The Dangers of Using Java Bullshifier for Code Quality
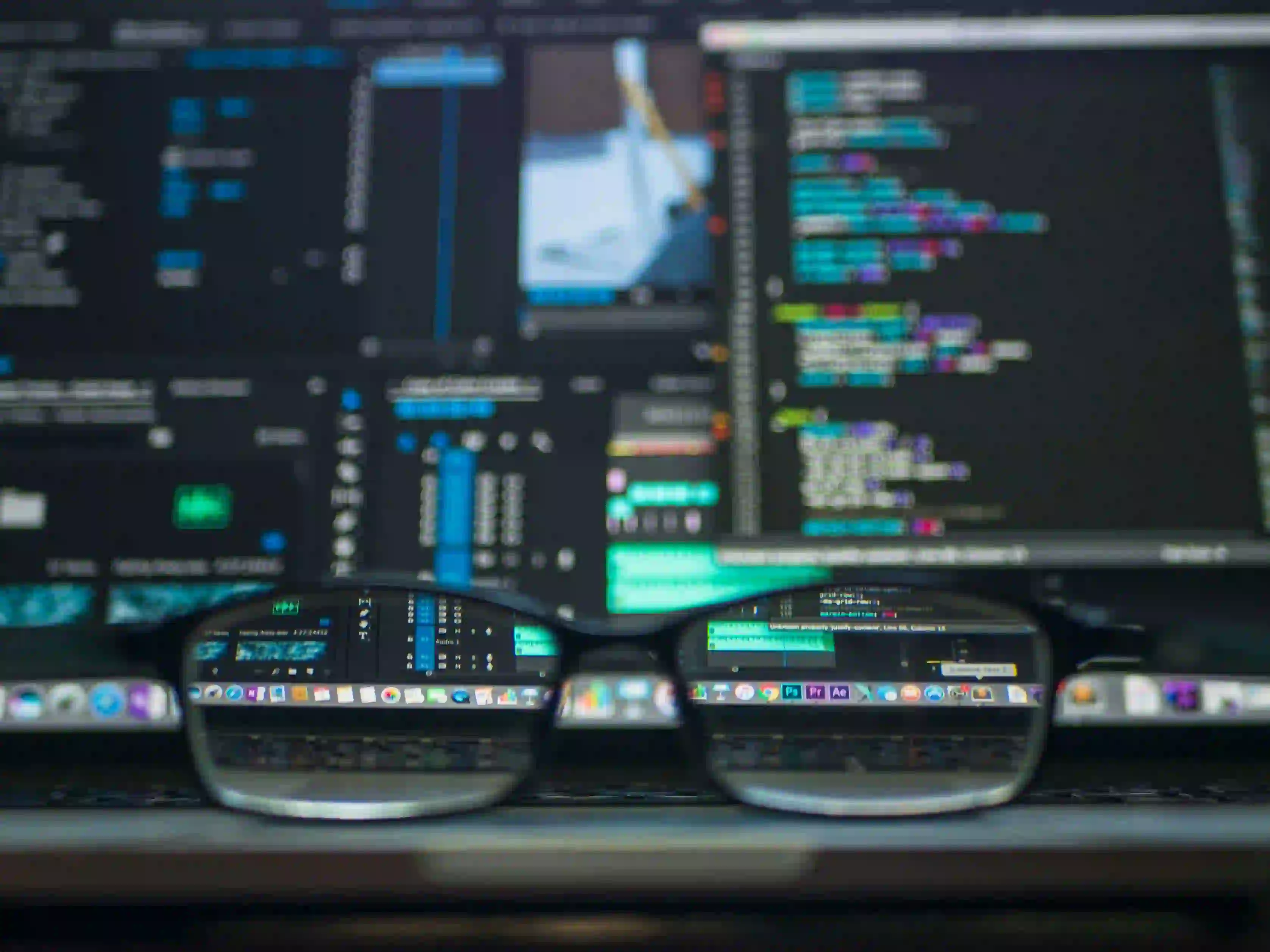
The Dangers of Using Java Bullshifier for Code Quality
In the realm of software development, code quality is a pillar that supports the overall health of an application. As developers, we strive to achieve clean, maintainable, and efficient code. However, various tools emerge in the market claiming to enhance code quality. One such tool is Java Bullshifier. While it might catch the eye of a busy developer, there are some significant dangers associated with relying on it to ensure quality in your Java applications.
What is Java Bullshifier?
Java Bullshifier is a tool that claims to automatically improve code quality by creating additional commentary and embellishing code. It aims to make the code more robust, improve readability, and sometimes even add "fluff" code to impress stakeholders or managers. In essence, Bullshifier focuses on transforming existing code into what it deems "better" code.
However, is it really enhancing code quality, or is it just adding noise? Let’s dig deeper.
The Illusion of Quality
At first glance, it may seem that Java Bullshifier could save time. However, what it offers often results in an illusion of quality. For instance, by adding comments on every line, we might mistakenly believe the code is well-documented.
Example of Misleading Commentary
// This function computes the the sum of two integers
public int sum(int a, int b) {
return a + b; // Adding two numbers
}
Here we see unnecessary commentary. While documentation is essential, over-documenting with vague comments can clutter the code. The same function without superfluous commentary looks much cleaner and conveys its purpose clearly:
public int sum(int a, int b) {
return a + b;
}
The excessive commentary does not enhance understanding of this trivial operation. Instead, code should be self-explanatory wherever possible.
Code Bloat
Java Bullshifier tends to generate a considerable amount of unnecessary code, leading to a phenomenon called "code bloat." This can complicate maintenance and increase a system's cognitive load.
Consider the following example where Bullshifier might introduce redundant classes and methods:
public class NumberManipulator {
public void performOperation(int number) {
// Perform operation with number
double result = number * 1.0; // Unused transformation
}
}
In the above NumberManipulator
example, the method performs an operation that is neither useful nor logical. Performing the operation with unused transformations introduces ambiguity without providing real value.
Complexity Over Simplicity
Simplicity should reign supreme in software development. Yet, tools like Bullshifier can distort this perspective. They can add layers of complexity, which leads to difficulties in debugging, testing, and understanding the code.
Instead of using a complex approach, here’s a simpler, more straightforward method to achieve the intended functionality:
public class NumberManipulator {
public double manipulate(int number) {
return (double) number; // Casting to double directly
}
}
The simpler approach is easier to follow, reducing the overall cognitive effort required by developers interacting with the code.
Loss of Ownership
Relying on Bullshifier can lead developers to lose ownership of their code. When tools begin dictating how code should look and function, developers can slip into a passive role—a dangerous prospect for software craftsmanship.
Losing a sense of ownership often results in decreased pride in one’s work, ultimately leading to lower quality outputs.
False Sense of Security
These tools might give a false sense of security regarding code quality. Developers may assume that if the tool deems the code "better," it is indeed of high quality. This can lead to complacency, which in turn can allow for more significant issues to slip through unnoticed.
Instead of blindly trusting tools, developers should enhance their skills in code review and testing. Tools should augment our capabilities, not replace critical thinking.
Best Practices for Code Quality
Embrace Documentation, but Be Concise
When documenting code, always strive for clarity and relevance. Aim to document the why and what of complex methods rather than the obvious.
Focus on Maintainability
Adopt principles like DRY (Don't Repeat Yourself) and KISS (Keep It Simple, Stupid). It ensures the code remains clean, maintainable, and less prone to bugs.
Perform Regular Code Reviews
Participate in regular code reviews to facilitate knowledge sharing and identify potential issues. Communication among team members can lead to a culture of high-quality standards.
Utilize Linter and Static Analysis Tools
Employing static analysis tools such as SonarQube or Checkstyle can provide more legitimate insights into your code quality. Unlike Bullshifier, these tools focus on specific issues like code smells or bugs without adding unnecessary complexity.
Bringing It All Together
While tools like Java Bullshifier may promise a quick fix to maintaining code quality, they often lead to counterproductive outcomes. The dangers of code bloating, loss of ownership, and false security are significant and can undermine the purpose of developing quality software.
As developers, it is our responsibility to prioritize best practices in coding, maintaining clarity, and ensuring that our code is robust and easy to maintain. Rely on your skills, collaborate with your team, and embrace genuine methods that promote quality in your Java applications.
In the end, the road to high-quality code is illuminated by a commitment to craftsmanship, not by automated tools that obscure true understanding.
Additional Resources
By following a measured and thoughtful approach to coding, you will foster a culture of quality that transcends the mere use of tools, ultimately benefiting the entire software development lifecycle.