Mastering Validation: Overcoming REST API Pitfalls
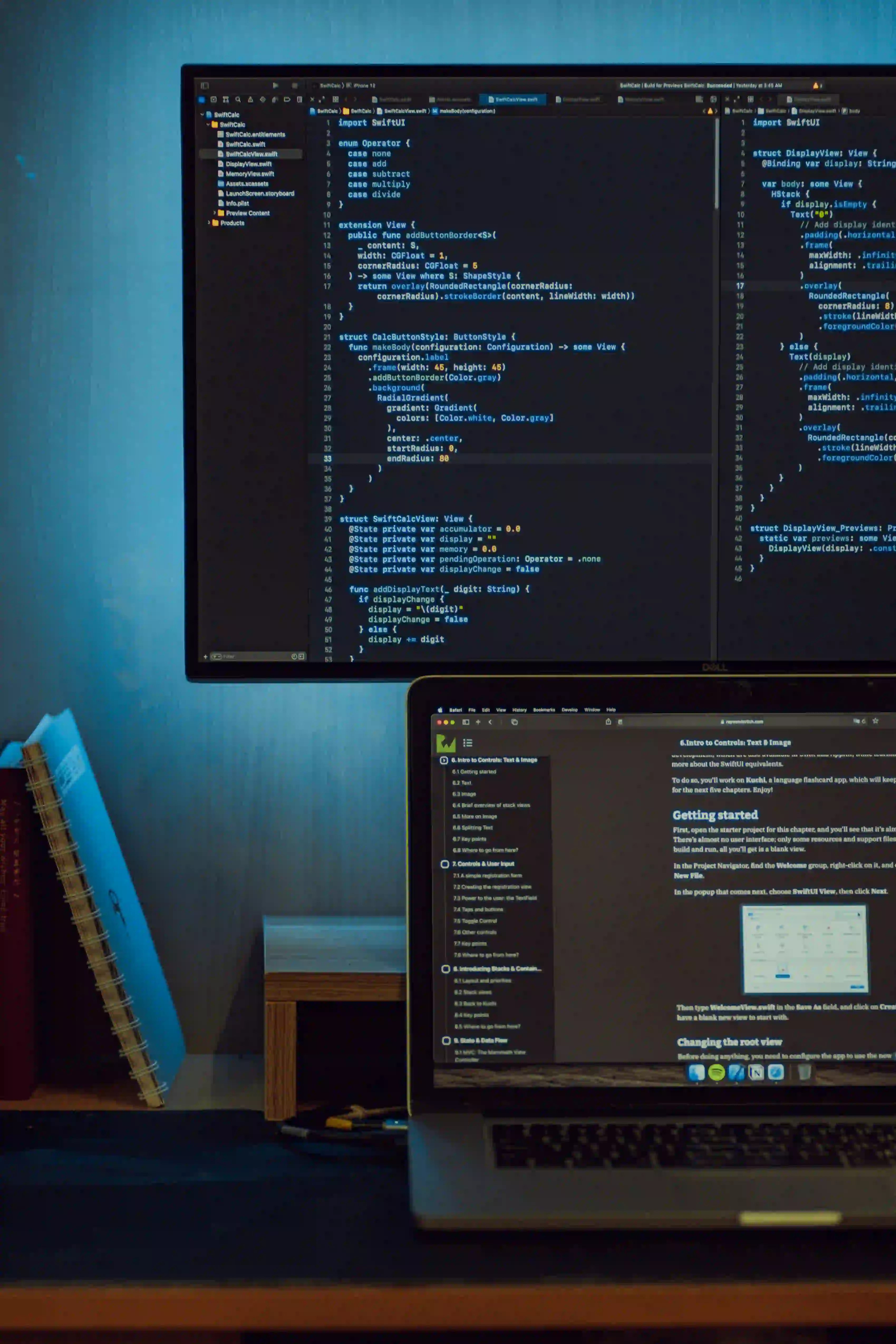
Mastering Validation: Overcoming REST API Pitfalls
In the world of software development, particularly when it comes to building REST APIs, proper data validation is paramount. Ensuring that your API handles input correctly can mean the difference between a reliable application and one riddled with errors. With increasing reliance on APIs for mobile apps, web front ends, and microservices, mastering validation is not just a recommended practice; it is a necessity.
In this blog post, we will delve into validation techniques, explore common pitfalls, and present strategies for overcoming these challenges using Java.
Understanding REST API Validation
Validation is the process of checking incoming data to ensure it meets the required criteria. In a REST API, this typically involves:
- Verifying that required fields are present.
- Ensuring that the data types match expectations.
- Checking for acceptable value ranges.
- Sanitizing input data to prevent injection attacks.
Ultimately, effective validation shields your application from invalid data, security vulnerabilities, and system crashes.
Importance of Validation
Poor validation practices can lead to various issues:
- Security Flaws: Improperly validated inputs can expose your application to threats like SQL injection or XSS attacks.
- Data Integrity: Accepted invalid data can severely corrupt your database, leading to erroneous results.
- User Experience: Without proper feedback on what went wrong, users are left frustrated.
For a deeper understanding of security threats, check out the article titled "Common Security Vulnerabilities in APIs".
Common Pitfalls in REST API Validation
1. Lack of Consistency in Validation
Different endpoints might use varying methods for validation, leading to inconsistencies. This can confuse developers and reduce trust in the API. Ensure validation logic is consistent across the API.
2. Relying on Client-Side Validation Alone
Client-side validation can enhance user experience but should not be relied upon for security. Clients can bypass it easily. Always validate on the server side before processing any data.
3. Ignoring Error Handling
Failing to provide meaningful error responses can hinder debugging and diminish user experience. Always communicate what went wrong and how to fix it.
4. Overly Complex Validation Logic
Validation should be straightforward. Overly complicated logic introduces needless complexity that can easily break down.
Strategies for Effective Validation in Java
Let’s discuss effective techniques for implementing validation in your REST API using Java, with a focus on simplicity and clarity.
1. Utilize Bean Validation (JSR 380)
Bean Validation is a powerful mechanism in Java that provides a standard way to validate object properties. It leverages annotations, making it relatively easy to implement. Below is a simple example of how to use Bean Validation.
import javax.validation.constraints.*;
public class User {
@NotNull(message = "Username cannot be null")
@Size(min = 3, max = 20, message = "Username must be between 3 and 20 characters")
private String username;
@Email(message = "Email should be valid")
@NotNull(message = "Email cannot be null")
private String email;
// Constructors, getters, and setters
}
Commentary:
@NotNull
ensures that the field must not be null.@Size
checks the user's length constraints.@Email
ensures the email format is valid.- Providing custom messages gives clarity on what failed during validation.
Next, you merely need to trigger the validation using the Validator
interface.
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
public class ValidationUtil {
public static void validate(Object object) {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set<ConstraintViolation<Object>> violations = validator.validate(object);
if (!violations.isEmpty()) {
for (ConstraintViolation<Object> violation : violations) {
System.out.println(violation.getMessage());
}
throw new IllegalArgumentException("Validation failed");
}
}
}
Commentary:
- The
Validator
validates the object and collects violations. - A clear error message is printed for each violation found.
2. Custom Validation Annotations
In some cases, the built-in annotations may not suffice. You may need to create custom validations. Here’s how to define a custom annotation.
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.*;
@Documented
@Constraint(validatedBy = PhoneValidator.class)
@Target({ElementType.METHOD, ElementType.FIELD, ElementType.ANNOTATION_TYPE, ElementType.PARAMETER})
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidPhone {
String message() default "Invalid phone number";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
For this annotation, we’ll provide a PhoneValidator
class to implement the logic.
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
public class PhoneValidator implements ConstraintValidator<ValidPhone, String> {
@Override
public void initialize(ValidPhone validPhone) { }
@Override
public boolean isValid(String phone, ConstraintValidatorContext context) {
return phone != null && phone.matches("\\d{10}");
}
}
Commentary:
- The
isValid
method contains the validation logic for phone numbers. - We ensure the phone number is exactly ten digits.
3. Centralize Error Handling with @ControllerAdvice
To construct coherent error messages across the API, use @ControllerAdvice
, which enables global exception handling in Spring applications.
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(IllegalArgumentException.class)
public ResponseEntity<String> handleValidationExceptions(IllegalArgumentException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.BAD_REQUEST);
}
}
Commentary:
- This handler will intercept all
IllegalArgumentException
instances thrown from anywhere in the application, centralizing our error handling approach and improving code maintainability.
4. Testing Your Validation Logic
Never underestimate the power of thorough testing. Unit tests help verify that your validation logic is correctly implemented and handles edge cases.
Here’s a straightforward test case using JUnit:
import org.junit.jupiter.api.Test;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import static org.junit.jupiter.api.Assertions.*;
public class UserValidationTest {
private Validator validator;
public UserValidationTest() {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
}
@Test
public void testValidUser() {
User user = new User("validUser", "user@example.com");
Set<ConstraintViolation<User>> violations = validator.validate(user);
assertTrue(violations.isEmpty(), "Should be no validation errors");
}
@Test
public void testInvalidUser() {
User user = new User("", "invalid-email");
Set<ConstraintViolation<User>> violations = validator.validate(user);
assertFalse(violations.isEmpty(), "Should be validation errors");
}
}
Commentary:
This test checks that a valid user has no violations, while an invalid user correctly reports errors. This enforces that your validation logic works as expected.
Closing Remarks
In conclusion, mastering validation for REST APIs is crucial for developing secure, reliable, and user-friendly applications. By understanding common pitfalls, utilizing Bean Validation, creating custom annotations, leveraging centralized error handling, and rigorously testing your logic, you can overcome the prevalent challenges faced in API development.
For further reading on REST API practices, consider checking out the informative guide from Martin Fowler on maturity in REST APIs.
Armed with these strategies, you are ready to face the complexities of API validation confidently. Always remember: a properly validated API is a gateway to user trust and application success. Happy coding!