Choosing Between JOOQ and Hibernate: Common Pitfalls
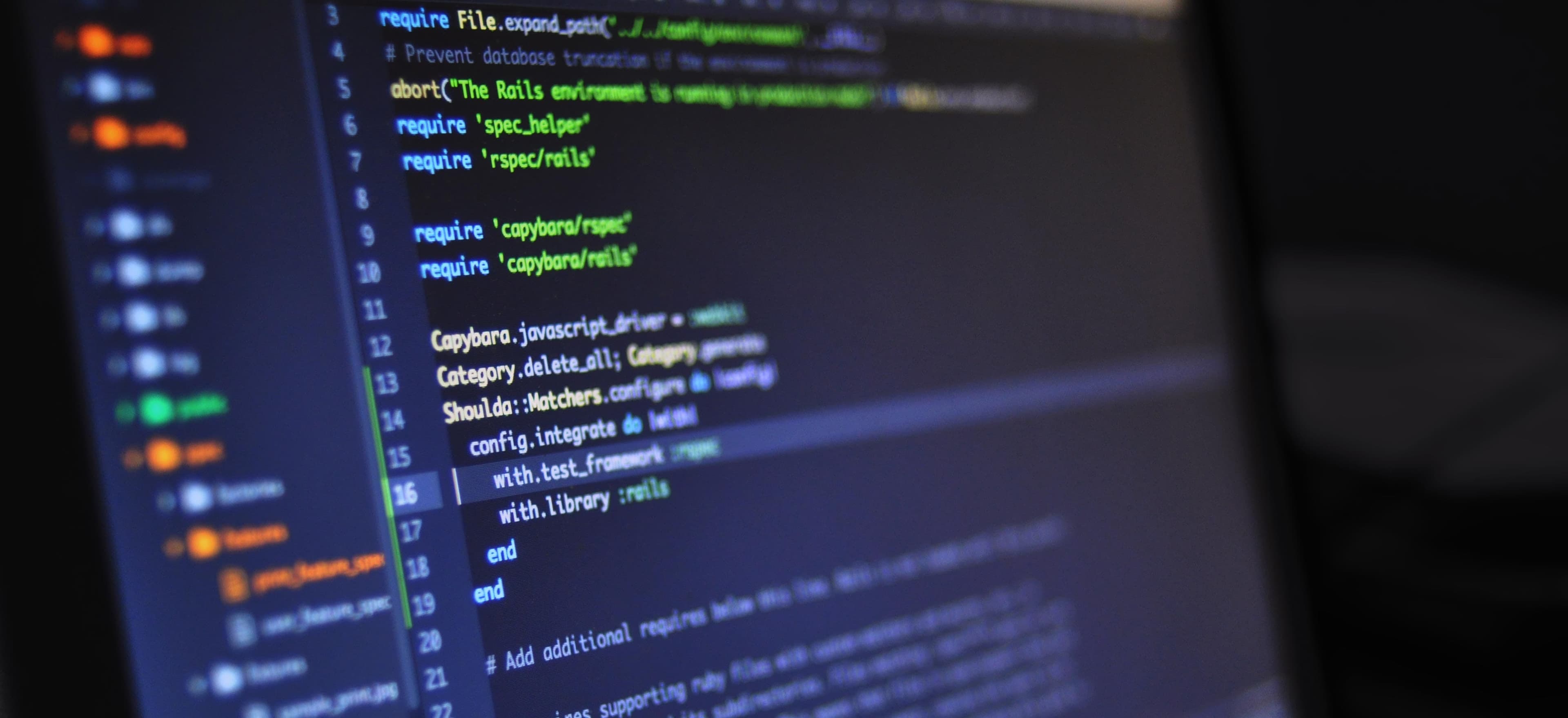
- Published on
Choosing Between JOOQ and Hibernate: Common Pitfalls
When it comes to Java ORM (Object-Relational Mapping), two popular options often emerge: JOOQ and Hibernate. Each has its strengths, but navigating the choice between them can be fraught with common pitfalls. In this blog post, we will explore these pitfalls while providing guidance on when to choose each technology for your projects.
Understanding the Fundamentals
First, let's clarify what JOOQ and Hibernate are.
JOOQ (Java Object Oriented Querying) allows developers to construct SQL queries in a type-safe manner. It works primarily with SQL databases and focuses on SQL rather than abstraction, emphasizing SQL as a first-class citizen.
Hibernate, on the other hand, is a powerful ORM framework for Java. It abstracts the database interactions away, making it easy to work with Java objects, allowing developers to focus on business logic rather than SQL ties.
Strengths of JOOQ
- SQL Focused: JOOQ empowers developers to write SQL. It's particularly valuable if you’re working with complex queries or stored procedures.
- Type Safety: With JOOQ, your SQL statements are type-checked at compile-time. This provides a safety net that can catch errors early in the development cycle.
- Flexibility: You’re not restricted to a predefined query language. Writing raw SQL queries provides flexibility in optimization and performance tuning.
Strengths of Hibernate
- Ease of Use: If you want to quickly map Java objects to database tables, Hibernate simplifies that process with automatic table generation and an identity map pattern.
- Caching: Hibernate comes with powerful caching mechanisms that can drastically improve performance for read-heavy applications.
- Integration: Hibernate seamlessly integrates with various Java frameworks and technologies, like Spring, making it an ideal choice for enterprise-level applications.
Common Pitfalls When Choosing Between JOOQ and Hibernate
1. Overlooking Use Case Scenarios
One key pitfall is not considering the specific requirements of your project. If your application is heavily SQL-dependent with complex queries, such as analytical operations or reports, JOOQ is often the better choice. Conversely, for simple CRUD (Create, Read, Update, Delete) operations, Hibernate can save time and reduce complexity.
Example:
// JOOQ example of a select query
Result<Record> result = DSL.using(configuration)
.select()
.from(TABLE)
.where(TABLE.ID.eq(1))
.fetch();
// Hibernate example of a select query
Session session = sessionFactory.openSession();
Query<MyEntity> query = session.createQuery("FROM MyEntity WHERE id = :id", MyEntity.class);
query.setParameter("id", 1);
List<MyEntity> results = query.getResultList();
In this instance, JOOQ is direct SQL, while Hibernate encapsulates it within methods, which might be overly abstracted for your needs.
2. Ignoring Performance Considerations
Performance is a fundamental factor that cannot be ignored. Hibernate’s various features, such as caching, lazy loading, and session management, can greatly enhance performance. However, if those features are not needed, they can also introduce overhead that JOOQ can circumvent.
For example, JOOQ allows for more efficient batch processing. You can run multiple queries in a single hit, thus reducing round trips to the database:
DSLContext dsl = DSL.using(configuration);
dsl.batch(
DSL.insertInto(TABLE)
.columns(TABLE.COLUMN1)
.values("value1"),
DSL.insertInto(TABLE)
.columns(TABLE.COLUMN1)
.values("value2")
).execute();
In contrast, with Hibernate, you might end up with multiple unnecessary inserts if not managed well.
3. Neglecting Learning Curve and Team Skillset
Before diving into either framework, assess your team’s familiarity with the tool. If your team has extensive experience with ORM concepts but limited SQL knowledge, Hibernate might be preferable.
However, investing in JOOQ’s learning curve might pay off if your project intensely interacts with databases and requires fine-tuned queries.
4. Misusing Abstractions
Abstraction can often lead to misunderstandings. Developers may misuse Hibernate's abstraction layers, leading to complex issues. For instance, relying too heavily on lazy loading without understanding the underlying concept can result in the “N+1 selects” problem, which can lead to performance degradation.
In contrast, JOOQ provides a straightforward approach that requires encapsulating operations into explicit queries. This transparency can be beneficial for debugging and performance optimization.
5. Not Leveraging Documentation and Community Support
Both JOOQ and Hibernate have extensive documentation and vibrant communities. Failing to utilize these resources can result in missed opportunities to optimize your use of the frameworks.
For JOOQ, the official documentation is a treasure trove of examples and best practices. Similarly, Hibernate ORM documentation provides detailed insights into its various features.
6. Underestimating the Transition Cost
Moving from one framework to another can introduce complexity. If you start with Hibernate and later switch to JOOQ (or vice versa), refactoring could be time-consuming. This includes redeveloping queries and mappings, as well as changing the way your application interacts with the database.
A Final Look
Choosing between JOOQ and Hibernate largely hinges on your specific project needs, your team's expertise, and the performance requirements. While JOOQ shines in SQL-centric scenarios offering flexibility and type safety, Hibernate excels in its ease of use and caching capabilities.
In summary, consider your use case scenarios, performance needs, team skill sets, and the treacherous path of abstraction. By keeping these pitfalls in mind, you can make an informed decision, leading your project toward success.
Additionally, if you're interested in seeing how these frameworks stack up in real-world applications, check out the following resources:
- Sorting Through The JOOQ vs. Hibernate Debate
- Harnessing Hibernate Performance Tips
Ultimately, the best choice is the one that aligns with your needs and allows your development team to excel!
Checkout our other articles