The Pitfalls of Field Setters in Constructor Injection
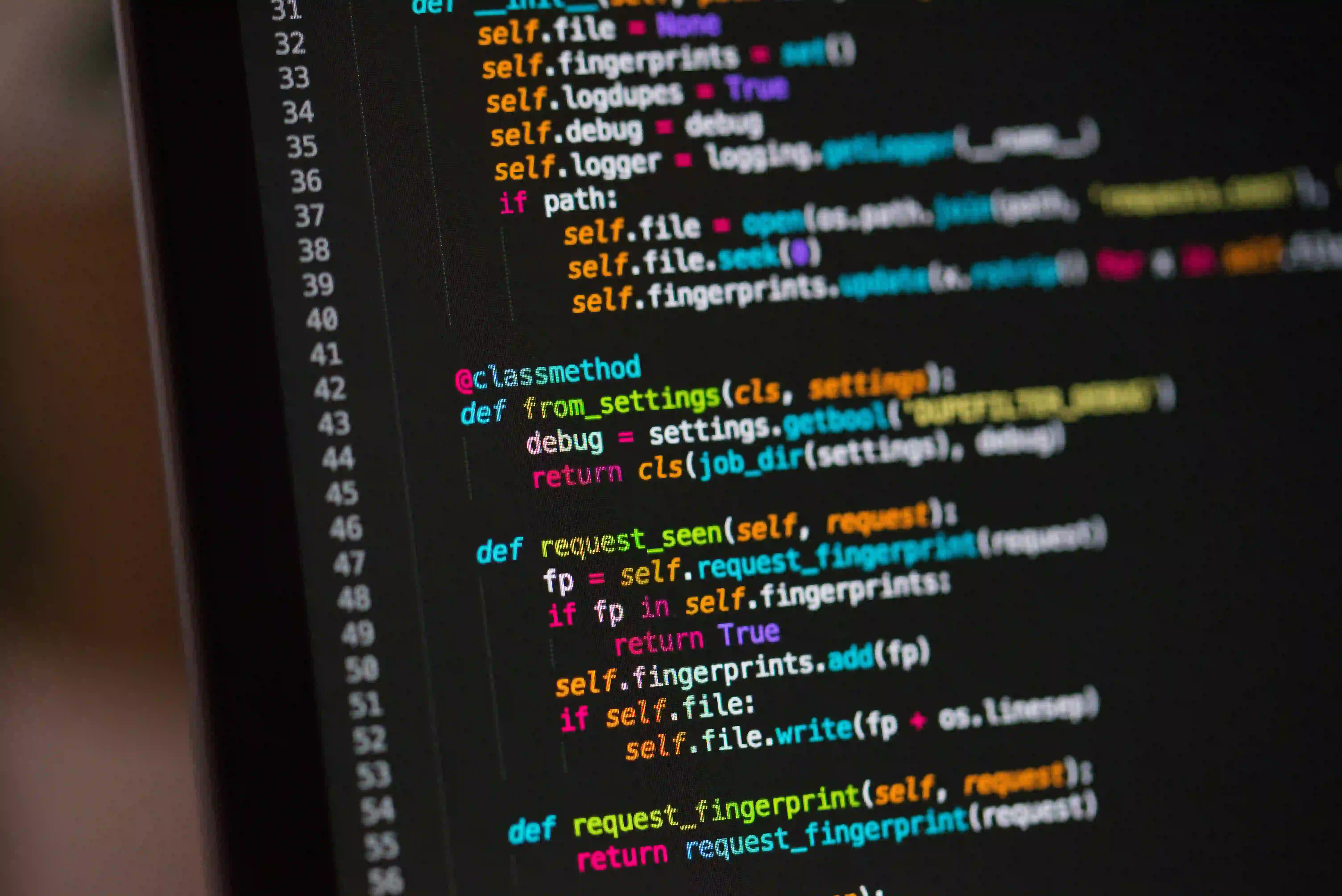
The Pitfalls of Field Setters in Constructor Injection
Constructor injection is a design pattern commonly used in object-oriented programming, particularly in Java, to manage dependencies. It ensures that a class has all necessary dependencies at the time of instantiation, fostering immutability and promoting good design practices. However, when field setters are introduced alongside constructor injection, they can lead to several pitfalls that compromise the intended benefits of this pattern. In this post, we'll explore these pitfalls, provide examples, and discuss best practices that can help you maintain clean and effective code.
Understanding Constructor Injection
Before delving into the pitfalls of field setters, let's clarify what constructor injection is. Constructor injection is the process of passing dependencies to a class through its constructor instead of setting them after the object has been created.
Example of Constructor Injection
Below is a simple example demonstrating constructor injection in Java:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void registerUser(User user) {
// Register logic using userRepository
userRepository.save(user);
}
}
In this example, UserService
requires a UserRepository
to function. By using constructor injection, we ensure that a valid UserRepository
is provided during the creation of UserService
.
Benefits of Constructor Injection
- Immutability: Dependencies are final and cannot be reassigned, promoting safer code.
- Mandatory Dependencies: If a class cannot function without certain dependencies, constructor injection enforces their presence.
- Easier Testing: Dependencies can easily be mocked or substituted, making unit tests more straightforward.
The Problem with Field Setters
While constructor injection has numerous advantages, introducing field setters can nullify many of these benefits. Here are the key pitfalls associated with field setters in conjunction with constructor injection:
1. Breaching Immutability
When field setters are employed, you can modify the instance variables after the object has been constructed. This breaches the principle of immutability guaranteed by constructor injection.
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository; // Immutability is compromised
}
}
Having a setter method allows object state to change unexpectedly, leading to potential issues where the class no longer has guaranteed behavior. This can make the class harder to maintain and understand.
2. Inconsistent State
Field setters can lead to a situation where an object exists in an inconsistent state. For example, if the UserRepository
is set to null
, any method expecting a valid repository would throw a NullPointerException
.
Example Code
Consider the following:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository; // Potential inconsistency
}
public void registerUser(User user) {
if (userRepository == null) {
throw new IllegalStateException("UserRepository must not be null");
}
userRepository.save(user);
}
}
Here, invoking registerUser
without having a valid userRepository
set can crash the application. This leads to hard-to-track bugs.
3. Dependency Injection Misconfiguration
Field setters can inadvertently lead to misuse of the dependency injection. If the dependencies are expected to be injected at creation, altering them from within the class can introduce errors.
Example Code
public class UserService {
private UserRepository userRepository;
// Constructor for initial setup
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void setUserRepository(UserRepository userRepository) {
if (userRepository == null) {
throw new IllegalArgumentException("UserRepository must not be null");
}
this.userRepository = userRepository;
}
}
An unexpected call to setUserRepository()
with a null
argument can lead to runtime exceptions. This situation can be avoided entirely by not having any setters in the first place.
4. Testing Complications
Constructing test cases becomes cumbersome when field setters are present. If dependencies are swapped after the object has been created, then tests must also account for the object's state after potential changes.
@Test
public void testRegisterUser() {
UserRepository mockRepo = Mockito.mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
// Changing the UserRepository after the construction
userService.setUserRepository(null); // Not valid in production code
// Will lead to an error
assertThrows(IllegalStateException.class, () -> userService.registerUser(new User()));
}
Confusion grows when the test outcome depends on the state from setters, leading to unreliable tests.
Best Practices
To maximize the benefits of constructor injection and avoid pitfalls with field setters, consider the following best practices:
-
Avoid Field Setters: Whenever possible, refrain from providing setters for fields that are essential to the operation of the class. This promotes immutability and simplifies object state management.
-
Use Constructor for All Dependencies: Even if certain dependencies are optional, consider defining them all in the constructor to maintain consistency.
-
Leverage Factory Patterns: If you need different instances of the class with various configurations, create factory methods or classes to handle this.
-
Implement Builder Pattern: For classes with numerous dependencies, using a builder pattern can reduce complexity while maintaining immutability.
Example Using Builder Pattern
public class UserService {
private final UserRepository userRepository;
private UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private UserRepository userRepository;
public Builder userRepository(UserRepository userRepository) {
this.userRepository = userRepository;
return this;
}
public UserService build() {
return new UserService(userRepository);
}
}
// Other methods...
}
Using a builder pattern allows flexible construction without compromising immutability.
Closing the Chapter
Constructor injection is a powerful pattern that provides clarity and reliability in your Java applications. However, combining it with field setters can lead to several pitfalls, such as inconsistent state, breaches of immutability, and complicated testing.
By avoiding field setters and adhering to best practices such as factory methods or the builder pattern, you can ensure your classes are easier to maintain and more predictable in behavior.
If you wish to delve deeper into Dependency Injection in Java, consider exploring resources like the Spring Framework Documentation or the Google Guice Documentation. Happy coding!