Mastering JavaFX 20: Common Pitfalls to Avoid
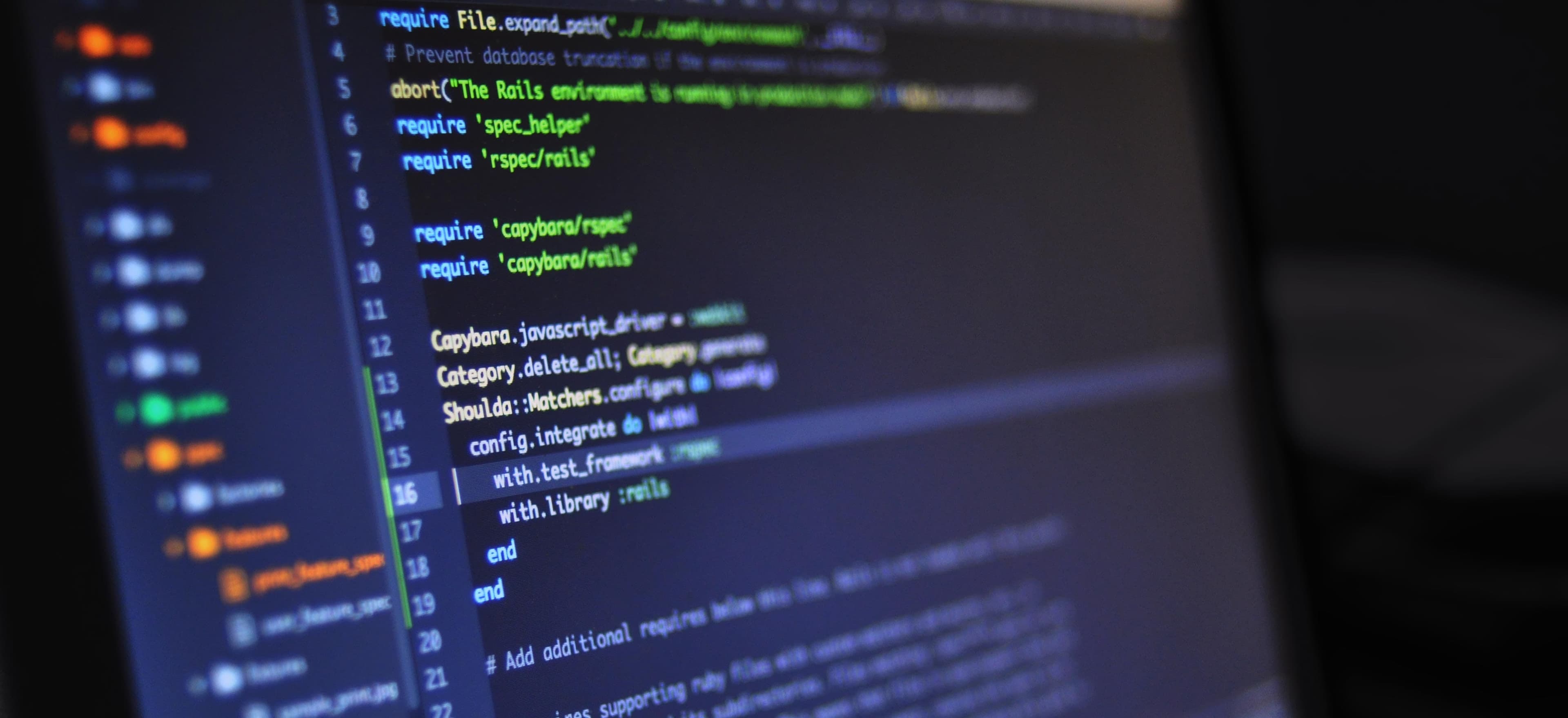
- Published on
Mastering JavaFX 20: Common Pitfalls to Avoid
JavaFX is a powerful framework for building desktop applications in Java. With the release of JavaFX 20, developers have access to new features and improvements that enhance their productivity and elevate user experience. However, as with any technology, pitfalls can arise. In this blog post, we'll explore common pitfalls when using JavaFX 20 and how to avoid them.
Table of Contents
- Understanding JavaFX 20
- Pitfall #1: Ignoring JavaFX's Threading Model
- Pitfall #2: Neglecting Scene Graph Performance
- Pitfall #3: Poor Resource Management
- Pitfall #4: Not Utilizing CSS for Styling
- Pitfall #5: Overcomplicating FXML
- Best Practices in JavaFX Development
- Conclusion
Understanding JavaFX 20
JavaFX is built to provide a rich user experience by supporting a range of features such as UI controls, 2D and 3D graphics, and multimedia. With each version, JavaFX continues to evolve, providing developers with more tools to create stunning applications. Understanding the updates in JavaFX 20 is crucial for effective application development. You can read about new features in the official JavaFX documentation.
Example: Basic Setup
Before diving into pitfalls, it’s important to ensure your JavaFX application is set up correctly. Below is a simple example of a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class HelloFX extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Say 'Hello World'");
btn.setOnAction(event -> System.out.println("Hello World!"));
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Hello JavaFX 20");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This simple application creates a window with a button that, when clicked, prints "Hello World!" to the console.
Pitfall #1: Ignoring JavaFX's Threading Model
One of the most critical aspects of JavaFX development is its threading model. JavaFX requires that all UI updates happen on the JavaFX Application Thread. Attempting to update the UI from a background thread can lead to unpredictable behavior and crashes.
Solution
To ensure safe UI updates, use the Platform.runLater
method:
Platform.runLater(() -> {
// Update UI components here
});
This sends the code inside the runLater
method to be executed on the JavaFX Application Thread.
Pitfall #2: Neglecting Scene Graph Performance
The scene graph in JavaFX is a hierarchical structure that represents the UI. A common pitfall is overloading the scene graph with too many nodes, which can lead to performance issues.
Solution
Minimize the number of nodes by grouping them into containers. Utilize simple shapes whenever possible for better performance. Here’s a best practice example:
Group group = new Group();
Ellipse ellipse = new Ellipse(50, 50, 100, 70);
group.getChildren().add(ellipse);
This example illustrates how to use a Group
to optimize rendering.
Pitfall #3: Poor Resource Management
Resources such as images and stylesheets need careful handling. Loading them multiple times or failing to release them can negatively impact your application's performance.
Solution
Load resources once and reuse them. For example:
Image image = new Image(getClass().getResourceAsStream("image.png"));
ImageView imageView = new ImageView(image);
Here, the image is loaded just once and can be displayed multiple times.
Pitfall #4: Not Utilizing CSS for Styling
JavaFX supports CSS for styling components, yet many developers rely solely on code for UI design. This approach can lead to cluttered code and difficult maintenance.
Solution
Use CSS to separate style from logic. For example, create a stylesheet:
.button {
-fx-background-color: blue;
-fx-text-fill: white;
}
Then, apply the stylesheet to your JavaFX application:
scene.getStylesheets().add("styles.css");
Utilizing CSS not only simplifies your Java code but also improves maintainability.
Pitfall #5: Overcomplicating FXML
FXML provides an easy way to build UIs, but overcomplicating it with unnecessary controls and layouts can lead to confusion and instability.
Solution
Keep your FXML files simple. Here’s an example of a clean FXML structure:
<AnchorPane xmlns="http://javafx.com/javafx" xmlns:fx="http://javafx.com/fxml">
<Button text="Click Me!" onAction="#handleButtonClick"/>
</AnchorPane>
This structure is straightforward and functional. You can define the controller method in your Java class like so:
@FXML
private void handleButtonClick() {
System.out.println("Button Clicked!");
}
Best Practices in JavaFX Development
- Keep UI updates on the JavaFX thread. Always use
Platform.runLater
for UI changes triggered from other threads. - Simplify the scene graph. Aim to use container classes like
VBox
,HBox
, andGridPane
. - Prefer CSS over inline styles. Maintain your style separately to keep your code organized.
- Utilize FXML effectively, but avoid overcomplication.
- Optimize resource loading. Ensure images and fonts are loaded efficiently.
Key Takeaways
JavaFX offers a rich platform for building modern applications. With JavaFX 20 comes the opportunity to take advantage of new features while avoiding common pitfalls. By understanding threading models, managing resources, leveraging CSS, and simplifying FXML, you can create maintainable and efficient applications.
To learn more about advanced JavaFX concepts, consider exploring the JavaFX Tutorial. Keep pushing the boundaries of your JavaFX applications, and remember that learning from these pitfalls will only make you a better developer. Happy coding!