’s explore this question in-depth.
What Are Integration Tests?
Integration tests are designed to ensure that different modules or services work together correctly. They go beyond unit tests, which focus on individual parts of the code, to assess how these components interact in a broader context.
Why Integration Tests Matter
- Catching Issues Early: By testing how components integrate, you can catch issues related to data flow between modules which might not be evident in unit tests.
- High-Level Validation: Integration tests validate the end-user experience by ensuring that the features work as expected when all components function together.
- Reduced Debugging Time: Identifying interaction bugs can significantly reduce the time spent debugging later phases of development.
Types of Integration Tests
Understanding the different types of integration tests can help clarify their utility:
-
Big Bang Integration Testing: All components are integrated simultaneously. While simple, it may complicate debugging if issues arise.
-
Top-Down Integration Testing: Testing starts from the top layer and progressively integrates lower levels. This can allow early detection of design flaws.
-
Bottom-Up Integration Testing: The lowest-level components are tested first, allowing gradual integration. This is useful for ensuring foundational components work before adding complexity.
-
Sandwich Testing: Combines both top-down and bottom-up approaches. This can be beneficial for large applications with complex interactions.
For more on testing strategies, consider reading this insightful article.
Real-World Use Cases
Example 1: Microservices Architecture
In a microservices architecture, services are independently developed and deployed. Integration tests are vital to ensure these services communicate effectively. For example, consider a simple e-commerce platform with services for user management, product catalog, and payment processing. An integration test could verify that a new user is correctly processed through the user service and the order can be placed successfully through the payment service.
@Test
public void testUserRegistrationAndOrderPlacement() {
// Simulating user registration
User user = new User("john.doe@example.com", "password123");
userService.register(user);
// Simulating product addition and order placement
Product product = new Product("Laptop", 999.99);
orderService.addProduct(product);
Order order = orderService.placeOrder(user.getId());
// Validating order status
assertEquals(OrderStatus.CONFIRMED, order.getStatus());
}
Why This Matters: This code demonstrates how integration tests can verify a user’s journey through multiple services, ensuring that all pieces fit together, which can help identify issues earlier in the process.
Example 2: Database Interaction
Consider an application that interacts with a database. Integration tests are essential for verifying that data is correctly saved and retrieved from a database. Here’s a snippet that shows how this could be approached:
@Test
public void testProductPersistence() {
Product product = new Product("Phone", 599.99);
// Saving the product to the database
productService.save(product);
// Retrieving the product from the database
Product retrievedProduct = productService.findById(product.getId());
// Validating the integrity of the data
assertEquals("Phone", retrievedProduct.getName());
assertEquals(599.99, retrievedProduct.getPrice(), 0.01);
}
Why This Matters: This test not only verifies that the save and retrieve operations work but also helps ensure that the application's data layer functions as intended.
Benefits of Integration Testing
1. Improved Code Quality
Each integration test you write forces you to consider how your components are linked. This often leads to better design decisions and a more maintainable codebase.
2. Enhanced Collaboration
When integration tests are a part of your Continuous Integration/Continuous Deployment (CI/CD) pipeline, they facilitate better team collaboration. Developers can confidently make changes knowing that existing functionalities will be validated automatically.
3. Realistic Test Scenarios
Integration tests simulate real-world usage scenarios much more closely than unit tests can, providing a more reliable safety net as you develop.
Potential Downsides
While integration tests are beneficial, they do come with their own set of challenges:
- Time-Consuming: Setting up integration tests can require a significant investment of time and resources.
- Complexity: The more components involved, the more complex the tests can become. This may lead to harder-to-read tests.
- Maintenance: As your application evolves, integration tests need to be updated, which can create a maintenance burden.
Best Practices
To maximize the value of your integration tests, consider the following best practices:
-
Consistent Environment: Ensure tests are run in a consistent environment to avoid discrepancies in results.
-
Use Mocks Wisely: Leverage mocking frameworks to simulate interactions where complete components aren't necessary. This can reduce complexity and speed up tests.
-
Create Clear, Focused Tests: Aim for specificity. Each integration test should focus on a single feature or interaction to simplify debugging.
-
Integrate with CI/CD: Integrating your tests into your pipeline ensures that they run automatically and catch issues early in the development cycle.
-
Document Your Intentions: Good documentation helps future developers understand the purpose behind each test case.
The Closing Argument
In conclusion, the question of whether integration tests are worth the effort can largely be answered with a resounding yes. Despite their upfront costs and ongoing maintenance requirements, the long-term benefits of improved code quality, enhanced collaboration, and more realistic testing scenarios make them an invaluable component of a comprehensive testing strategy.
As with any testing methodology, balance is key. Use integration tests in conjunction with unit tests for the best results. By fostering a culture of testing, you can create a more reliable application and a more efficient development process.
For those interested in taking their Java testing capabilities to the next level, consider diving deeper into existing frameworks that can help streamline the process. Happy coding!