Mastering Java ROP: Common Command Line Pitfalls
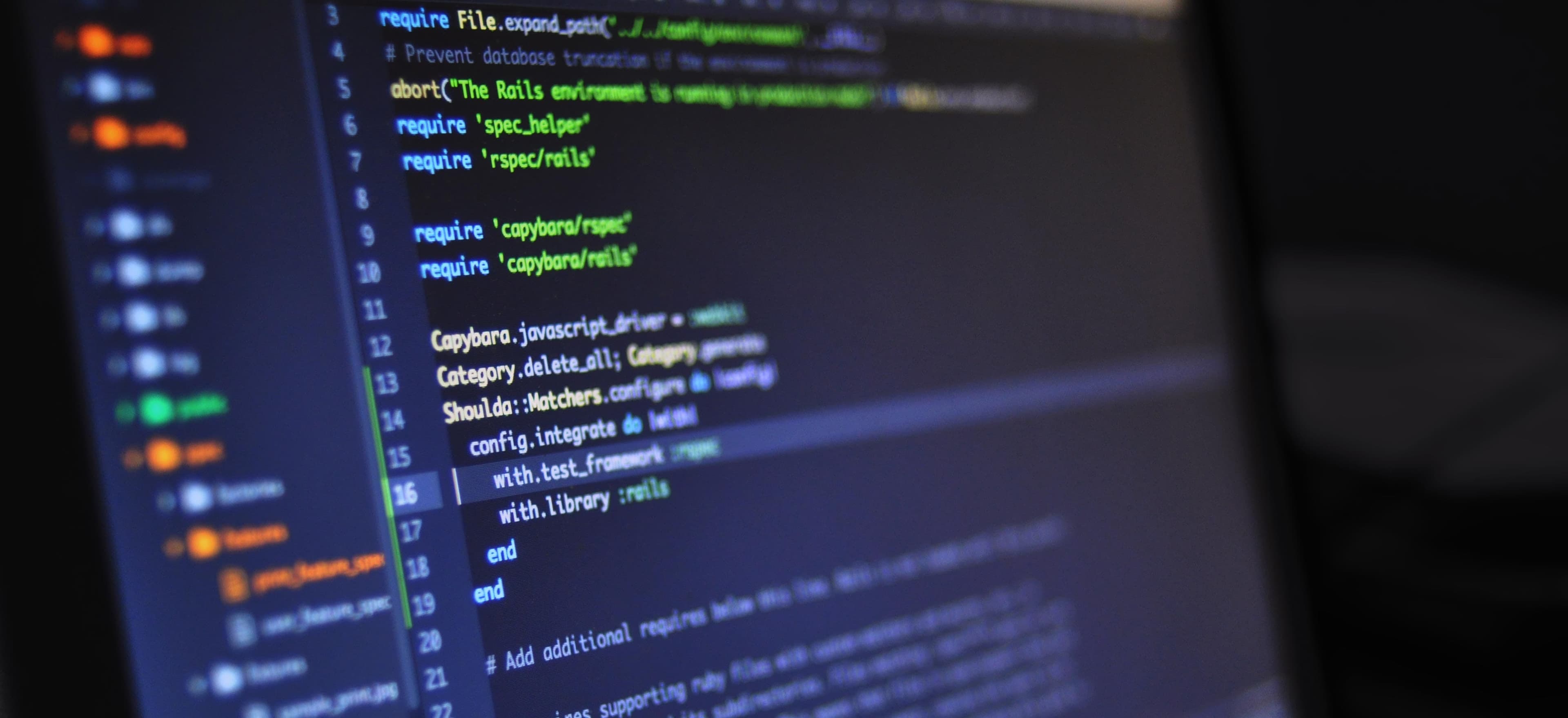
- Published on
Mastering Java ROP: Common Command Line Pitfalls
Java Rank Order Processing (ROP) can be a powerful tool in performance tuning and server management. However, mastering it involves navigating through various command line pitfalls that can impact your application's performance or even lead to unexpected behavior. In this guide, we will explore common pitfalls encountered when using the command line with Java ROP, and provide guidance on how to avoid them.
Understanding Java ROP
Before diving deep into common pitfalls, it's essential to have a solid understanding of what Java ROP is. In simple terms, ROP is a mechanism that helps applications efficiently process ordered elements. It is particularly prominent in data-intensive applications where performing operations in a certain order can significantly affect performance.
For those looking to deepen their understanding, consider checking out Java ROP Documentation.
Common Command Line Pitfalls
1. Incorrect JAVA_HOME Configuration
The Issue
One of the most common issues Java developers experience is an improperly set JAVA_HOME
environment variable. When this variable isn’t correctly configured, Java commands in your terminal may not work as expected, leading to runtime errors.
Solution
To check your JAVA_HOME
, you can execute:
echo $JAVA_HOME
Ensure that it points to the correct Java installation directory. For example, on UNIX systems, it should look something like this:
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64
2. Command Line Argument Parsing
The Issue
Java applications often require command-line arguments for configuration. A common pitfall occurs when arguments are improperly parsed, leading to application failures or unintended behavior.
Solution
Use the args
parameter in your main
method effectively. Here’s a simple example of parsing command-line arguments:
public class CommandLineExample {
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("No arguments provided");
return;
}
for (String arg : args) {
System.out.println("Argument: " + arg);
}
}
}
This code checks if any arguments are passed. If not, it notifies the user. Valid argument parsing is critical, as it allows you to handle configurations dynamically.
3. Misconfigured JVM Options
The Issue
When using the command line to launch your Java application, improper JVM options can lead to performance bottlenecks or crashes. The JVM options can control memory allocation, garbage collection, and debugging features.
Solution
Here is an example command with JVM options:
java -Xms512m -Xmx2g -XX:+UseG1GC -jar myapp.jar
-Xms512m
: sets the initial Java heap size to 512 MB.-Xmx2g
: limits the maximum Java heap size to 2 GB.-XX:+UseG1GC
: enables the G1 garbage collector, which is excellent for applications with large heaps.
Inappropriate settings could either waste memory or cause your application to run out of memory, so always monitor and modify these parameters as needed.
4. File Path Issues
The Issue
Working with files requires precise file paths. A common pitfall occurs when relative paths are misinterpreted, leading to FileNotFoundException
.
Solution
Always use absolute paths or ensure your working directory is correctly configured. Here’s how you might read a file in Java:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileReaderExample {
public static void main(String[] args) {
File file = new File("data/input.txt");
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
Using a try-with-resources
statement ensures that resources are closed after usage. Always handle exceptions gracefully, allowing you to pinpoint issues efficiently.
5. Ignoring Error Logs
The Issue
Another prevalent pitfall is overlooking error logs generated by your Java application. Early detection of errors is crucial for maintenance and performance.
Solution
Always redirect error and output with proper logging:
java -jar myapp.jar > output.log 2> error.log
This command separates standard output and error streams, allowing you to analyze issues more thoroughly.
Best Practices to Avoid Pitfalls
Use Version Control
Version control systems like Git can help you keep track of changes in your application. Keeping your codebase organized can significantly reduce confusion and potential errors when running from the command line.
Create a Shell Script for Common Tasks
Automating frequently executed commands can minimize the likelihood of errors.
#!/bin/bash
java -Xms512m -Xmx2g -XX:+UseG1GC -jar myapp.jar "$@"
Here, the script allows you to always launch your application with recommended JVM options while passing additional arguments seamlessly.
Regular Updates
Always keep your JDK updated. Performance improvements and security patches can help avoid potential issues down the line. For information on updates, refer to the official Java SE Updates page.
Bringing It All Together
Mastering Java ROP means being acutely aware of the command line pitfalls that can impact your application. By recognizing and addressing these pitfalls — from environment configuration and argument parsing to JVM tuning and file paths — you can ensure a smoother development process.
For deeper explorations into Java performance tuning, consider reading more about Java Performance Tuning for strategies and methods.
Incorporate these practices into your development workflow to not only enhance your Java development experience but also to deliver robust applications efficiently. Happy coding!