Common Pitfalls When Using ActiveMQ in Production
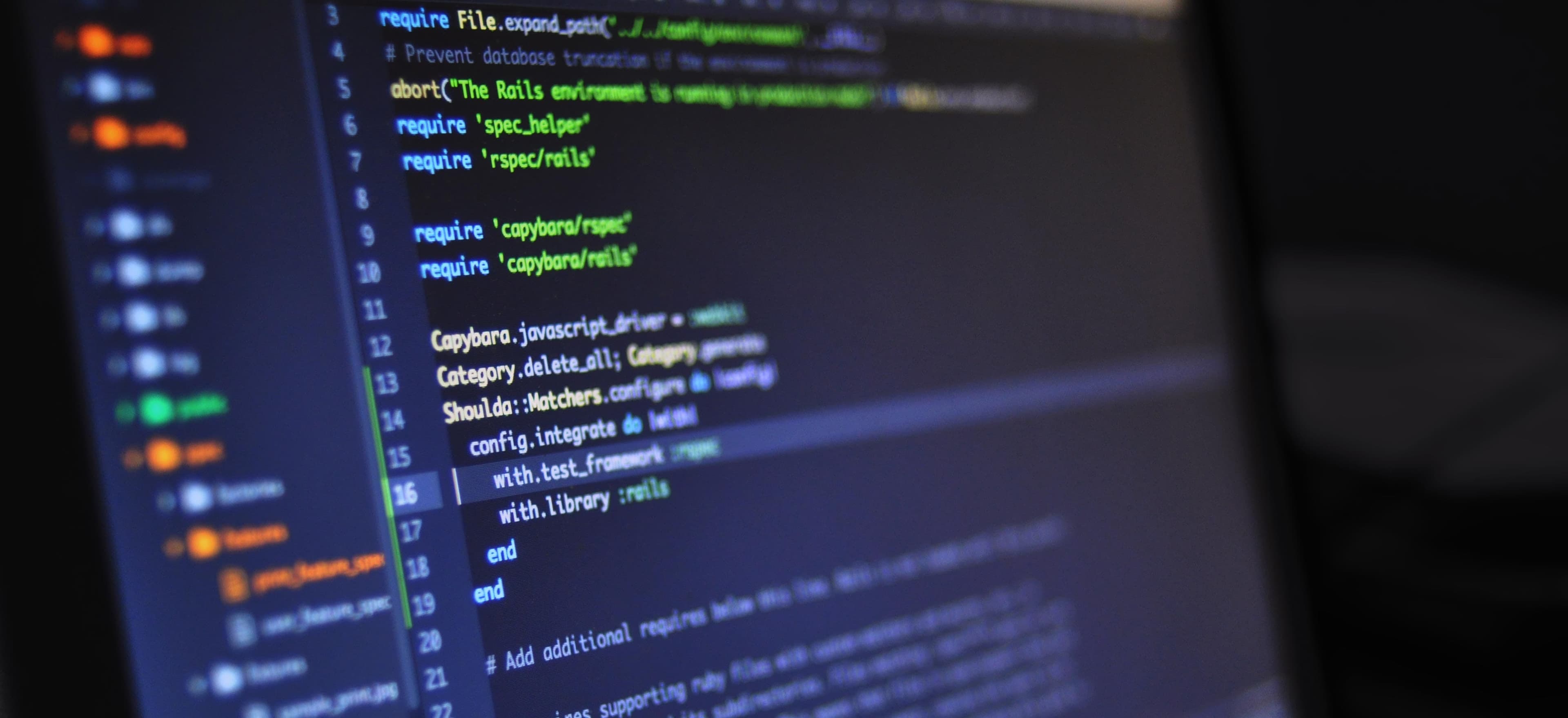
- Published on
Common Pitfalls When Using ActiveMQ in Production
ActiveMQ is a popular open-source message broker that facilitates communication between different components of a distributed application. While ActiveMQ offers robustness and scalability, several common pitfalls can arise when deploying it in production. This blog will discuss these pitfalls in detail, providing best practices and code snippets to illustrate key points.
Understanding ActiveMQ
ActiveMQ serves as a powerful messaging system that enables different systems to communicate asynchronously and reliably. This decoupling of services can lead to improved performance and fault tolerance. However, its effectiveness in production relies heavily on proper configuration and management.
Why Proper Configuration Matters
Misconfiguration can lead to performance bottlenecks, message loss, or even system outages. The choice of broker, message protocols, and persistence options can all influence the effectiveness of ActiveMQ.
Common Pitfalls
Now let's discuss some of the most common pitfalls you might encounter when using ActiveMQ in a production environment:
1. Inadequate Resource Allocation
One of the most frequent mistakes in ActiveMQ deployments is underestimating the resource requirements.
Why It Matters
ActiveMQ, like any other server, needs sufficient CPU, memory, and disk I/O to perform well. Inadequate resources can lead to issues like slowing down or crashing of the broker.
Best Practices
- Monitor resource usage regularly. Use tools like JMX or the ActiveMQ Web Console to keep tabs on memory and CPU metrics.
- Plan for scalability. Ensure you allocate additional resources based on expected growth.
public class Main {
public static void main(String[] args) {
System.out.println("Monitoring ActiveMQ Resources...");
// Use JMX to monitor CPU and memory
}
}
2. Improper Message Persistence
ActiveMQ supports different types of persistence mechanisms.
Why It Matters
Choosing the wrong persistence strategy can lead to message loss during system failures or unexpected shutdowns.
Best Practices
- Use a durable subscription for reliable message delivery.
- If you do not need messages to persist beyond a failure, consider transient messages to avoid unnecessary resource drain.
<destinationPolicy>
<policyEntry queue=">" producerPolicy="Allow" >
<pendingQueuePolicy>
<vmQueuePolicy/>
</pendingQueuePolicy>
</policyEntry>
</destinationPolicy>
3. Connection Leak
Connection leaks can happen when your application fails to close connections properly.
Why It Matters
Leaked connections can quickly exhaust the broker's available connections, leading to service degradation or outages.
Best Practices
- Always use a connection pool to manage connections.
- Ensure your code handles exceptions properly with a
finally
block to close connections.
Connection connection = null;
try {
// Create a connection
connection = connectionFactory.createConnection();
connection.start();
// Process messages...
} catch (JMSException e) {
e.printStackTrace();
} finally {
if (connection != null) {
try {
connection.close(); // Ensure the connection is closed.
} catch (JMSException e) {
e.printStackTrace();
}
}
}
4. Lack of Monitoring and Metrics
Failing to monitor your ActiveMQ instance can lead to missed warnings before significant failures occur.
Why It Matters
You must constantly keep track of metrics such as queue depth, message processing time, and error rates to maintain system health.
Best Practices
- Use tools like Grafana and Prometheus to monitor metrics in real-time.
- Consider enabling alerting on specific metrics to stay informed about potential issues.
# Example command to enable JMX monitoring
JAVA_OPTS="$JAVA_OPTS -Dcom.sun.management.jmxremote -Dcom.sun.management.jmxremote.port=9999 -Dcom.sun.management.jmxremote.authenticate=false -Dcom.sun.management.jmxremote.ssl=false"
5. Using Default Configuration
Many users set up ActiveMQ with default configurations, neglecting to fine-tune these settings for their specific use case.
Why It Matters
Default settings may not fit your production requirements, leading to performance issues or other unexpected behavior.
Best Practices
- Customize memory limits, max connections, and thread pools according to your needs.
- Review the ActiveMQ documentation for optimal configurations.
<broker xmlns="http://activemq.apache.org/schema/core" brokerName="localhost" dataDirectory="${activemq.data}" >
<systemUsage>
<systemUsage>
<memoryLimit>64 mb</memoryLimit>
<storeUsage>
<storeUsage limit="1 gb"/>
</storeUsage>
<tempUsage>
<tempUsage limit="1 gb"/>
</tempUsage>
</systemUsage>
</systemUsage>
</broker>
6. Ignoring Security
Security should always be a priority, especially when using message brokers that handle sensitive data.
Why It Matters
An unsecured ActiveMQ instance can become a target for various attacks, including unauthorized access and data breach.
Best Practices
- Implement user authentication and authorization mechanisms in ActiveMQ.
- Use SSL/TLS to encrypt communication between clients and the broker.
<transportConnector name="openwire+ssl" uri="ssl://localhost:61617"/>
7. Not Testing in Production-Equivalent Environments
Deploying directly to production without sufficient testing can introduce unseen issues.
Why It Matters
Differences between development, staging, and production environments can lead to problems that arise only in production.
Best Practices
- Use containers (e.g., Docker) to replicate the production environment for testing.
- Conduct load testing to identify performance bottlenecks before going live.
Closing Remarks
ActiveMQ is a robust messaging solution with numerous capabilities. However, to leverage its full potential, it is vital to avoid common pitfalls associated with its deployment in production environments. By understanding these pitfalls and incorporating best practices, you can create a more resilient and efficient messaging system.
Let your ActiveMQ journey be one of success by paying close attention to the insights discussed in this blog. For further reading, check out ActiveMQ Official Documentation and related forums to stay updated with community insights.
By adhering to these principles, you can mitigate risks and ensure that your communication infrastructure is both robust and performant. Happy coding!