Common Misunderstandings About Code Refactoring
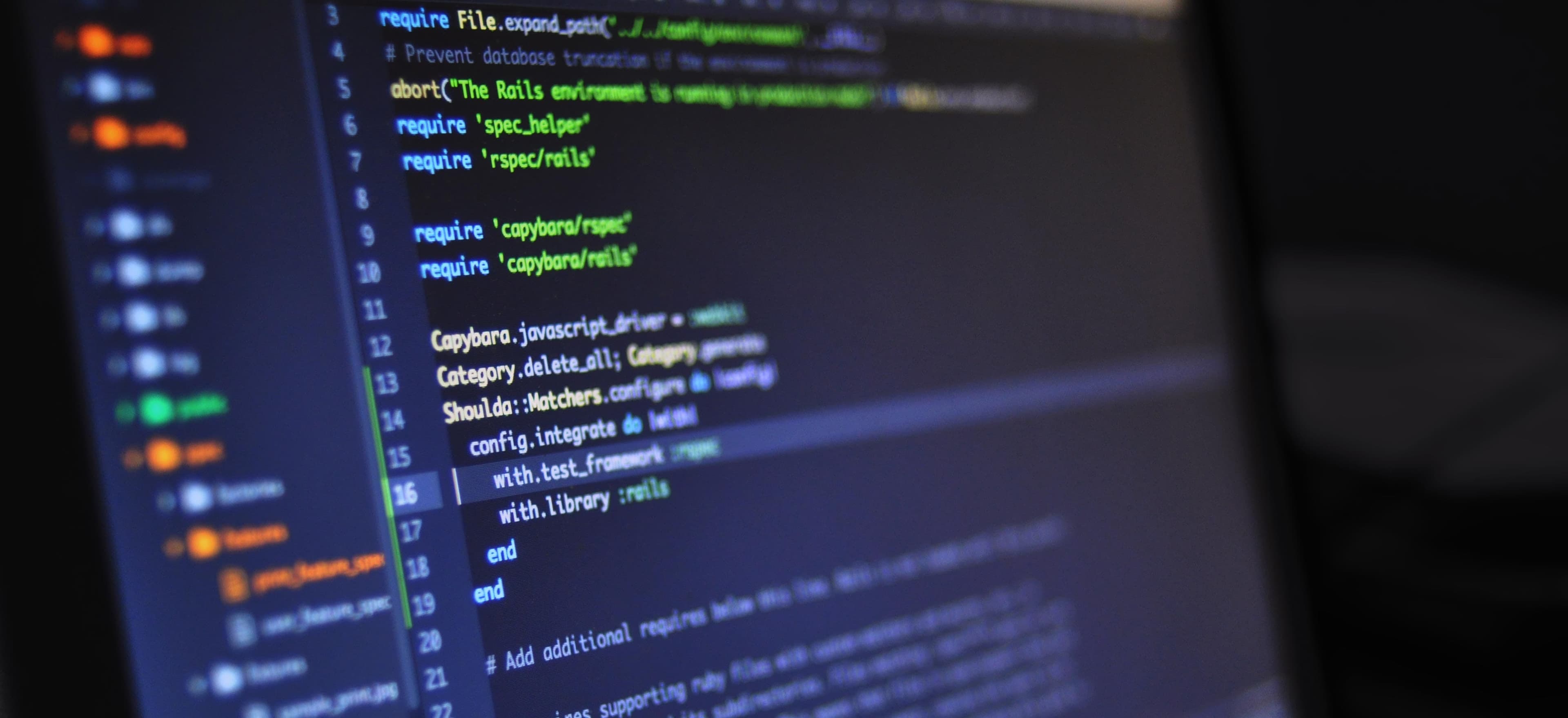
- Published on
Common Misunderstandings About Code Refactoring
Code refactoring is an integral part of software development. It allows developers to improve the structure, design, and implementation of existing code without altering its external behavior. However, despite its importance, several misunderstandings surround the process of refactoring. In this post, we will clarify these misconceptions and explore the benefits of effective code refactoring in Java.
What is Code Refactoring?
Before delving into common misunderstandings, it's essential to define what code refactoring is. Refactoring involves modifying code to enhance readability, reduce complexity, and improve maintainability while ensuring that the original functionality remains intact.
Some widely accepted reasons for code refactoring include:
- Improving code readability: Clean and clear code is easier for developers to understand.
- Reducing technical debt: Addressing poor coding practices prevents long-term issues.
- Enhancing performance: Streamlined code can boost an application's efficiency.
Misunderstanding #1: Refactoring is Only About Makeovers
One common misunderstanding is that refactoring is merely about making code look pretty. This oversimplification overlooks the real benefits of refactoring.
Reality
Refactoring is much more than cosmetic changes. It fundamentally alters the internal structure of the code. Consider the following code snippet:
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFullName() {
return firstName + " " + lastName;
}
}
While the basic functionality is clear, let's say we want to improve the code's maintainability and readability. By introducing a single getFullName()
method, we've centralized the logic for generating a user's full name, making future changes easier.
Misunderstanding #2: Refactoring is Time-Consuming
Another prevalent belief is that refactoring takes too much time, often leading to delays in project delivery. This is a fair concern, but it doesn't tell the entire story.
Reality
Investing time in refactoring can save developers more time in the long run. Code that is hard to read and maintain often results in numerous bugs and defects that are also labor-intensive to fix.
Here's a more refactored approach to our User
class:
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFullName() {
return String.format("%s %s", firstName, lastName);
}
}
By using String.format()
, we improve clarity and maintainability. The additional time spent refactoring now pays off by reducing future maintenance efforts.
Misunderstanding #3: Refactoring is Only for Failed Code
Some developers think that refactoring is only necessary when the existing code has obvious flaws or issues.
Reality
Refactoring isn't limited to broken or poorly written code. Even clean and well-structured code can benefit from refactoring as the application grows. Constant evolution in business requirements may render parts of your code redundant or overly complex.
For instance, if you have multiple classes for user management, you could refactor them into a more cohesive structure, such as:
public class UserManager {
private List<User> users;
public UserManager() {
this.users = new ArrayList<>();
}
public void addUser(User user) {
users.add(user);
}
public String getAllUserNames() {
return users.stream()
.map(User::getFullName)
.collect(Collectors.joining(", "));
}
}
This approach centralizes user management while allowing for easier future modifications, such as adding new features related to user management.
Misunderstanding #4: Refactoring is Always Beneficial
While refactoring has numerous advantages, it is not universally beneficial. Some developers think that refactoring will always result in improved performance or code quality.
Reality
Refactoring should be purposeful. If it is done without a clear goal, it can introduce new bugs or complicate the code further. The goal of refactoring should be to simplify and improve the codebase rather than just making changes for the sake of change.
Moreover, during refactoring, developers must adhere to best practices and maintain testing. Ensuring that existing tests continue to pass post-refactoring is imperative to guarantee that the application remains reliable.
Example Code with a Test Case
Consider the previous UserManager
class. Before refactoring, you should have some test cases in place:
import org.junit.Test;
import static org.junit.Assert.*;
public class UserManagerTest {
@Test
public void testAddUser() {
UserManager userManager = new UserManager();
User user = new User("John", "Doe");
userManager.addUser(user);
assertEquals("John Doe", userManager.getAllUserNames());
}
}
This ensures that even after refactoring, all necessary functionalities are intact and that you didn’t inadvertently break anything.
Misunderstanding #5: Tools Can Do All the Refactoring
Many developers assume that software tools can handle all refactoring needs, ultimately removing the need for manual oversight.
Reality
While tools can automate parts of the refactoring process, they cannot completely replace a developer's insight and judgment. Automated tools provide suggestions or perform refactorings (like changing variable names), but a human touch is often required to determine the best path forward.
Manual review is crucial to understanding the complexities of the code, improving code quality, and ensuring that the overall architecture meets the application's requirements.
Here is an example of using a tool
Using an IDE like IntelliJ IDEA, you can invoke refactoring options:
- Right-click on a method name.
- Choose "Refactor" > "Rename".
However, always review changes—both automated and manual—before finalizing them, as human errors can still arise during the process.
Final Thoughts
Refactoring is an invaluable practice in software development, particularly in Java. By dispelling common misunderstandings, developers can approach code refactoring with the right mindset, appreciation, and preparedness to tackle challenges and seize opportunities for improvement.
The importance of readable, maintainable, and efficient code cannot be overstated. To engage in successful refactoring, developers must focus on clarity and purpose, supported by relevant tests throughout the process.
For further in-depth exploration on refactoring techniques, consider reading resources such as Refactoring: Improving the Design of Existing Code by Martin Fowler. Embracing the principles of refactoring can lead not only to better code but also a more positive development experience.