Mastering Debugging: Fixing Java Streams in IntelliJ
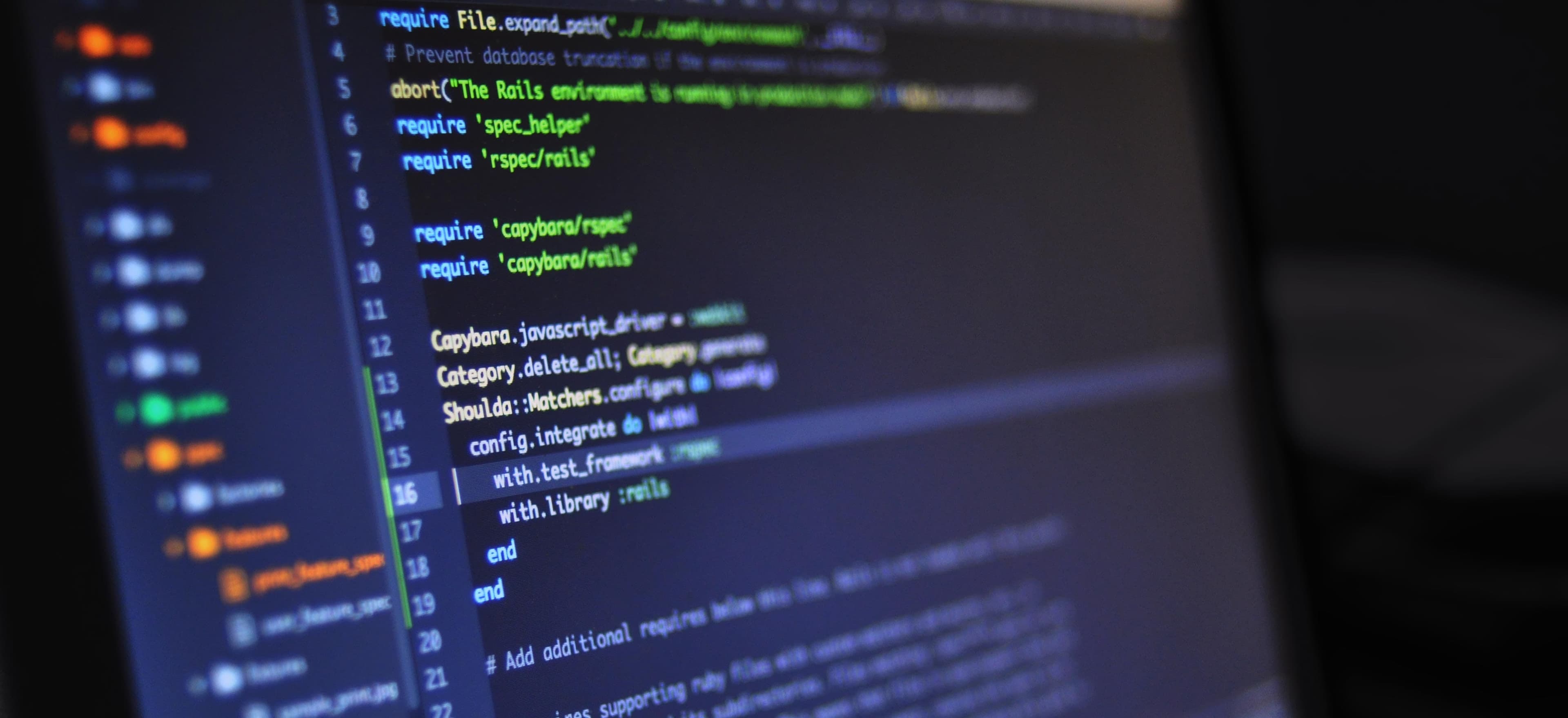
- Published on
Mastering Debugging: Fixing Java Streams in IntelliJ
Debugging is an essential skill for every developer. In the world of Java, leveraging streams has become a powerful way to handle collections of data. However, debugging streams can sometimes feel like navigating a labyrinth of complexities. This blog post will guide you through debugging Java streams in IntelliJ IDEA, providing you with practical insights and tips to sharpen your skills.
Understanding Java Streams
Java streams are a part of the Java 8 Stream API. They enable functional-style operations on sequences of elements, such as collections. Streams allow for operations like filtering, mapping, and reducing, making the code more concise and expressive.
Key Features of Java Streams
- Laziness: Streams are lazy; computations are not performed until absolutely necessary.
- Chaining Operations: You can chain multiple operations in a pipeline, which can lead to cleaner code.
- Parallel Processing: You can process large data sets efficiently using parallel streams.
To grasp the power of streams, consider this simple example of filtering a list of integers to keep only the even ones:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
Why This Code Works
- Stream Creation: We create a stream from the list of numbers.
- Filtering: The
filter
operation checks if each number is even, and only those that pass the check are included in the resulting stream. - Collection: Finally, the results are collected into a new list.
In this section, we’ve observed how Java streams make operations on collections intuitive. But how do we deal with issues that arise when things don't work as expected?
Debugging Java Streams in IntelliJ
Common Pitfalls in Stream Usage
Before diving into IntelliJ's debugging tools, let’s identify some common pitfalls with Java streams:
- Null Pointer Exceptions: Streams can throw exceptions if they encounter null values. Always ensure your collections do not contain null elements.
- Complex Operations: Chaining multiple operations can sometimes lead to unexpected results because of how data is transformed at each step.
- Stateful Operations: Operations like
sorted()
ordistinct()
might yield unexpected results if you don't understand the behavior of the stream.
Setting Up Your IntelliJ IDEA
IntelliJ IDEA is a powerful IDE that provides various tools for debugging. Here’s how to set it up for debugging Java streams:
- Running Your Application in Debug Mode: Start your application in debug mode by clicking the green bug icon next to the run button in IntelliJ.
- Setting Breakpoints: You can set breakpoints in your code where you want the execution to pause. Click in the gutter area next to a line number.
Example: Debugging a Stream Operation
Let’s take a closer look at debugging a complex stream operation. Consider the following modified version of our earlier example, where we attempt to filter even numbers, but mistakenly include an intention to handle null
values:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class DebuggingStream {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, null, 4, 5, null, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n != null && n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
Debugging Steps
-
Set a Breakpoint: Place a breakpoint at the line where the stream processes the collection.
-
Inspect Variables: Once the execution hits the breakpoint, hover over the
numbers
variable to inspect its values. You should see[1, 2, null, 4, 5, null, 6]
. -
Step Through Code: Use the "Step Into" feature to dive into the filter function. Check how values are being passed through.
-
Evaluate Expressions: IntelliJ allows you to evaluate expressions while debugging. You can check how the stream behaves with different filter conditions. For example, change
n -> n != null && n % 2 == 0
temporarily ton -> n != null
. -
View the Result: After running this original version, you will see how the presence of
null
values disrupts your expected results.
Best Practices for Debugging Streams
-
Use
peek()
for Reporting: You can use thepeek()
method to log intermediary results without altering the stream's final outcome.List<Integer> evenNumbers = numbers.stream() .peek(n -> System.out.println("Current value: " + n)) .filter(n -> n != null && n % 2 == 0) .collect(Collectors.toList());
-
Write Unit Tests: Unit tests can help identify issues early before you even need to debug. Use JUnit to write tests for different aspects of your stream logic.
Final Thoughts
Debugging Java streams in IntelliJ might seem challenging at first, but knowing the tools at your disposal can immensely ease the process. By setting breakpoints, inspecting collections at various stages, and understanding the flow of data, you can fix issues more effectively.
Remember, every bug is a learning opportunity. Whether you're identifying null pointer exceptions or working through complex state transformations, mastering these skills can lead to more robust code.
For more information on Java streams, consider checking the official Java documentation. Happy coding and debugging!
Checkout our other articles