Common Pitfalls When Creating Couchbase Views in Java
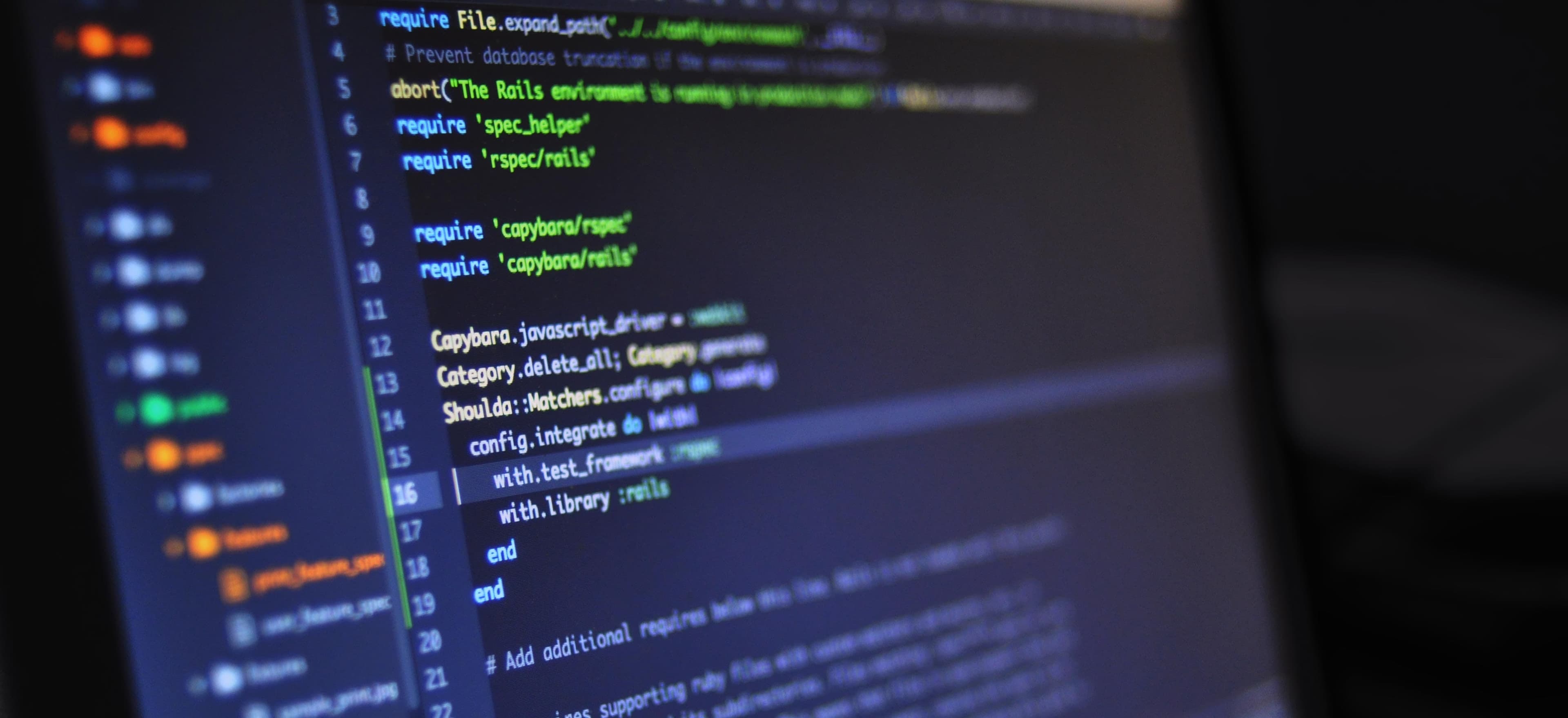
- Published on
Common Pitfalls When Creating Couchbase Views in Java
Couchbase is a powerful NoSQL database that provides robust data storage, enhanced performance, and real-time analytics. One of its key features is the ability to create MapReduce views to query data efficiently. However, developers can encounter several pitfalls when creating these views in Java. In this blog post, we’ll explore the common mistakes, how to avoid them, and best practices when working with Couchbase views in Java.
Understanding Couchbase Views
Before diving into pitfalls, it’s essential to understand what Couchbase views are. At its core, a view is a way to filter and aggregate documents stored in Couchbase using JavaScript functions. The Map function processes each document, while the Reduce function aggregates the results of the Map step.
Example of a Couchbase View
Here is a simple example of a view definition:
{
"views": {
"by_type": {
"map": "function (doc, meta) { if (doc.type) { emit(doc.type, null); } }"
}
}
}
The above view emits the type
field of each document. If it exists, the emitted value is null
. This is a common scenario for categorizing documents based on the type.
Common Pitfalls When Creating Couchbase Views
1. Neglecting Indexing
One of the most frequent pitfalls is forgetting to create indexes for the views. Without proper indexing, the queries may return slow performance, which affects your application's responsiveness.
How to Avoid
Always ensure that your views are indexed. Use the Couchbase Console or CLI to create the necessary indexes, or include them programmatically.
2. Not Handling Missing Fields
JavaScript functions in views must handle missing fields gracefully. If your documents do not always contain certain fields, a naive approach may lead to unexpected errors.
Example:
function (doc, meta) {
if (doc.type !== undefined) {
emit(doc.type, null);
}
}
In this modified code, we check for the existence of the type
field using undefined
. This retains reliability even when documents vary in their structure.
3. Using Reduce Functions Inappropriately
Another common mistake is misusing Reduce functions. Developers may overlook that Reduce functions are only executed after Map functions, potentially leading to confusion about data aggregation.
Reduce Function Example:
function (keys, values, rereduce) {
return sum(values);
}
This function takes the output from the Map phase and aggregates the data. Use Reduce cautiously, and decide if it's necessary for your use case.
4. Over-Loading Views
Creating a single view that does too much can introduce inefficiencies. Overloading views with excess logic or multiple emissions can drastically slow performance.
What To Do
Break complex views into simpler, focused views. This technique not only enhances performance but also simplifies the maintenance of individual views.
5. Ignoring Design Document Changes
When changes occur in the document structure or view definition, ensure you update the design document appropriately. Failing to do so may lead to runtime issues.
Example of an Updated Design Document
{
"views": {
"by_category": {
"map": "function (doc, meta) { if (doc.category) { emit(doc.category, null); } }"
}
}
}
6. Not Testing Views
Testing is paramount, yet some developers neglect to validate their views through rigorous testing. It can lead to unforeseen errors in production environments.
Recommended Approach
Use Couchbase’s query and view testing capabilities. Create unit tests to ensure views return expected outputs. This practice aids in identifying issues before deploying code changes.
// Example of a simple unit test for a Couchbase view
@Test
public void testView() {
ViewQuery query = ViewQuery.from("design_doc", "by_type").key("example_type");
ViewResult result = bucket.query(query);
assertNotNull(result);
assertTrue(result.size() > 0);
}
7. Overlooking Security and Permissions
As you define views and design documents, ensure you comply with security best practices. Each design document should be accessible only to properly authenticated users.
Recommended Practices
Utilize Role-Based Access Control (RBAC) capabilities in Couchbase to grant permissions judiciously. This avoids exposure of sensitive data.
8. Neglecting to Optimize Data Retrieval
Sometimes, developers retrieve more data than necessary due to poorly constructed queries. This can lead to bandwidth and performance issues.
Best Practice
Be specific in your Queries. Instead of fetching all data with a generic view, tailor your keys to retrieve only the necessary documents.
9. Failing to Monitor and Optimize Performance
Performance can degrade over time as your dataset grows. It is crucial to monitor view performance and optimize it accordingly.
Performance Monitoring
Leverage Couchbase Server's built-in analytics and monitoring to track query performances over time. Optimize views based on usage patterns.
10. Neglecting to Write Documentations
Documentation is often an afterthought. Poor documentation can lead to misunderstandings during maintenance and development.
Documentation Importance
Clearly document your view structures, logic, and anything relevant to its design. Use Markdown files or developer wikis to maintain clear visibility of design decisions.
In Conclusion, Here is What Matters
While Couchbase views are powerful tools, several common pitfalls can hinder your application’s performance and reliability. Avoiding these pitfalls through proper indexing, handling missing fields, using reduce functions correctly, and more will enhance your Couchbase experience.
For more in-depth information, you can check the official Couchbase View documentation to familiarize yourself with best practices.
By staying mindful of these potential issues, you can create a robust database structure that scales effortlessly with your application's needs.
Happy coding!