Fixing Common Java Logging Statement Mistakes
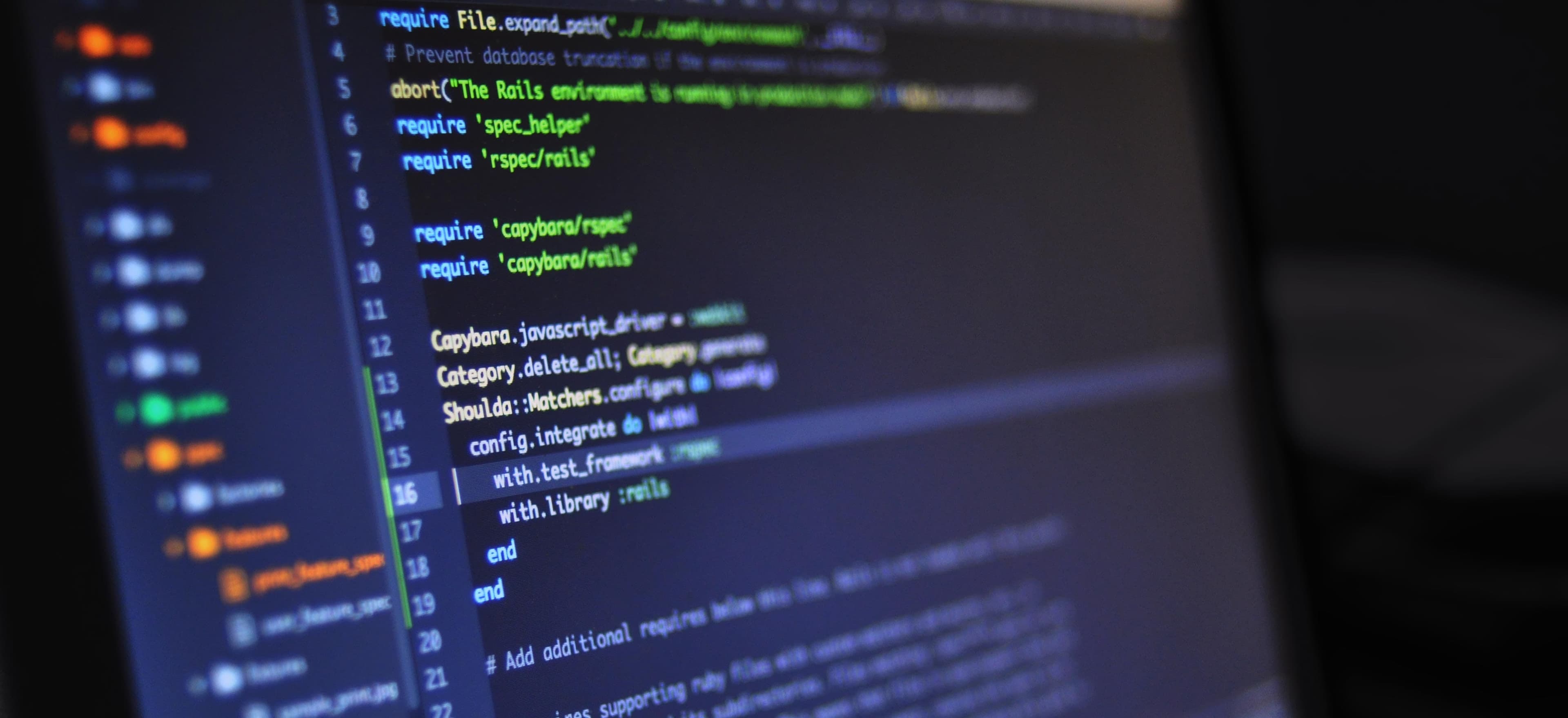
- Published on
Fixing Common Java Logging Statement Mistakes
Java logging is an essential aspect of application development. It simplifies tracking down issues, helps in monitoring application behavior, and enhances the debuggability of your code. Yet, many developers often make mistakes in their logging practices, leading to performance issues, unreadable logs, or even missing critical information.
In this blog post, we’ll explore some common Java logging statement mistakes and provide solutions to improve your logging practices. Whether you’re a beginner or an experienced Java developer, mastering logging can significantly boost the quality and maintainability of your code.
1. Ignoring log levels
One of the most frequent mistakes developers make is not using the appropriate log levels. Java provides different logging levels, such as SEVERE
, WARNING
, INFO
, CONFIG
, FINE
, FINER
, and FINEST
.
Example:
logger.info("User logged in");
logger.warning("Failed login attempt");
logger.severe("Database connection error");
Explanation:
Using the correct log level is crucial. It helps to categorize logs and allows for efficient log handling. INFO
is for general information about application flow, WARNING
indicates potential problems, and SEVERE
signifies serious failures that need immediate attention.
By not using the correct log level, you risk overwhelming your logs with unfiltered information, making it challenging to sift through when diagnosing issues. Always ask yourself: What is the significance of this log? Does it require immediate attention?
2. Logging Exceptions Improperly
Another common mistake is logging exceptions without sufficient context. Logging just the exception message misses valuable information that could help debug the issue.
Example:
try {
// some code that might throw an exception
} catch (IOException e) {
logger.severe(e.getMessage());
}
Improved Example:
try {
// some code that might throw an exception
} catch (IOException e) {
logger.log(Level.SEVERE, "Failed to read the file at path: " + filePath, e);
}
Explanation:
In the improved example, we add context to the log message. Logging the file path and the exception itself provides more information to the developer. This approach allows for a better understanding of what might have led to the exception and can significantly reduce troubleshooting time.
For further information on logging exceptions, you can visit the Java Logging documentation.
3. Over-logging
Just like under-logging, over-logging can also become a problem. Logging every single action can clutter your logs, making it harder to find the information that really matters.
Poor Logging Example:
logger.fine("Entering method doSomething");
logger.fine("Setting value to 5");
logger.fine("Value is now set to: " + value);
logger.fine("Exiting method doSomething");
Better Logging Example:
logger.fine("Entering method doSomething");
// Perform logic...
logger.fine("Exiting method doSomething");
Explanation:
In the better logging example, we’ve streamlined the messages. Instead of logging each minor step, we only log the entry and exit of the method. This practice improves readability and prevents excessive logging, which may lead to performance degradation.
4. Not Using Parameterized Logging
String concatenation in log messages is not only less efficient, but it can also lead to unnecessary object creation. Using parameterized logging avoids this issue.
Example of Concatenation:
logger.info("User " + username + " has logged in at " + timestamp);
Recommended Parameterized Logging:
logger.log(Level.INFO, "User {0} has logged in at {1}", new Object[]{username, timestamp});
Explanation:
By using parameterized logging, the Java Logging API handles message construction efficiently. The logging framework evaluates the parameters only if that specific log level is enabled. This means avoiding heavy calculations when the log is not required.
Learn more about the efficiency of logging practices in the Java Logging API.
5. Failing to Configure Logging Properly
A common issue developers face is not configuring their logging framework correctly. Whether it's a misconfigured logging level or not implementing appenders properly, this can lead to loss of crucial logs.
Example:
In a simple Java application, using the default console logger without setting a proper logging level might look like this:
logger.setLevel(Level.ALL);
Recommendation:
Always ensure your logger is correctly configured based on your environment (development, testing, production). Using external configuration files (like logging.properties
or log4j.xml
) enables maintaining varying configurations across environments easily.
Summary of a Basic Configuration:
handlers= java.util.logging.ConsoleHandler
.level= INFO
java.util.logging.ConsoleHandler.level = ALL
This configuration would allow you to see all logs on the console while keeping application logic clean and maintaining high performance.
My Closing Thoughts on the Matter
Effective logging in Java can save you from many headaches during the development process. Implementing well-structured logging practices can enhance your debugging capabilities while maintaining performance and readability. Always choose the correct log level, add context to your logs, avoid over-logging, use parameterized statements, and configure your logging framework properly.
Remember that maintaining organized and meaningful logs is an ongoing process. Keep refining your logging strategies and learn from your experiences.
Do you have any logging tips or practices that have worked for you? Share your thoughts in the comments below. Happy coding!
For further reading, check out the official Java Tutorials for comprehensive insights into logging best practices and examples.
Checkout our other articles