Common Pitfalls in Setting Up Spring 3 Development Environment
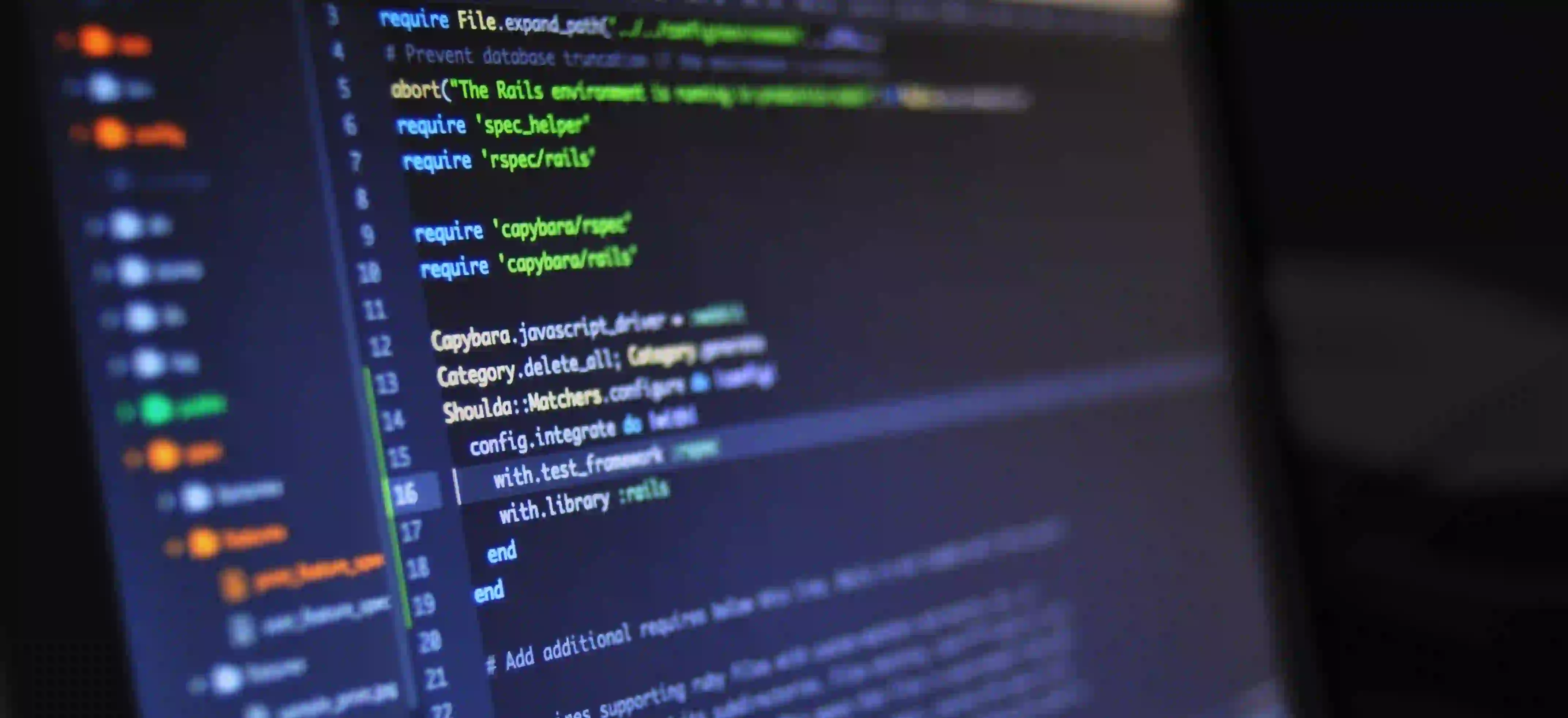
Common Pitfalls in Setting Up Spring 3 Development Environment
Setting up a development environment for Spring 3 can be a daunting task for both novices and seasoned developers alike. The power of Spring lies in its extensive feature set, which, if not configured correctly, can lead to significant issues. In this guide, we will discuss common pitfalls and how to navigate them effectively, ensuring a smoother Spring 3 development experience.
1. Improper Dependency Management
Explanation:
One of the most frequent mistakes is mismanaging project dependencies. Spring 3 requires specific versions of various libraries, and using incompatible ones can lead to ClassNotFoundException or NoSuchMethodError.
Best Practices:
-Leverage Maven or Gradle for dependency management. These tools help define dependencies and their versions in a centralized manner.
-Ensure that your pom.xml
or build.gradle
contains the necessary Spring dependencies, like this example in Maven:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>3.2.18.RELEASE</version>
</dependency>
Why?
Using Maven or Gradle helps to avoid version conflicts. These tools manage transitive dependencies and ensure that the correct versions of libraries are fetched.
2. Not Configuring the Application Context Properly
Explanation:
Spring's application context is fundamental for managing beans. Failing to set it up correctly can cause your application not to start or behave unexpectedly.
Best Practices:
-Carefully review and ensure your XML or Java-based configuration files are correct. Here's an example of a simple XML configuration:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myBean" class="com.example.MyBean" />
</beans>
Why?
Properly configuring the application context ensures that Spring can manage your beans effectively. Misconfigurations lead to runtime errors that can be hard to debug.
3. Ignoring Dependency Injection Best Practices
Explanation:
One of Spring's core features is dependency injection (DI). Failing to utilize DI properly can result in tightly coupled code.
Best Practices:
-Use constructor injection for mandatory dependencies and setter injection for optional ones. Here is a basic illustration:
@Component
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
Why?
Constructor injection promotes immutability and ensures that a bean is created in a valid state. This is important for writing clean, testable code.
4. Misunderstanding the Scope of Beans
Explanation:
Spring allows defining the scope of beans (singleton, prototype, request, session). Ignoring this feature could lead to unexpected behaviors, especially in web applications.
Best Practices:
-Define bean scopes appropriately in XML or using annotations. Here is an example using annotations:
@Service
@Scope("prototype")
public class MyService {
// Prototype-scoped service bean
}
Why?
Choosing the correct scope helps manage the lifecycle of beans correctly in your application. Singleton beans are shared, while prototype beans are created anew with each request.
5. Not Leveraging Spring Profiles
Explanation:
If you have different environments (development, testing, production), failing to use Spring profiles can result in configuration mishaps.
Best Practices:
-Define profiles in your configuration files to separate environment-specific configurations. For example:
<beans profile="dev">
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="url" value="jdbc:h2:mem:testdb" />
<property name="driverClassName" value="org.h2.Driver" />
</bean>
</beans>
Why?
Spring profiles help in maintaining clean and organized configurations for each environment. It prevents the risk of deploying development settings to production.
6. Overlooking Exception Handling Mechanisms
Explanation:
Many developers may overlook exception handling features provided by Spring. This can lead to uncaught exceptions and application crashes.
Best Practices:
Utilize Spring’s @ControllerAdvice
for exceptions in web applications:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why?
Implementing proper exception handling provides a centralized way to manage errors within your application, improving reliability and user experience.
7. Failing to Test Your Application
Explanation:
Testing is crucial, and neglecting to write tests for your configuration or services can lead to hard-to-find bugs later on.
Best Practices:
-Leverage Spring’s testing support with JUnit or TestNG. Example of a simple test case:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = AppConfig.class)
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
// Assert some expected outcomes from myService
}
}
Why?
Testing ensures that your application behaves as expected. It acts as a safety net when making changes or adding new features.
8. Over-Complicating the Configuration
Explanation:
While Spring is powerful, over-complicating configurations can lead to confusion and maintenance challenges.
Best Practices:
-Start with simple configurations and gradually introduce complexity as needed. Stick to prevalent design patterns.
Why?
Simpler configurations are easier to read, maintain, and debug. They help new developers grasp the project structure more easily.
Wrapping Up
Setting up a Spring 3 development environment requires careful attention to detail. By avoiding these common pitfalls, you can streamline your setup process and focus on building robust applications. Remember, a well-configured environment can significantly reduce debugging time and improve the overall quality of your code.
For more in-depth information, refer to the official Spring Documentation.