Performance Issues Caused by Spring's Lazy Annotation
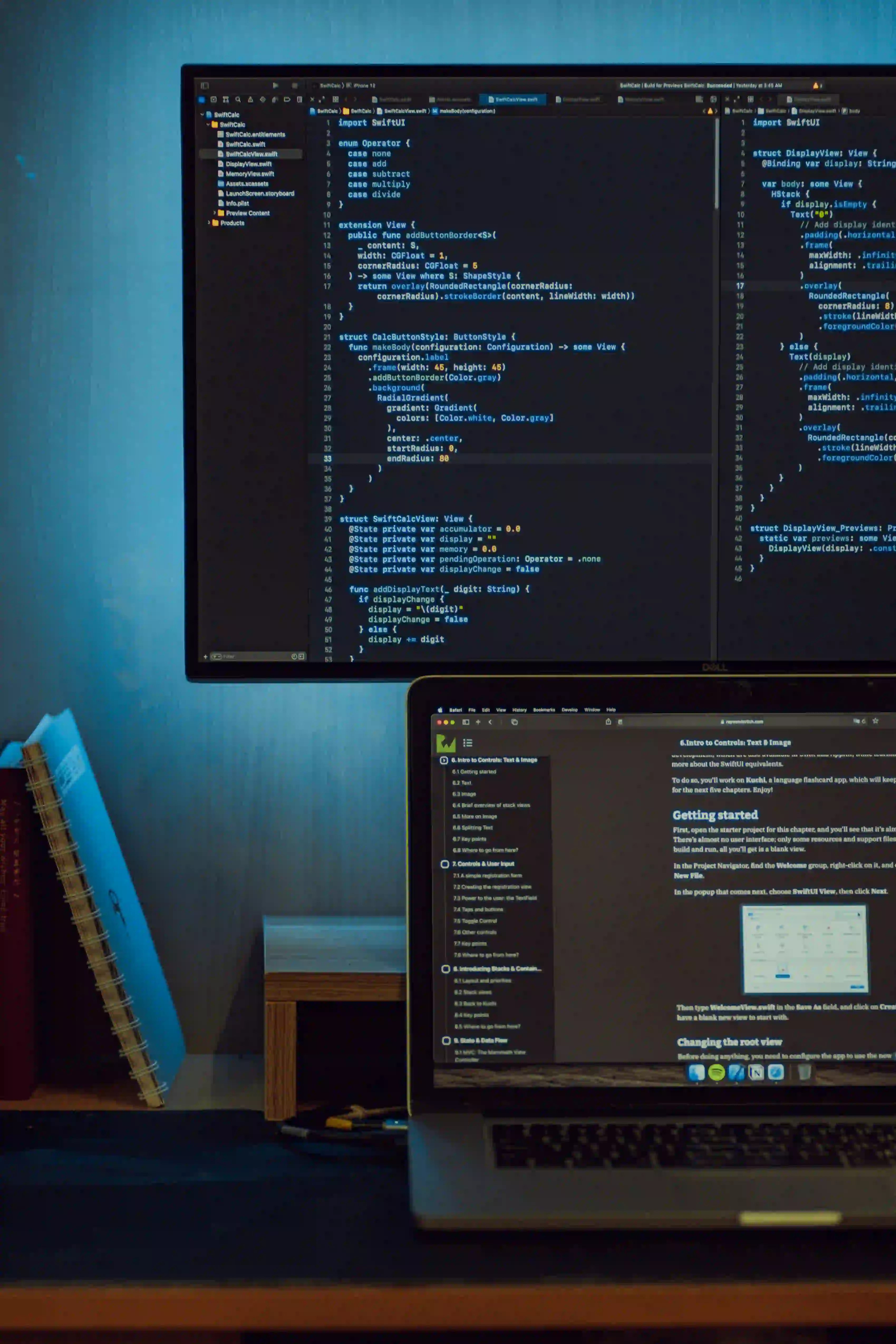
Performance Issues Caused by Spring's Lazy Annotation
In the world of software development, performance is paramount. As applications grow in complexity, developers seek ways to improve efficiency while maintaining functionality. One aspect of Spring Framework that often comes into play is the use of lazy initialization, typically achieved through the @Lazy
annotation. Although this feature can enhance performance in some scenarios, it can also lead to unexpected performance bottlenecks if not used judiciously. This blog post delves into the implications of Spring's @Lazy
annotation and examines when its use can cause more problems than it solves.
Understanding Lazy Initialization
Before discussing the potential pitfalls of the @Lazy
annotation, let's first clarify what lazy initialization means. In simple terms, lazy initialization defers the creation of an object until the moment it is needed, rather than at the start of the application. This can lead to reduced memory consumption and quicker startup times, especially when dealing with large and complex beans.
Example of the @Lazy Annotation
Here's a straightforward example of using the @Lazy
annotation in a Spring application:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
private final PaymentService paymentService;
@Autowired
public OrderService(@Lazy PaymentService paymentService) {
this.paymentService = paymentService;
// The PaymentService isn't instantiated until it's actually needed.
}
public void processOrder(Order order) {
paymentService.processPayment(order);
// More logic for processing the order
}
}
In this example, the PaymentService
is annotated with @Lazy
, meaning it will not be instantiated until processOrder
is invoked. This may seem beneficial in saving resources, but it introduces a trade-off that we must evaluate.
Potential Performance Pitfalls of @Lazy
While lazy initialization can improve startup performance, there are situations where its use can introduce performance overhead. Here are some key concerns:
1. Impact on Startup Time
Although lazy beans may start faster, initializing them on-demand can lead to slow execution times during the first call to the method that relies on them. This may not only affect user experience but also system performance under load.
public void handleRequest(Request request) {
// First call to OrderService triggers PaymentService initialization
orderService.processOrder(request.getOrder());
}
As seen above, if multiple requests hit your application simultaneously, each initial request will incur initialization overhead, affecting the perceived performance.
2. Complex Dependency Management
When multiple components depend on each other and have @Lazy
annotations, it can complicate the dependency graph. In some cases, this might lead to circular dependencies which can further degrade performance and lead to application failures.
3. Increased Latency in High Traffic Situations
If a bean is not being used frequently, the lazy-load performance might seem to offer benefits. However, during high-volume traffic, the cumulative latency introduced by the on-demand initialization may create a bottleneck, especially if it involves expensive resource allocations (e.g., database connections, HTTP requests).
Assessing Performance with @Lazy
To assert the actual performance impact of using @Lazy
, profiling your application is key. Utilize tools such as Spring Boot Actuator to monitor bean initialization times. This data can provide insights for optimizing lazy-loading usage.
Alternative Solutions
Eager Initialization
If profiling reveals that your application heavily relies on certain beans, consider not using @Lazy
and opting for the default eager initialization. Eager beans are created at startup, providing predictability in performance.
@Service
public class PaymentService {
// Eagerly loaded by Spring at startup
}
Conditional Beans
Another alternative is to conditionally load beans based on profile or environment. This can offer more control and mitigate unnecessary resource consumption.
@Profile("production")
@Service
public class ProductionPaymentService implements PaymentService {
// Only instantiated in production
}
For more information on conditional beans, refer to the Spring documentation here.
Caching
If your use case allows for it, implementing caching mechanisms can significantly enhance performance by avoiding repetitive initialization costs. Libraries like Redis and Ehcache provide efficient caching strategies that can reduce service call load.
import org.springframework.cache.annotation.Cacheable;
@Service
public class CachedOrderService {
@Cacheable("orders")
public Order fetchOrderById(String orderId) {
// Simulate a costly database fetch
return orderRepository.findById(orderId).orElse(null);
}
}
In this case, the fetchOrderById
method won’t trigger the database call if the order has already been retrieved, saving time and resources.
In Conclusion, Here is What Matters
The @Lazy
annotation is a double-edged sword in the Spring Framework. While it can help manage resource utilization and improve startup times, over-reliance on lazy initialization can lead to significant performance hits in specific scenarios. Understanding when and how to use it effectively is crucial for optimal application performance.
Before applying the @Lazy
annotation, be sure to profile your application to identify the actual impact on performance. Also, consider alternatives like eager initialization and caching strategies to strike a better balance between performance and resource utilization.
Remember, in the realm of performance optimization, knowledge and understanding are power. Choose wisely!
For more detailed insights into Spring performance best practices, check out the article on Spring Boot Performance Tuning.
By staying informed and making calculated decisions regarding bean initialization, you can build robust Spring applications that meet both performance and scalability needs.