Overcoming Challenges in Continuous Testing for CI/CD Success
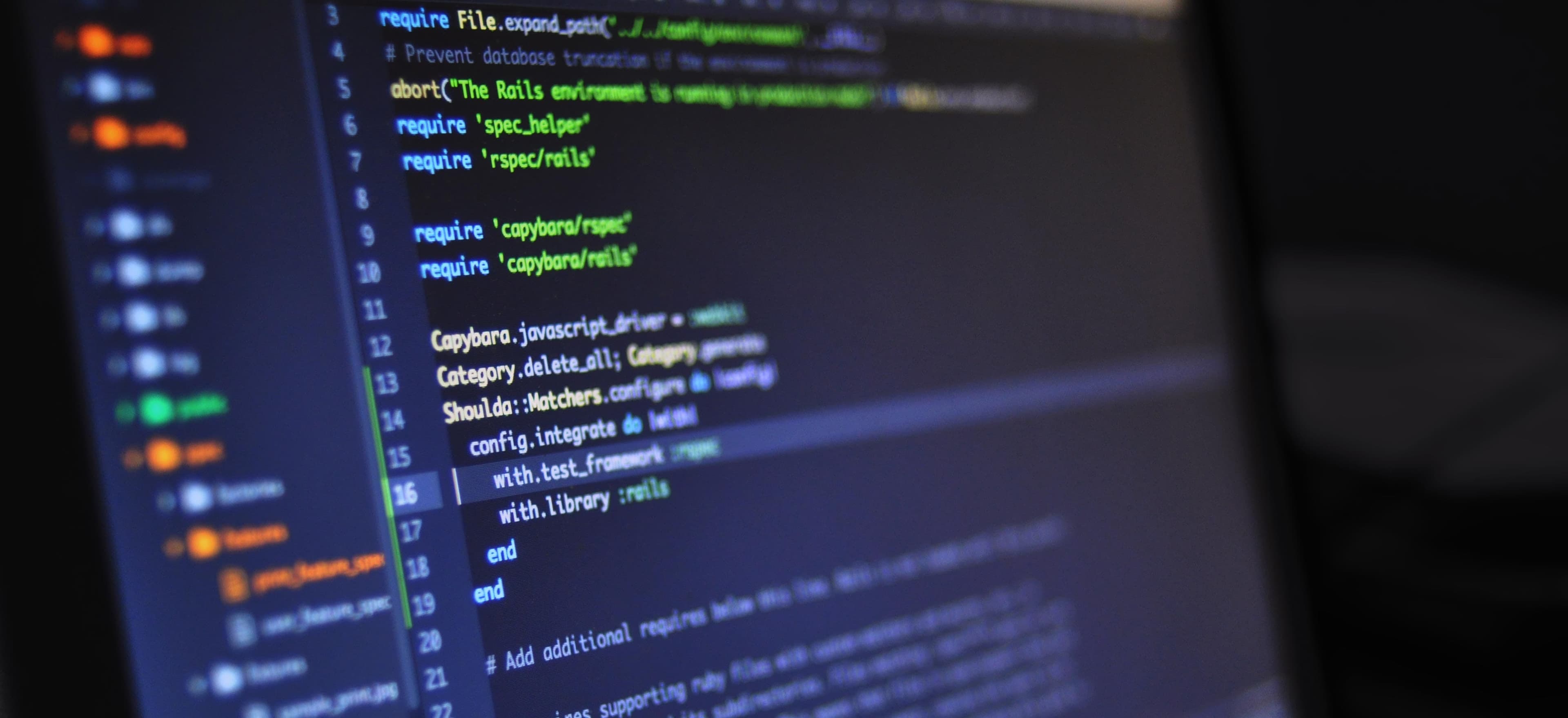
- Published on
Overcoming Challenges in Continuous Testing for CI/CD Success
In the fast-paced world of software development, Continuous Integration and Continuous Delivery (CI/CD) has emerged as a standard practice. It promotes the frequent integration of code changes, ensuring quick delivery and reliable releases. However, achieving CI/CD success is not straightforward, especially when it comes to continuous testing.
In this post, we will explore the common challenges associated with continuous testing in CI/CD environments, along with strategies to overcome them. We will also look at some examples in Java to give you a hands-on understanding of how to implement effective testing strategies.
Understanding Continuous Testing
Before we dive into the challenges, let's briefly define Continuous Testing. It refers to the automated process of running tests as part of the software delivery pipeline. This occurs continuously, rather than only at the end of a development cycle. The main goal is to get immediate feedback on the quality of the software, allowing developers to make informed decisions quickly.
Key Challenges in Continuous Testing
1. Test Automation Complexity
One of the most significant hurdles in continuous testing is the complexity of automation. Automated tests can be painstaking to write, maintain, and scale. Furthermore, the plethora of testing tools can create confusion regarding which ones to adopt.
Solution:
Prioritize and standardize your testing tools. This helps streamline the process and reduces the overhead of managing numerous tools. The Java ecosystem has robust options, such as JUnit for unit testing and Selenium for functional testing.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals("Addition should return correct sum", 5, calculator.add(2, 3));
}
}
In the code snippet above, we showcase a simple unit test using JUnit. It ensures that the addition functionality of a calculator works correctly. Utilizing a testing framework like JUnit helps reduce complexity, as it simplifies the structure of your tests.
2. Inadequate Test Coverage
Another barrier is achieving adequate test coverage. Without comprehensive tests, teams may release software that contains bugs, leading to a poor end-user experience.
Solution:
Develop a robust testing strategy that includes various test types:
- Unit Tests: These tests validate individual components.
- Integration Tests: They assess the interaction between modules.
- End-to-End Tests: These tests simulate real-world user scenarios.
Consider using code coverage tools like JaCoCo for Java projects. This tool provides insights into which parts of your code are and aren't tested, helping you focus your efforts where they are most needed.
# Command to run JaCoCo with Maven
mvn clean test jacoco:report
By integrating JaCoCo in your CI/CD pipeline, you will make informed decisions about which tests to write and where to improve your test coverage.
3. Environment Issues
Another common complication arises from testing in different environments. The installation of dependencies and the configuration of environment settings can introduce inconsistencies in the test outcomes.
Solution:
Employ containerization tools like Docker. By creating a standardized testing environment, you eliminate the "it works on my machine" syndrome.
Here’s a simple example of a Dockerfile that sets up a Java testing environment:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/my-app.jar .
CMD ["java", "-jar", "my-app.jar"]
This Dockerfile creates a lightweight Java environment that can run your application’s tests consistently across all stages of the CI/CD pipeline.
For more insights on using Docker for CI/CD, check out Docker’s official documentation.
4. Feedback Loops
In CI/CD, the feedback loop is critical. Slow or unreliable feedback mechanisms can delay the identification of defects, undermining the rapid deployment ethos of CI/CD.
Solution:
Integrate reporting tools that provide real-time feedback on testing outcomes. Implement chat integrations like Slack to notify teams when tests succeed or fail.
Example of a simple notification process in Java:
public void notifyTeam(String message) {
// Here, we would normally connect to a messaging service.
System.out.println("Team Notification: " + message);
}
This code can be integrated into your tests to alert the team should an important test fail, ensuring timely responses to critical issues.
5. Skill Gaps
Finally, a lack of skills in automated testing can be a barrier to success. A team untrained in modern practices may struggle to reap the benefits of CI/CD.
Solution:
Invest in training and development. Encourage team members to learn testing tools and methodologies through workshops or online courses. A better-educated team will lead to better testing practices and higher software quality.
You can check out platforms like Udemy or Coursera for online courses focusing on testing methodologies and tools.
Key Takeaways
In conclusion, continuous testing plays a pivotal role in the success of CI/CD efforts. By systematically addressing challenges such as test automation complexity, inadequate test coverage, environment issues, feedback loops, and skill gaps, teams can ensure that their software is reliable, high-quality, and ready for deployment.
Embrace the principles outlined in this blog post, and remember that investing time and effort into developing effective continuous testing practices will ultimately streamline your development workflow.
For those looking to dive deeper, consider reading our articles on CI/CD Best Practices or Modern Testing Strategies. Happy coding!
Checkout our other articles