Debugging Apache Camel: Uncovering Java Flight Recorder Issues
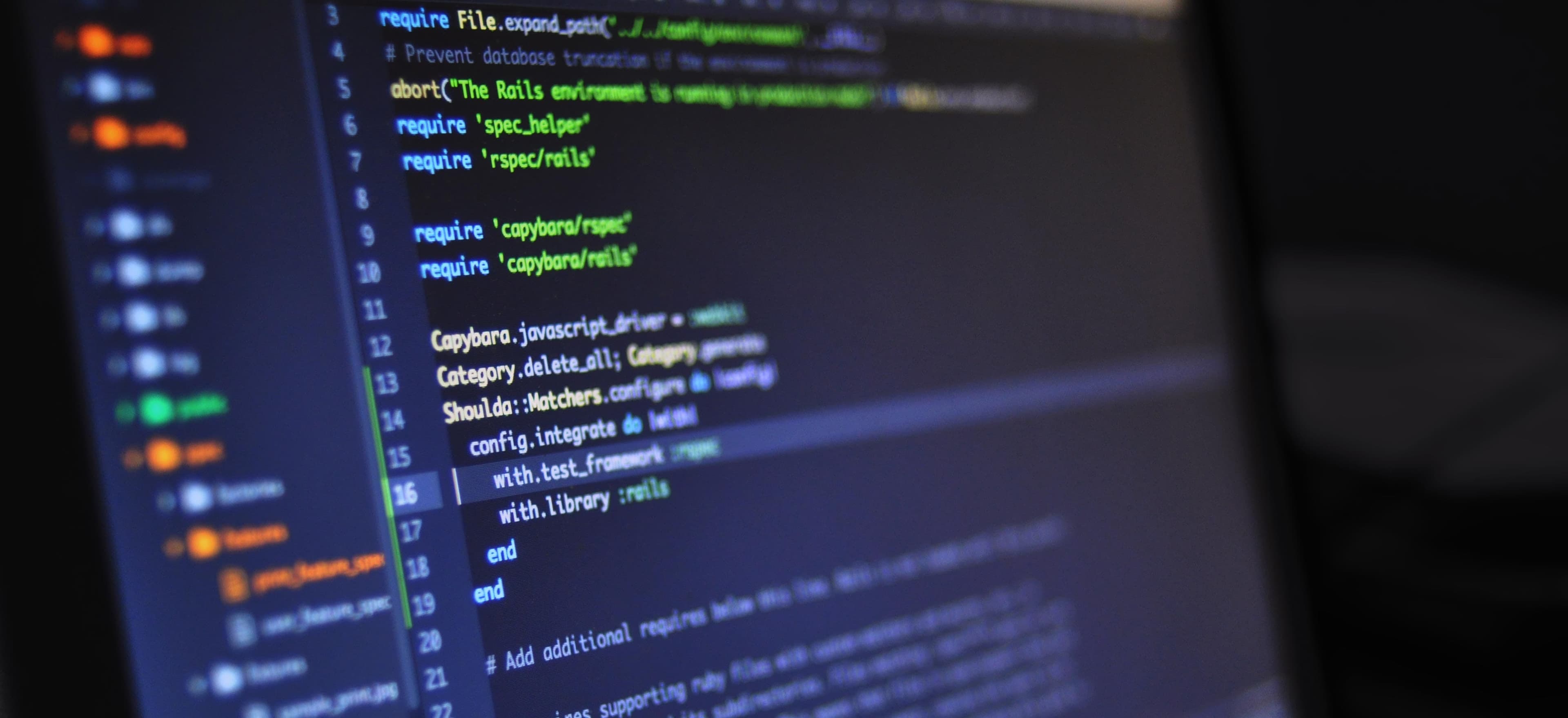
- Published on
Debugging Apache Camel: Uncovering Java Flight Recorder Issues
In today's fast-paced software development ecosystem, debugging performance issues in integration frameworks like Apache Camel is essential for maintaining high application efficiency. This post focuses on leveraging Java Flight Recorder (JFR) to uncover performance hurdles in your Camel routes. We will explore how to utilize JFR effectively and present practical examples to enhance your understanding.
Understanding Apache Camel
Apache Camel is an open-source integration framework that aids developers in implementing various integration patterns seamlessly. With its extensive set of components and straightforward DSL (Domain-Specific Language), Camel simplifies the process of integrating disparate systems.
Benefits of Using Apache Camel
- Simplicity: The Camel DSL allows for intuitive route definitions.
- Flexibility: It supports a wide variety of data formats and transport protocols.
- Scalability: Easily integrates with cloud-based solutions and microservices architectures.
If you want to dive deeper into Camel, check out the official Camel documentation.
The Starting Line to Java Flight Recorder
Java Flight Recorder is a powerful tool integrated into the Java Development Kit (JDK). It enables you to collect and analyze performance data from Java applications at runtime. JFR provides valuable insights concerning CPU usage, memory consumption, threading, and much more.
Key Features of Java Flight Recorder
- Low Overhead: JFR is designed to have minimal impact on application performance.
- Continuous Monitoring: It runs in production, allowing you to gather performance data without stopping your application.
- Rich Data Visualization: Tools like Java Mission Control provide detailed reports on the collected data.
Why Use JFR with Apache Camel?
When integrating multiple systems, performance bottlenecks tend to crop up in various parts of your application. With JFR, you can:
- Monitor Camel route performance.
- Identify slow components or routes that need optimization.
- Analyze memory usage particular to your routes.
Getting Started with Apache Camel and Java Flight Recorder
To effectively debug Apache Camel applications with JFR, you need to ensure you're using a compatible JDK. Java Flight Recorder is bundled with the Oracle JDK and OpenJDK since version 11.
Setting Up JFR
-
Enable JFR on JVM: You can start your Java application with the following options, allowing JFR to capture data:
java -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr -jar my-app.jar
This command records data for 60 seconds and saves it to
myrecording.jfr
. -
Integrate into Your Application Code: Consider adding triggering points in your Camel routes. Use the
@MessageDriven
annotation for message-driven beans, which allows you to begin a JFR recording at a specific route.
Example Camel Route with JFR
Consider the following Camel route that processes file inputs:
from("file:input?fileName=*.txt")
.process(exchange -> {
String body = exchange.getIn().getBody(String.class);
// Potentially slow processing
String processed = expensiveOperation(body);
exchange.getIn().setBody(processed);
})
.to("file:output");
Commentary:
In this example:
- We consume files from a specified directory.
- An expensive operation is performed on the input data.
To identify performance issues, we can leverage JFR by placing timing markers around the expensiveOperation(body)
function.
Using JFR to Profile the Camel Route
-
Add Instrumentation for Profiling: Update the
expensiveOperation
method to include JFR markers:public String expensiveOperation(String input) { long startTime = System.nanoTime(); // Simulate a slow task try { Thread.sleep(1000); // Simulated delay } catch (InterruptedException e) { Thread.currentThread().interrupt(); } long duration = System.nanoTime() - startTime; // Record the performance metric JFR.record("expensiveOperation", duration); return "Processed: " + input; }
-
Create a JFR Event: The
JFR.record()
method logs the performance data in an internal JFR buffer. It can be customized to record additional metrics as needed.
Analyzing the JFR Data
After executing your Camel application, open the recording file (myrecording.jfr
) using Java Mission Control.
- View Thread Activity: Observe how long the
expensiveOperation
took. - Check Memory Usage: Determining if memory consumption spikes during specific operations.
Common Performance Bottlenecks
While using JFR with Apache Camel, you may encounter several performance bottlenecks:
- Slow Routers/Endpoints: Identify which routes are taking longer to process.
- Heavy Payloads: Large messages can slow down processing. Optimize your routes to use lightweight formats like JSON or XML efficiently.
- Data Transformation Issues: Evaluate whether data transformations are done optimally.
Optimization Strategies
Once you've identified areas of concern with JFR, you can take steps towards optimization:
-
Use Asynchronous Processing: Modify your routes to handle processing asynchronously.
from("file:input?fileName=*.txt") .threads(5) // Use a thread pool .process(exchange -> { /* processing */ }) .to("file:output");
-
Batch Processing: Consolidate messages into batches to reduce overhead:
from("file:input?fileName=*.txt") .split(body().tokenize("\n")).streaming() .process(exchange -> { /* processing */ }) .to("file:output");
A Final Look
Debugging performance issues in Apache Camel is crucial for smooth integration and operations. Utilizing Java Flight Recorder is an effective strategy to uncover the underlying performance metrics.
Whether tracking CPU usage, memory consumption, or operation duration, JFR offers vital insights that can help you optimize your Camel routes effectively.
Additional Resources
- Java Flight Recorder Guide
- Apache Camel Documentation
- Java Mission Control Overview
By implementing performance monitoring and enhancement strategies, you can ensure that your integrations run smoothly, leading to improved system performance and reliability. Happy coding!
Checkout our other articles