Common Pitfalls in Implementing Asymmetric Encryption
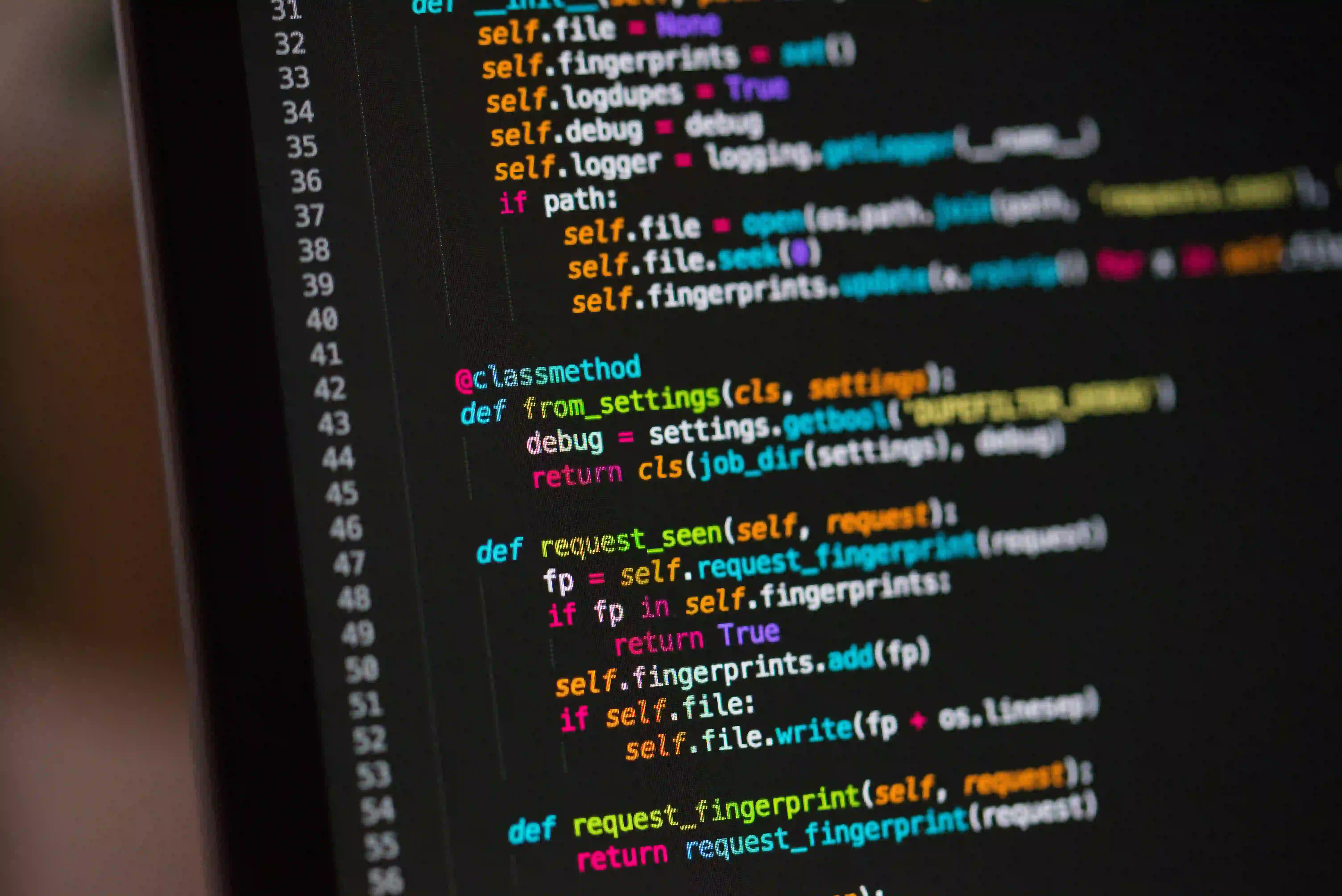
Common Pitfalls in Implementing Asymmetric Encryption
Asymmetric encryption is a vital component of modern cybersecurity. Unlike symmetric encryption, where the same key is used for both encryption and decryption, asymmetric encryption employs a pair of keys: a public key to encrypt data and a private key to decrypt it. This mechanism underpins many security protocols, including SSL/TLS and digitally signing data. However, implementing asymmetric encryption can be fraught with pitfalls. In this blog post, we'll discuss common mistakes to avoid when dealing with asymmetric encryption and provide practical examples to illustrate these points.
1. Mismanagement of Keys
The Importance of Key Management
One of the most significant mistakes developers make is insufficient key management. Asymmetric encryption relies on the confidentiality of the private key. If it gets exposed or mishandled, the security assurances provided by asymmetric cryptography are utterly compromised.
Best Practices for Key Management
- Secure Key Storage: Store private keys in a secure location, such as a hardware security module (HSM) or encrypted storage.
- Regular Rotation: Implement regular key rotation and revocation policies.
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.SecureRandom;
public class KeyManagement {
public static void main(String[] args) throws Exception {
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048, new SecureRandom());
KeyPair pair = keyGen.generateKeyPair();
// Store pair.getPrivate() and pair.getPublic() securely
System.out.println("Private Key: " + pair.getPrivate());
System.out.println("Public Key: " + pair.getPublic());
}
}
Why? This code generates a secure RSA key pair that you should store thoughtfully. Keeping the private key secure is vital to maintain the integrity of your encryption.
2. Weak Key Generation
Key Generation Vulnerabilities
Another common pitfall is using weak keys. The strength of the encryption algorithm relies on the key length and generation process. Using short or predictable keys can lead to vulnerabilities.
Recommendations
- Use Recommended Key Sizes: Generally, RSA keys should be at least 2048 bits.
- Randomness is Key: Use a reliable source of randomness (like
SecureRandom
) to generate keys.
import java.security.KeyPairGenerator;
public class KeyStrength {
public static void main(String[] args) throws Exception {
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048); // Ensure key size is 2048 or greater
System.out.println("Key size: " + keyGen.getKeySize());
}
}
Why? This snippet ensures your key size meets recommended standards, crucial for maintaining strong security against brute force attacks.
3. Lack of Proper Error Handling
Importance of Error Reporting
Implementing asymmetric encryption without adequate error handling can expose your application to vulnerabilities. If errors go unreported, it becomes difficult to track down issues for both encryption and decryption processes.
Strategies for Handling Errors
- Log Errors: Always log the errors when key operations fail.
- Graceful Degradation: Ensure the system can handle failures gracefully without exposing sensitive information.
import java.security.PrivateKey;
import java.security.Signature;
public class ErrorHandling {
public static void signData(byte[] data, PrivateKey privateKey) {
try {
Signature signature = Signature.getInstance("SHA256withRSA");
signature.initSign(privateKey);
signature.update(data);
byte[] signedData = signature.sign();
System.out.println("Data signed successfully.");
} catch (Exception e) {
// Log the error without exposing sensitive information
System.err.println("Error during signing process: " + e.getMessage());
}
}
}
Why? This example demonstrates good error handling practices. It prevents sensitive error details from being exposed while logging sufficient information for troubleshooting.
4. Not Using Digital Signatures
The Value of Digital Signatures
Ignoring the importance of digital signatures can undermine the entire purpose of using asymmetric encryption. Digital signatures provide a mechanism for verifying the authenticity and integrity of a message.
How to Implement Digital Signatures
import java.security.PublicKey;
import java.security.Signature;
public class DigitalSignature {
public static boolean verifySignature(byte[] data, byte[] signatureBytes, PublicKey publicKey) {
try {
Signature signature = Signature.getInstance("SHA256withRSA");
signature.initVerify(publicKey);
signature.update(data);
return signature.verify(signatureBytes); // Return true if signature is valid
} catch (Exception e) {
e.printStackTrace();
return false; // Return false on failure
}
}
}
Why? This code verifies the digital signature using a public key. Signing the data during transmission adds a crucial layer of security to your communication.
5. Overcomplicating Implementation
Keep It Simple
Many developers strive for fancy implementations that can obfuscate the purpose of asymmetric encryption. This often leads to insecure practices or vulnerabilities in the code.
Simple and Effective Approach
Stick to established libraries and frameworks that have been tried and tested. The Java Cryptography Architecture (JCA) provides built-in classes for most operations.
import javax.crypto.Cipher;
import java.security.PublicKey;
public class SimpleEncryption {
public static byte[] encryptData(byte[] data, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data); // Encrypt the data
}
}
Why? This straightforward code snippet utilizes JCA to encrypt data using a public key. Keeping the process simple helps in reducing potential mistakes.
Final Thoughts
Implementing asymmetric encryption is undeniably challenging but rewarding when executed correctly. By understanding and avoiding the common pitfalls discussed in this blog, you can strengthen your security measures and better protect sensitive information.
For a more in-depth understanding of encryption techniques, you might find useful resources at OWASP's Guide to Cryptography or NIST's Special Publication on Cryptographic Standards.
By following best practices — from effective key management, robust error handling, and leveraging digital signatures, to keeping the implementation as simple as possible — you can navigate the complexities of asymmetric encryption confidently. Happy coding!