Troubleshooting Common LDAP Authentication Issues in Spring
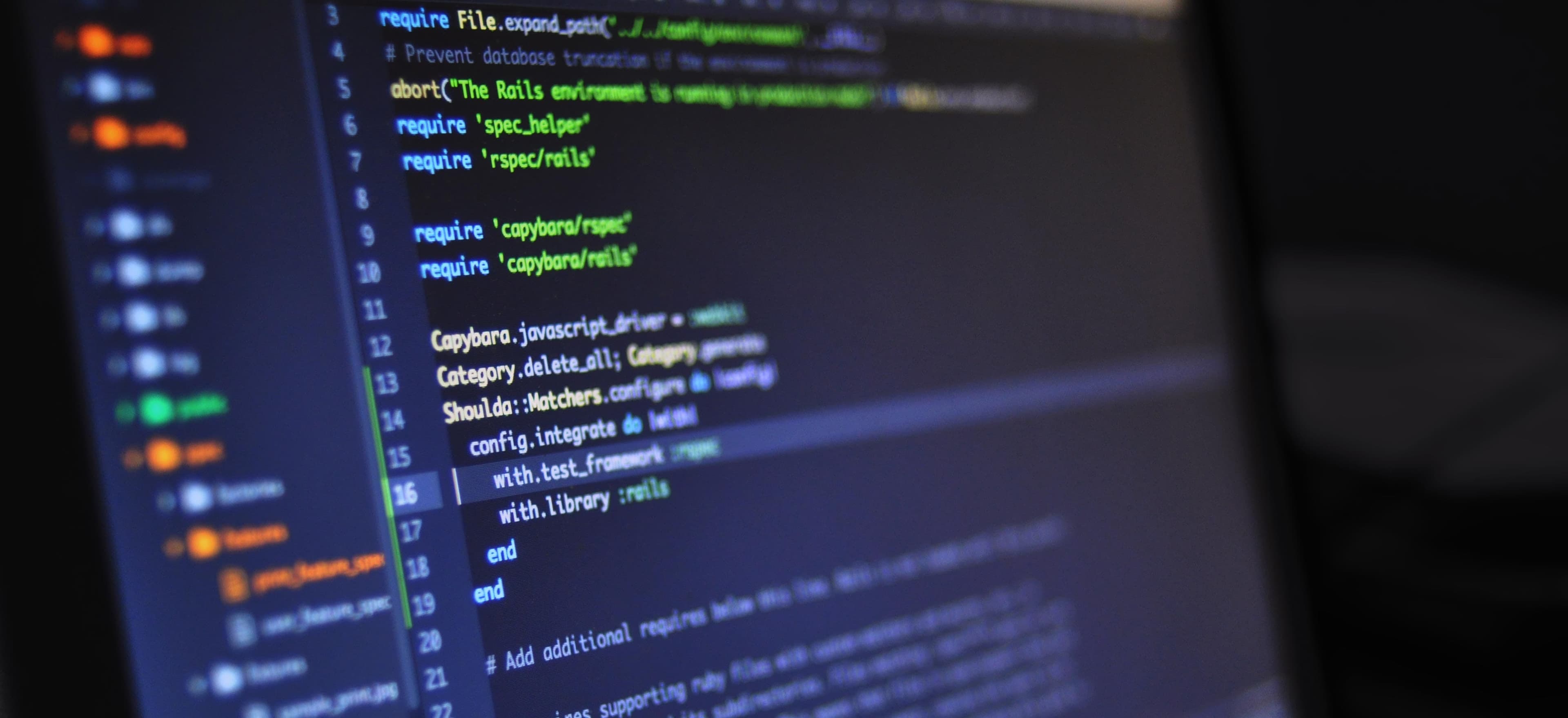
- Published on
Troubleshooting Common LDAP Authentication Issues in Spring
LDAP (Lightweight Directory Access Protocol) is a widely-used protocol for accessing and maintaining distributed directory information services. Spring Security provides excellent support for integrating LDAP authentication into Java applications. However, developers often encounter various challenges during setup and troubleshooting. In this post, we will discuss common LDAP authentication issues in Spring and how to resolve them effectively.
Understanding LDAP Authentication
LDAP authentication is key for many enterprise applications. It allows user credentials to be managed centrally and helps maintain security across different systems. In Spring, integrating LDAP can be done with the spring-boot-starter-ldap
dependency.
To start off, let's look at a basic setup for integrating LDAP in a Spring application.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-ldap</artifactId>
</dependency>
The above dependencies will set up your Spring Boot application for LDAP authentication.
Common Issues to Troubleshoot
1. Incorrect LDAP Configuration
One of the most frequent issues is misconfiguration in the application properties. Common properties include spring.ldap.urls
, spring.ldap.base
, and user DN configurations. Below is an example configuration in application.properties
:
spring.ldap.urls=ldap://localhost:389
spring.ldap.base-dn=dc=example,dc=com
spring.ldap.username=cn=admin,dc=example,dc=com
spring.ldap.password=yourpassword
Why it matters: Proper configuration is essential for connecting to your LDAP server successfully. An incorrect URL or password will lead to authentication failures.
2. Binding Issues
LDAP authentication often requires binding as a particular user before searching for other users. If you encounter issues, check the binding DN.
@Bean
public LdapContextSource contextSource() {
LdapContextSource contextSource = new LdapContextSource();
contextSource.setUrl("ldap://localhost:389");
contextSource.setBase("dc=example,dc=com");
contextSource.setUserDn("cn=admin,dc=example,dc=com");
contextSource.setPassword("yourpassword");
return contextSource;
}
Why it matters: The binding user must have permissions to search for users in the LDAP directory. If the binding fails, your authentication will not work, leading to permission issues.
3. User Not Found
If you are getting an error stating "User not found," you need to check how you are constructing the UserDN
for authentication. The way that usernames are stored in the directory might not match what your application expects.
Here’s how to configure user search in your security configuration:
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.ldapAuthentication()
.userDnPatterns("uid={0},ou=users")
.contextSource(contextSource());
}
Why it matters: The userDnPatterns
defines the format for querying users. If it doesn't match your LDAP structure, it will fail to authenticate users.
4. SSL/TLS Configuration
If your LDAP server requires SSL/TLS, make sure you configure your Spring application accordingly. Here’s how you can enable SSL:
@Bean
public LdapContextSource contextSource() {
LdapContextSource contextSource = new LdapContextSource();
contextSource.setUrl("ldaps://localhost:636");
contextSource.setBase("dc=example,dc=com");
contextSource.setUserDn("cn=admin,dc=example,dc=com");
contextSource.setPassword("yourpassword");
return contextSource;
}
Remember, you also need to ensure that your Java environment trusts the LDAP server's certificate.
Why it matters: Using insecure connections can lead to data breaches or man-in-the-middle attacks. Always prefer secure connections.
5. Insufficient Permissions
Another common issue lies in access control. Ensure that your LDAP user has the necessary permissions to read the entries in your directory.
To check user permissions, you can use tools like Apache Directory Studio, which provides a GUI for inspecting LDAP structures and attributes.
Why it matters: Without proper permissions, even correctly bound users will be unable to authenticate or access necessary attributes, causing a cascade of failures in your application.
6. Exceptions in Logs
Always check the application logs for meaningful exception messages. Common exceptions include AuthenticationFailedException
, InvalidCredentialsException
, and NameNotFoundException
.
You can enhance logging by configuring a logging framework such as Logback or Log4j.
Why it matters: Logs provide direct insights into failures and can help pinpoint the exact step where the authentication process breaks down.
7. Debugging LDAP Queries
You may want to see the actual LDAP queries being sent to your server. You can enable debugging for Spring LDAP using the following settings:
# Logging LDAP queries
logging.level.org.springframework.ldap=DEBUG
Why it matters: Debugging can reveal unforeseen issues such as incorrect queries or unexpected results from the directory.
Lessons Learned
Troubleshooting LDAP authentication issues in Spring can feel daunting, but a systematic approach can help alleviate most common problems. We discussed several common pitfalls and how to effectively resolve them through configuration adjustments and proper logging practices.
By ensuring correct configurations, reviewing permission levels, leveraging debugging, and examining the logs, developers can confidently implement LDAP authentication in their Spring applications.
Remember, for more information on Spring Security and LDAP, check out the official Spring Security Documentation and Spring LDAP Documentation.
Feel free to share your experiences below or any further challenges you’ve faced with LDAP authentication in Spring. Happy coding!
Checkout our other articles