Solving Cache Invalidation Issues in Spring Boot with Hazelcast
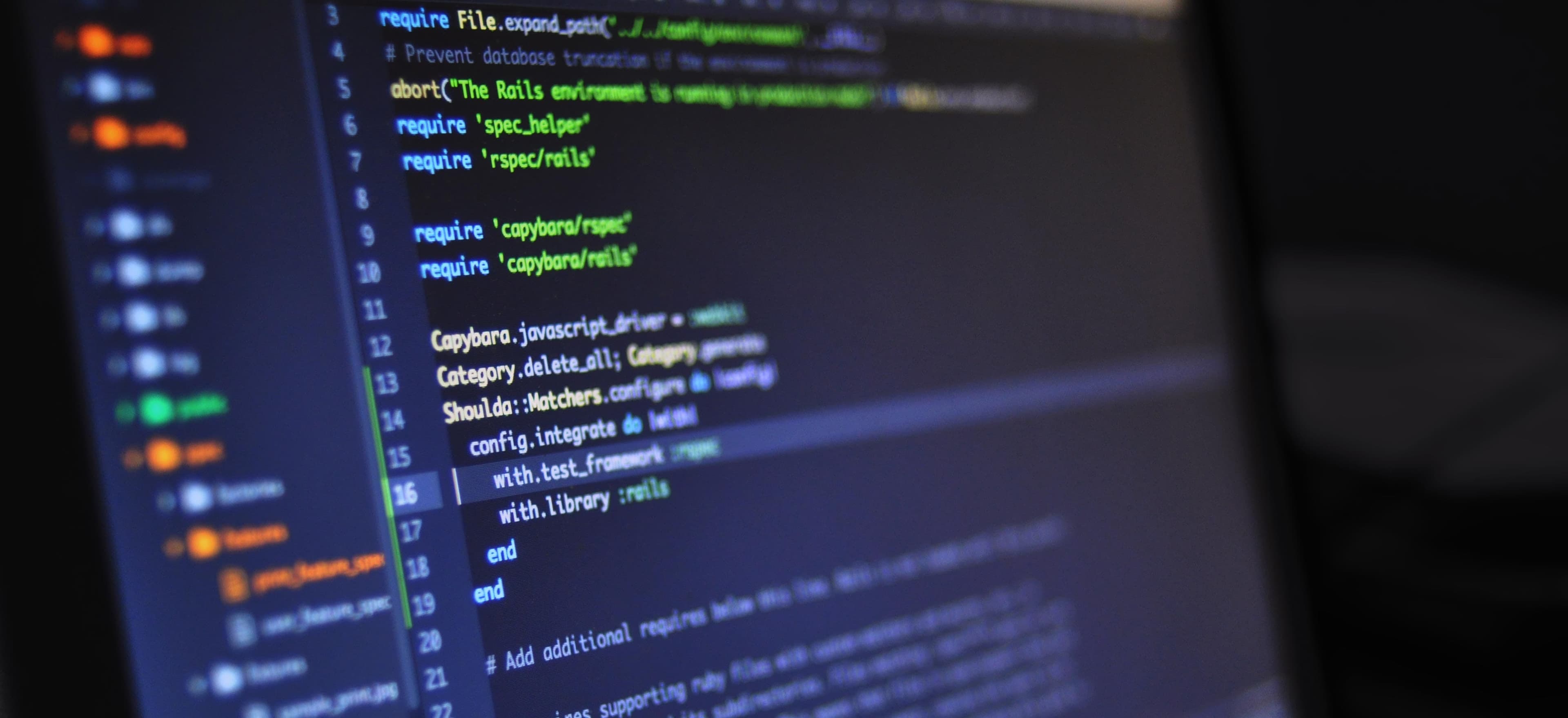
- Published on
Solving Cache Invalidation Issues in Spring Boot with Hazelcast
In modern applications, caching plays a pivotal role in enhancing performance. However, implementing an effective caching strategy raises some challenges—most notably, cache invalidation. In this blog post, we will delve into cache invalidation issues when using Spring Boot with Hazelcast, outlining strategies to overcome them.
What is Cache Invalidation?
Cache invalidation is the process of removing or updating cache entries when the underlying data changes. It's a critical concept because the accuracy of the application depends heavily on the freshness of the cached data. Failing to manage cache invalidation can lead to stale data being served, which ultimately leads to poor user experience and potential business losses.
Why Choose Hazelcast?
Hazelcast is an in-memory data grid that provides distributed caching capabilities. It offers features such as scalability, persistence, and easy integration with Spring Boot, making it an excellent choice for enterprise-level applications.
In this blog, we'll walk through common cache invalidation issues in Spring Boot applications using Hazelcast and present strategies to resolve them.
Setting Up Spring Boot with Hazelcast
First, let’s set up a simple Spring Boot application that integrates with Hazelcast.
Maven Dependency
Add the following dependencies to your pom.xml
for Hazelcast and Spring Cache:
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast-spring</artifactId>
<version>5.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
Application Properties
Next, configure Hazelcast in the application.properties
file:
spring.cache.type=hazelcast
hazelcast.config=classpath:hazelcast.xml
Hazelcast Configuration
Create a hazelcast.xml
configuration file at the root of your src/main/resources
directory:
<hazelcast>
<network>
<join>
<multicast enabled="false"/>
<tcp-ip enabled="true">
<member>127.0.0.1:5701</member>
</tcp-ip>
</join>
</network>
</hazelcast>
This configuration makes Hazelcast listen on 127.0.0.1:5701
.
Sample Service
Here, we will create a simple service to demonstrate caching.
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
simulateSlowService(); // Simulate a slow service.
return findUserById(id);
}
private User findUserById(Long id) {
// Fetch user from the database.
}
private void simulateSlowService() {
try {
Thread.sleep(3000); // Simulate delay
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
}
}
This UserService
class caches user data for future requests. However, how do we ensure that the cache remains consistent with the database?
Cache Invalidation Strategies
1. Using @CacheEvict
In many applications, data changes are common. When user data is updated, the relevant cache entry must be removed to prevent stale reads. Spring Boot provides the @CacheEvict
annotation to facilitate this process.
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.stereotype.Service;
@Service
public class UserService {
// Other methods...
@CacheEvict(value = "users", key = "#user.id")
public void updateUser(User user) {
// Update user in the database.
}
}
In this example, when an update occurs in updateUser
, the associated cache entry for that user is evicted. This guarantees that the next time getUserById
is called, the service will fetch the fresh data.
2. Cache Synchronization for Distributed Environments
In a distributed system, cache entries may be invalidated from different application instances. In such cases, synchronizing cache invalidation across nodes is crucial. Hazelcast supports distributed events, which can help synchronize cache entries.
import com.hazelcast.map.IMap;
import com.hazelcast.monitor.LocalMapStats;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
@Component
public class UserCacheEventListener implements ApplicationListener<UserUpdatedEvent> {
@Autowired
private HazelcastInstance hazelcastInstance;
@Override
public void onApplicationEvent(UserUpdatedEvent event) {
IMap<Long, User> userMap = hazelcastInstance.getMap("users");
userMap.delete(event.getUserId());
}
}
In this code snippet, when a UserUpdatedEvent
is published, it triggers the deletion of the relevant user entry from all cache nodes. This ensures that all instances reference the latest data.
3. Expiry Policies
Implementing an expiry policy can help alleviate some pressure from cache invalidation. Setting an expiry time for cached entries allows the system to automatically refresh entries after a specified duration.
<map name="users">
<backup-count>1</backup-count>
<time-to-live-seconds>60</time-to-live-seconds> <!-- Cache entry expires after 60 seconds -->
</map>
Setting the time-to-live-seconds
in your hazelcast.xml
ensures that all cached users are refreshed every 60 seconds.
4. Manual Cache Management
In complex scenarios, you may require more control over your caching strategy. In such cases, you might manage the lifecycle of cache entries manually:
import org.springframework.cache.CacheManager;
import org.springframework.beans.factory.annotation.Autowired;
@Autowired
private CacheManager cacheManager;
public void customCacheEvict(Long userId) {
cacheManager.getCache("users").evict(userId);
}
This manual cache management gives you the flexibility to evict, refresh, or add new entries based on your application requirements.
Wrapping Up
Cache invalidation presents challenges, but utilizing a robust caching solution like Hazelcast with Spring Boot can make it seamlessly manageable. By leveraging strategies such as @CacheEvict
, cache synchronization, expiry policies, and manual cache management, you can ensure data consistency across your application while enjoying the performance benefits of caching.
For further reading on caching strategies, you can check out the official Spring Cache documentation and Hazelcast documentation.
By implementing these strategies, you will build not only a performant application but a reliable and user-friendly experience. Happy coding!