Common Spring Boot Mistakes Beginners Must Avoid
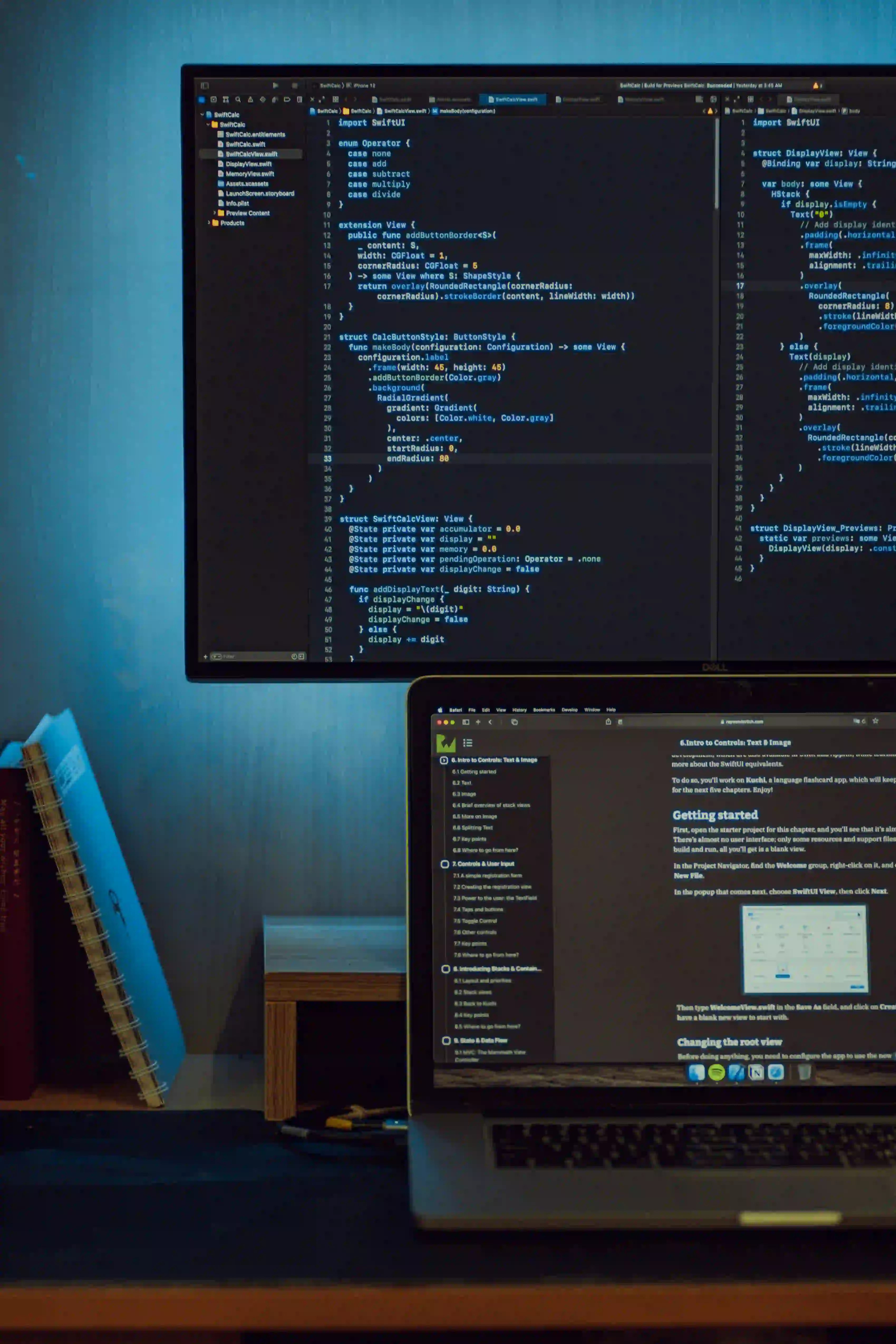
Common Spring Boot Mistakes Beginners Must Avoid
Spring Boot has revolutionized the world of Java development by providing a number of features that simplify application development. Its convention over configuration philosophy allows developers to concentrate on coding rather than configuring. However, even with its user-friendliness, beginners can still stumble upon common pitfalls. In this blog post, we will explore these mistakes, offering not just insights but also examples to help you avoid them in your projects.
1. Not Utilizing Spring Boot Features Fully
Explanation
One of the unique selling propositions of Spring Boot is its extensive feature set, including auto-configuration, embedded servers, and Spring's powerful dependency injection. Many beginners, however, stick to plain Spring features without leveraging the extended functionalities.
Solution
Explore the capabilities Spring Boot offers and integrate them into your development process. For instance, instead of configuring an application server separately, use the built-in Tomcat or Jetty servers.
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
By simply adding the @SpringBootApplication
annotation, your application can run standalone on an embedded server. There’s no need for deploying it to a separate server, which greatly reduces setup time and complexity.
2. Ignoring the Power of Profiles
Explanation
Spring Boot allows for the use of profiles to manage different configurations for different environments. Beginners sometimes fail to leverage this feature, creating a single configuration for all environments.
Solution
Use application-{profile}.yml
or application-{profile}.properties
files to separate your configurations.
# application-dev.yml
server:
port: 8081
# application-prod.yml
server:
port: 80
In this way, when you run your application in development mode (spring.profiles.active=dev
), it will use the necessary configurations suitable for a development environment. This ensures that your production settings remain secure and stable.
3. Failing to Properly Manage Dependencies
Explanation
Spring Boot simplifies dependency management through its Spring Boot Starter dependencies. Beginners may not fully understand the importance of using these starters and might manually add dependencies for each library.
Solution
Use Spring Boot Starters. For instance, for a web application, you can include spring-boot-starter-web
in your build file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
By doing this, you automatically get the necessary dependencies like Spring MVC, Tomcat, and Jackson for JSON processing. This will not only save you time but also minimize versioning issues.
4. Misconfiguring Data Sources
Explanation
Connecting to databases is a common task in Spring Boot applications. Beginners often face issues when misconfiguring data sources, leading to connection failures or performance bottlenecks.
Solution
Make sure to configure your data sources properly. Opt for a connection pool like HikariCP, which is the default for Spring Boot.
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: user
password: password
hikari:
maximum-pool-size: 10
Make use of the hikari
section to specify connection pool settings. Proper management of database connections can significantly improve your application's performance.
5. Overlooking Testing
Explanation
Testing is often an afterthought for many beginners. They might create an application and skip writing tests until the very end. This is a common mistake that can lead to significant technical debt down the line.
Solution
Incorporate testing from the start. Use the built-in testing capabilities that Spring Boot offers, including testing annotations such as @SpringBootTest
.
@SpringBootTest
public class MyApplicationTests {
@Test
public void contextLoads() {
}
}
This simple test ensures that the Spring application context loads successfully. Integrating unit tests and integration tests from the beginning will make your application more robust and easier to maintain.
6. Not Securing Applications
Explanation
Security is critical in modern applications. Beginners sometimes neglect the security configurations that Spring Security provides, exposing their applications to vulnerabilities.
Solution
Use Spring Security’s built-in mechanisms to secure your application right from the start.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
This configuration secures all endpoints and enables form-based login. Ensure you implement security measures early in your development process to protect sensitive data and user credentials.
7. Missing Out on Actuator Endpoints
Explanation
Spring Boot's Actuator module is an invaluable asset for monitoring and managing applications. However, beginners often overlook it due to a lack of awareness.
Solution
Add the Actuator dependency to your project and configure it:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
You can access various endpoints, such as health checks and metrics, which can provide a wealth of information about your application's runtime behavior.
8. Skipping Documentation and Comments
Explanation
Understanding your code becomes increasingly difficult without proper documentation and comments. Beginners might neglect this aspect, leading to unmaintainable code.
Solution
Document your code adequately. Use meaningful comments to explain complex logic, and consider employing Javadoc to provide context about your classes and methods.
/**
* Service class to handle user operations.
*/
@Service
public class UserService {
/**
* Registers a new user.
*
* @param user The user to be registered.
* @return The registered user.
*/
public User register(User user) {
// Logic to register the user
}
}
Not only will this practice make your code more maintainable, but it will also aid your future self—and others—when revisiting codebases.
Key Takeaways
Spring Boot is a fantastic framework for Java developers, but like any powerful tool, it comes with its own set of complexities and nuances. By avoiding the common mistakes outlined in this post, beginners can greatly improve the quality, maintainability, and security of their applications. Always remember to leverage the built-in features of Spring Boot, secure your applications, and maintain proper documentation to set a strong foundation for your projects.
For further reading, you may find the following resources helpful:
By addressing these common pitfalls, new developers can focus on building innovative applications and enjoying the process of programming. Happy coding!