Mastering SQL Date and Timezone Queries: Your Ultimate Guide
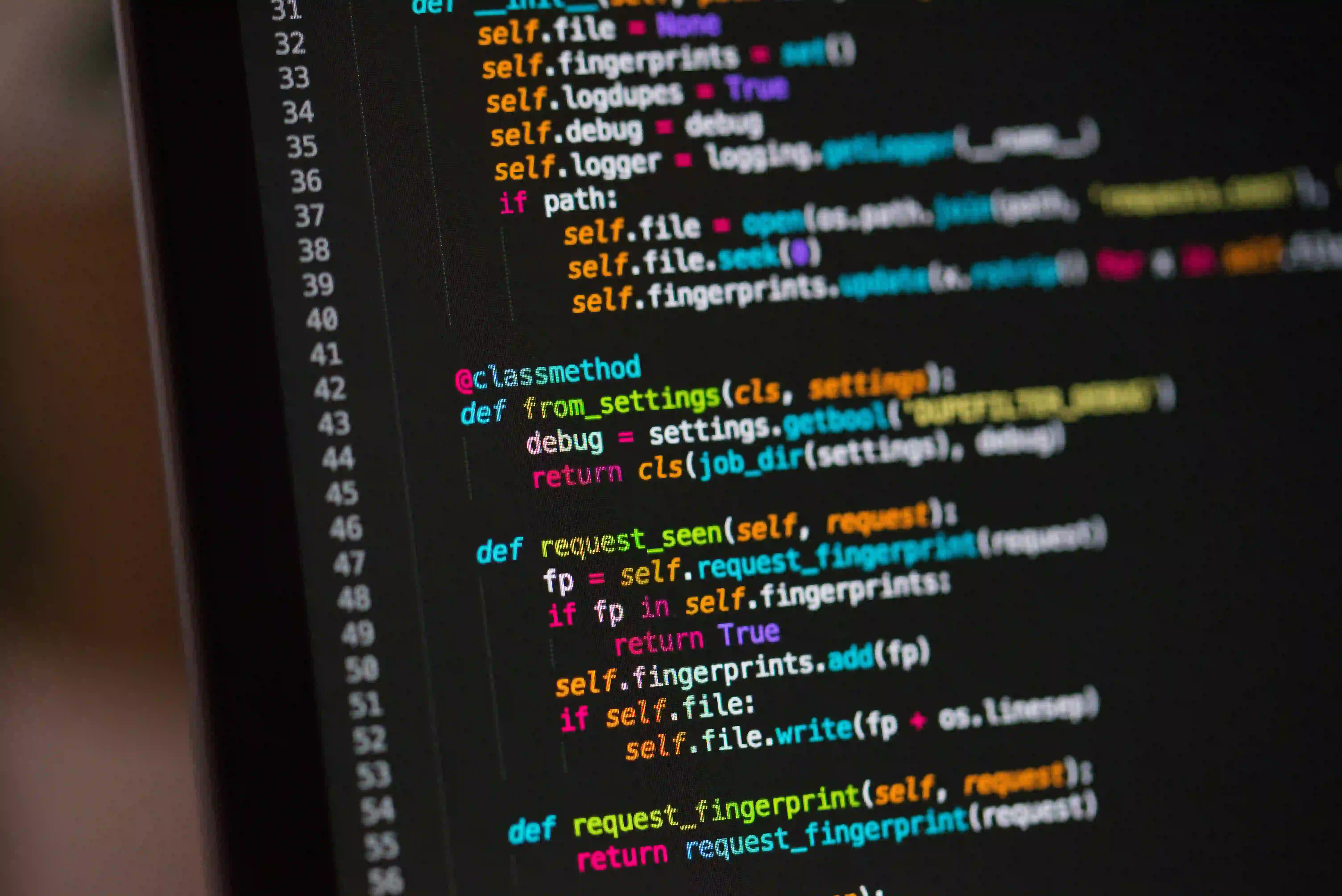
Mastering SQL Date and Timezone Queries: Your Ultimate Guide
When dealing with databases, one of the most complex data types is undoubtedly dates and times. As software applications evolve, global interactions have become more commonplace, leading to an increased need for proper timezone handling in SQL queries. In this guide, we will explore various SQL date and timezone queries, enhancing your ability to manipulate and query temporal data effectively.
Table of Contents
- Understanding SQL Date and Time Types
- Working with Timestamps
- Timezone Management in SQL
- Common SQL Date Functions
- Practical Examples
- Conclusion
Understanding SQL Date and Time Types
SQL provides various data types for storing date and time information:
- DATE: Stores dates (year, month, day).
- TIME: Stores time (hours, minutes, seconds).
- DATETIME: Combines date and time.
- TIMESTAMP: Similar to DATETIME but typically used to track time of events and can include timezone information.
- INTERVAL: Represents a duration of time.
Understanding these types is fundamental when you query for date or time-related data.
Working with Timestamps
Timestamps are useful for recording the exact moment an event occurs. In SQL, TIMESTAMP
typically includes both date and time components.
Creating a Table with TIMESTAMP
Let’s begin by creating a simple table to store event information:
CREATE TABLE events (
id SERIAL PRIMARY KEY,
event_name VARCHAR(100),
event_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
- Why use
DEFAULT CURRENT_TIMESTAMP
? This ensures that when a new record is created, it automatically records the current date and time.
Inserting Data
To add events to this table, use the following query:
INSERT INTO events (event_name) VALUES ('Sample Event');
The event_date
will automatically get set to the current timestamp.
Timezone Management in SQL
Timezone management is crucial for global applications. Different SQL databases provide unique methods for handling timezones.
Getting Timezone Information
In PostgreSQL, you can retrieve the current timezone using:
SHOW TIMEZONE;
To see a list of available timezones:
SELECT * FROM pg_timezone_names;
Setting the Timezone
You can set the timezone at the session level:
SET TIME ZONE 'America/New_York';
Converting Between Timezones
To convert TIMESTAMP
values between timezones, you can use the AT TIME ZONE
clause:
SELECT event_name, event_date AT TIME ZONE 'UTC' AS event_date_utc
FROM events;
- Why use
AT TIME ZONE
? This feature allows you to view timestamps in different time zones, which is particularly important for applications with a global user base.
Common SQL Date Functions
Certain functions come in handy while working with SQL dates and times. Here’s a list of the most common SQL date functions:
CURRENT_DATE
: Returns the current date.CURRENT_TIME
: Returns the current time.EXTRACT
: Retrieve sub-parts of a date (like year, month, etc.).
Examples of Date Functions
Extracting Year from a Date
SELECT EXTRACT(YEAR FROM event_date) AS event_year
FROM events;
- Why use
EXTRACT
? This helps in categorizing or grouping events by year, essential for reporting.
Finding Events within a Date Range
For example, to find events in the last 7 days:
SELECT * FROM events
WHERE event_date >= CURRENT_DATE - INTERVAL '7 days';
- Why use
INTERVAL
? It allows you to define a specific time period, which is flexible and easy to adjust.
Practical Examples
Let's dive into several scenarios to apply what we've learned.
Scenario 1: Insert a New Event
Imagine we want to enter a new event happening in a specific timezone.
INSERT INTO events (event_name, event_date)
VALUES ('Meeting', '2023-10-01 14:00:00'::TIMESTAMP AT TIME ZONE 'America/Los_Angeles');
- Why specify a timezone for the timestamp? This ensures we accurately capture the event's intended timing based on its locality.
Scenario 2: Convert to Local Timezone
If you want to convert an event time to the user's timezone, you can perform a query like this:
SELECT event_name, event_date AT TIME ZONE 'Asia/Tokyo' AS local_event_time
FROM events;
Scenario 3: Aggregate Events by Month
To see how many events are being held each month:
SELECT DATE_TRUNC('month', event_date) AS month, COUNT(*) AS event_count
FROM events
GROUP BY month
ORDER BY month;
- Why use
DATE_TRUNC
? This function allows you to group by month effectively, providing a clearer picture of event frequency.
The Closing Argument
Mastering SQL date and timezone queries is essential for modern database management, especially for applications with a global reach. This guide has provided a robust framework to understand how to manipulate and query date and time data effectively.
To delve deeper into the subject, check out the official documentation for:
- PostgreSQL Date/Time Types
- MySQL Date and Time Functions
- SQL Server Date and Time Data Types
With these skills under your belt, you'll be well-equipped to handle temporal data in SQL confidently and competently. Happy querying!