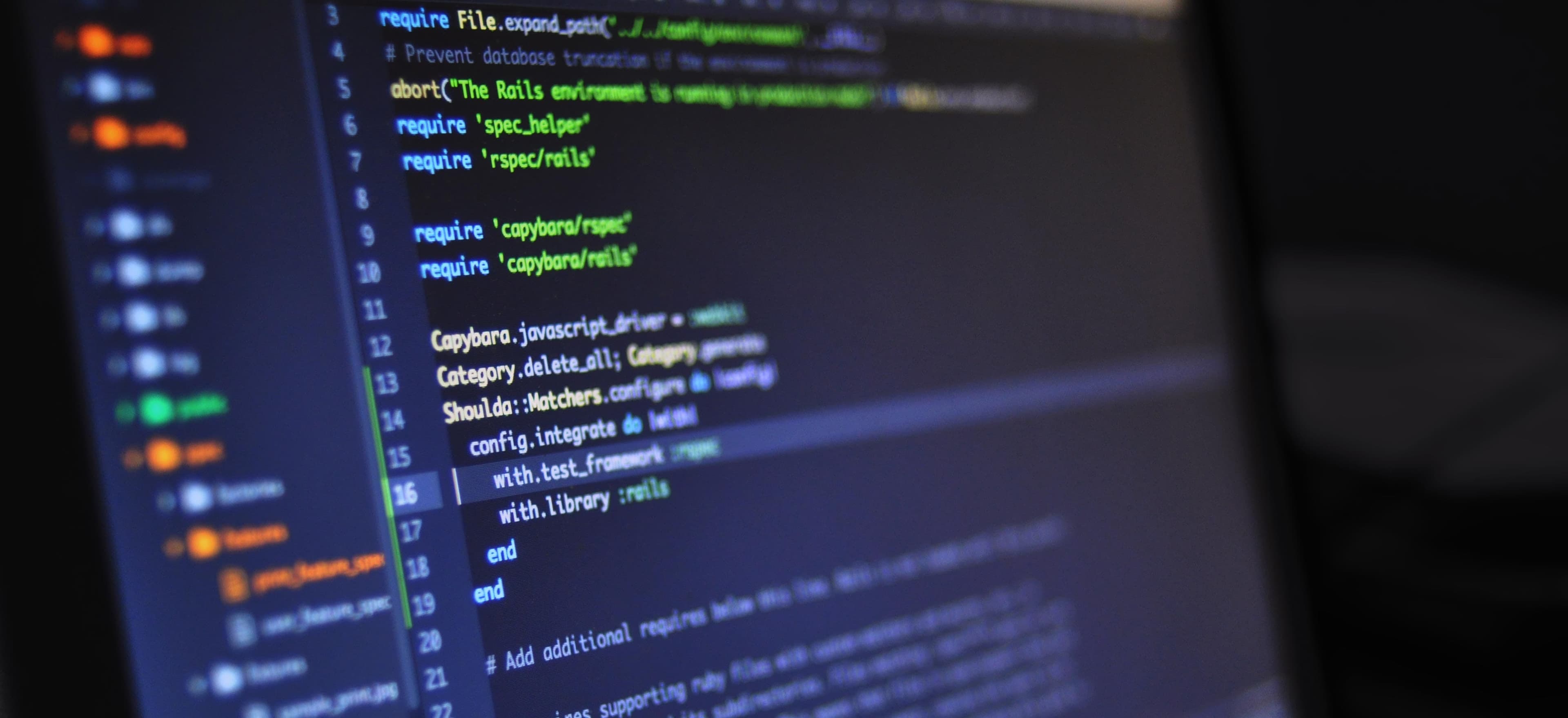
- Published on
Managing Multiple Security Realms in Spring Security
Spring Security is a powerful and customizable authentication and access control framework for Java applications. As application requirements grow, it is common to have different security realms that serve specific purposes. In this blog post, we will explore how to manage multiple security realms in Spring Security effectively.
Why Use Multiple Security Realms?
Multiple security realms can provide several advantages, such as:
- Separation of Concerns: Different areas of your application might require distinct authentication mechanisms.
- Enhanced Security: By having separate realms, you can apply varying security rules to different parts of your application, reducing the risk of a blanket security failure.
- Complex Applications: Larger applications may need to integrate with multiple domains or systems, each potentially requiring unique authentication processes.
Core Concepts of Spring Security
Before diving into managing multiple realms, it's crucial to understand a few fundamental concepts in Spring Security:
- Authentication: The process of determining whether someone is who they claim to be.
- Authorization: The process of determining if an authenticated user has permission to perform an action.
Setting Up Spring Security
To get started, ensure that your Spring project is set up with Spring Security dependencies. In a typical Maven setup, you can add the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Now that we have set up our environment, let's dive into configuring multiple security realms.
Configuring Multiple Security Realms
To configure multiple security realms, we need to follow these steps:
- Define Security Configurations: Create separate security configuration classes.
- Register the Authentication Manager: Use the
AuthenticationManager
to manage different authentication providers. - Specify Filter Chains: Define how different URL patterns are protected.
Step 1: Define Security Configurations
First, create separate security configuration classes for each realm. For example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class RealmOneSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user1").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}adminpass").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.antMatcher("/realm1/**")
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
In the example above, we configure RealmOneSecurityConfig
for the /realm1/**
endpoint. The @EnableWebSecurity
annotation allows Spring Security to provide an additional layer of protection.
Step 2: Register the Authentication Manager
Now, let’s create a second security configuration for another realm.
@Configuration
@EnableWebSecurity
public class RealmTwoSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user2").password("{noop}password2").roles("USER")
.and()
.withUser("superuser").password("{noop}superpass").roles("SUPERUSER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.antMatcher("/realm2/**")
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
Here, RealmTwoSecurityConfig
is handling authentication for the /realm2/**
endpoint with a different set of users and roles.
Step 3: Specify Filter Chains
Spring Security uses filter chains to decide which security configuration to apply based on the incoming request. The antMatcher
method in our configuration classes ensures that requests to /realm1/**
are handled by the first configuration, while requests to /realm2/**
are handled by the second configuration.
Step 4: Testing the Configurations
You can create REST controllers to test our security configurations.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class RealmController {
@GetMapping("/realm1/resource")
public String realmOneResource() {
return "You have accessed realm one resource!";
}
@GetMapping("/realm2/resource")
public String realmTwoResource() {
return "You have accessed realm two resource!";
}
}
With these endpoints in place, you can test your application by hitting the URLs /realm1/resource
and /realm2/resource
. To manipulate authentication, you can use tools like Postman or curl with the relevant credentials.
Final Thoughts
Managing multiple security realms in Spring Security enhances the security strategy of Java applications. By isolating the authentication and authorization logic into subnetworks defined by URL patterns, applications can become more secure and maintainable.
To learn more about Spring Security, check out the official documentation for comprehensive details and advanced configurations.
Feel free to comment below or reach out for any questions or clarifications on managing multiple security realms in Spring Security!
Happy coding!