Mastering AI Pathfinding for JavaFX Tower Defense Games
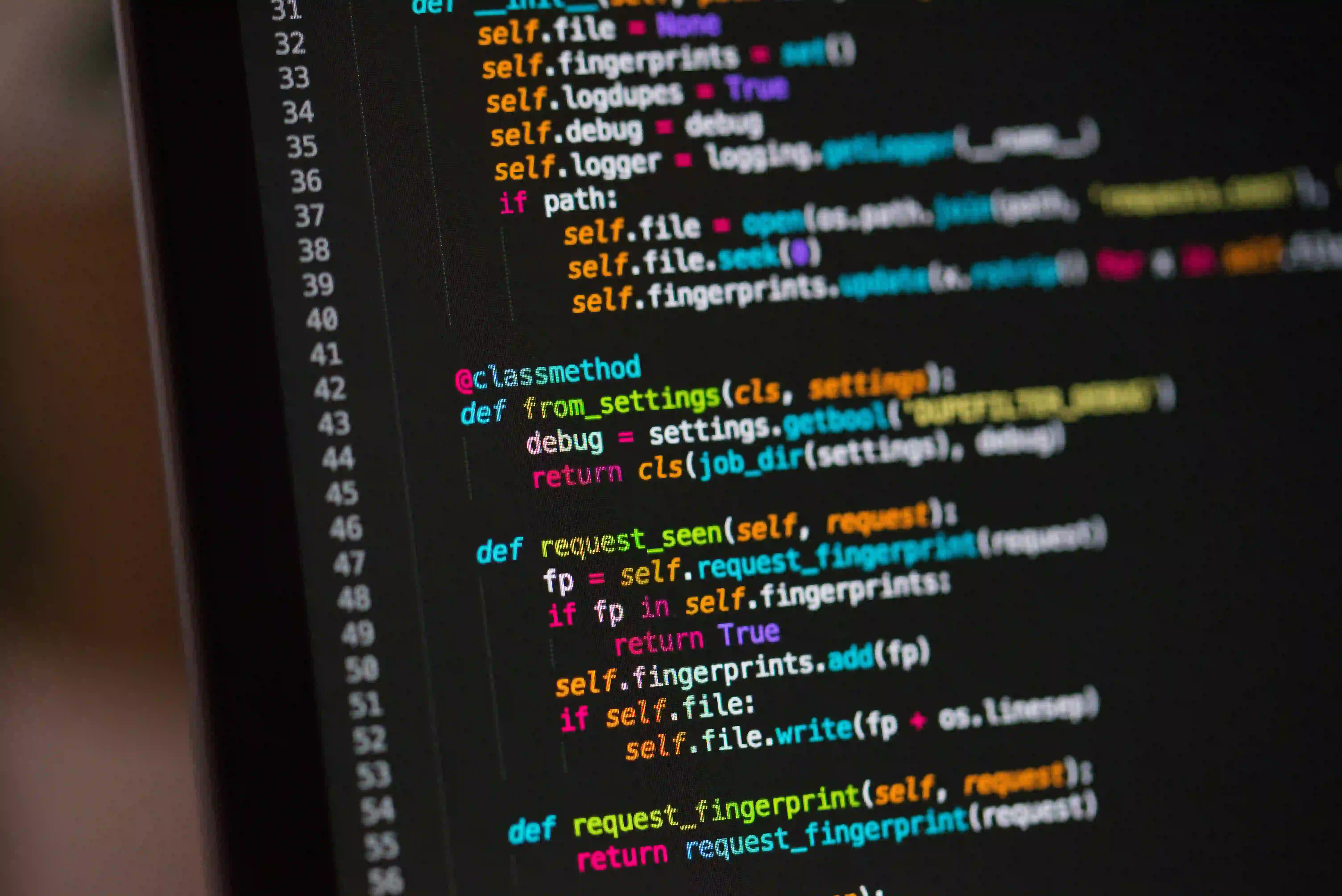
Mastering AI Pathfinding for JavaFX Tower Defense Games
If you are venturing into the realm of game development, particularly in creating tower defense games using JavaFX, mastering AI pathfinding is crucial. In this blog post, we will delve into the intricacies of pathfinding algorithms, primarily focusing on *A (A-Star)**, and how you can implement it in your JavaFX tower defense game. By the end of this article, you will have a solid understanding of how AI can intelligently navigate a game grid, bypassing obstacles while targeting a goal.
Table of Contents
- Understanding Pathfinding
- Choosing the Right Algorithm
- Implementing A* Pathfinding Algorithm
- Visualizing Pathfinding with JavaFX
- Integrating Pathfinding into Your Game
- Additional Resources
Understanding Pathfinding
Pathfinding is the process of determining a path from a starting point to a target point. In a tower defense game, this involves navigating the AI-controlled enemies from their spawn point to the player's base while considering obstacles such as towers.
JavaFX provides a graphical and interactive environment suitable for creating visually stunning games. However, the core functionality of AI pathfinding must be implemented efficiently to ensure a smooth gaming experience.
Choosing the Right Algorithm
Different pathfinding algorithms exist, each with its strengths and weaknesses. Here’s a brief comparison of some popular algorithms:
- Dijkstra’s Algorithm: Guarantees the shortest path, but is computationally expensive for larger grids.
- Breadth-First Search (BFS): Simple to implement and works well for unweighted graphs but doesn't always find the shortest path in weighted graphs.
- *A (A-Star)**: Combines the advantages of Dijkstra’s Algorithm and heuristic methods, making it efficient for most games.
For a tower defense game, A* is widely regarded as the best choice due to its balance between performance and optimality.
Implementing A* Pathfinding Algorithm
Let's dive into how to implement the A* algorithm in your JavaFX tower defense game.
The A* Algorithm Overview
A* works by maintaining a priority queue of nodes to explore based on two costs:
- g(n): The cost from the starting node to any node n.
- h(n): The heuristic estimated cost from node n to the goal (common heuristics include Manhattan or Euclidean distance).
The algorithm continues until the goal is reached or all nodes are explored.
Code Snippet: Basic Structure of A*
Here’s a simplified version of the A* algorithm in Java:
import java.util.*;
public class AStarPathfinding {
static class Node {
int x, y;
Node parent;
double g, h;
Node(int x, int y) {
this.x = x;
this.y = y;
this.g = Double.MAX_VALUE;
this.h = 0;
}
double f() {
return g + h; // Total cost function
}
}
public List<Node> findPath(Node start, Node goal, boolean[][] grid) {
PriorityQueue<Node> openSet = new PriorityQueue<>(Comparator.comparingDouble(Node::f));
Set<Node> closedSet = new HashSet<>();
start.g = 0;
start.h = heuristic(start, goal);
openSet.add(start);
while (!openSet.isEmpty()) {
Node current = openSet.poll();
if (current.equals(goal)) {
return reconstructPath(current);
}
closedSet.add(current);
for (Node neighbor : getNeighbors(current, grid)) {
if (closedSet.contains(neighbor)) continue;
double tentativeGScore = current.g + 1; // Assuming cost between neighboring nodes is 1
if (tentativeGScore < neighbor.g) {
neighbor.parent = current;
neighbor.g = tentativeGScore;
neighbor.h = heuristic(neighbor, goal);
if (!openSet.contains(neighbor)) {
openSet.add(neighbor);
}
}
}
}
return Collections.emptyList(); // Return empty if no path is found
}
private double heuristic(Node a, Node b) {
return Math.abs(a.x - b.x) + Math.abs(a.y - b.y); // Manhattan distance
}
private List<Node> reconstructPath(Node node) {
List<Node> path = new ArrayList<>();
while (node != null) {
path.add(node);
node = node.parent;
}
Collections.reverse(path);
return path; // Return reversed path
}
private List<Node> getNeighbors(Node node, boolean[][] grid) {
List<Node> neighbors = new ArrayList<>();
// Implement logic to get valid neighboring nodes...
return neighbors;
}
}
Commentary on the Code
- Node Class: Each node represents a point on the grid (either passable or blocked).
- findPath Method: This method implements the A* algorithm. It maintains a list of nodes to explore (
openSet
) and a list of explored nodes (closedSet
). - Heuristic Function: In this case, we use the Manhattan distance, which is suitable for grid-based games.
- Reconstructing the Path: We backtrack from the goal to create the path upon completion.
Implementing these components will allow your A* search to find the most efficient path for your enemies effectively.
Visualizing Pathfinding with JavaFX
With the A* algorithm set up, it's time to visualize the pathfinding in JavaFX.
Code Snippet: Visualizing the Path
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class PathfindingVisualization extends Application {
private static final int TILE_SIZE = 30;
private static final int GRID_SIZE = 10;
@Override
public void start(Stage primaryStage) {
Pane root = new Pane();
boolean[][] grid = new boolean[GRID_SIZE][GRID_SIZE]; // Example grid setup
Node start = new Node(0, 0);
Node goal = new Node(9, 9);
AStarPathfinding pathfinder = new AStarPathfinding();
List<Node> path = pathfinder.findPath(start, goal, grid);
drawGrid(root, path);
Scene scene = new Scene(root, GRID_SIZE * TILE_SIZE, GRID_SIZE * TILE_SIZE);
primaryStage.setTitle("Pathfinding Visualization");
primaryStage.setScene(scene);
primaryStage.show();
}
private void drawGrid(Pane root, List<Node> path) {
for (int i = 0; i < GRID_SIZE; i++) {
for (int j = 0; j < GRID_SIZE; j++) {
Rectangle rect = new Rectangle(i * TILE_SIZE, j * TILE_SIZE, TILE_SIZE, TILE_SIZE);
rect.setFill(Color.LIGHTGRAY);
root.getChildren().add(rect);
}
}
// Highlight the path
if (!path.isEmpty()) {
for (Node node : path) {
Rectangle rect = new Rectangle(node.x * TILE_SIZE, node.y * TILE_SIZE, TILE_SIZE, TILE_SIZE);
rect.setFill(Color.GREEN);
root.getChildren().add(rect);
}
}
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Visualization
- JavaFX Pane: We create a
Pane
to draw the grid. Each cell represents a node in our grid. - Grid Visualization: The grid is rendered with gray tiles, while the path found by A* is highlighted in green.
By running this JavaFX application, you will visualize how the pathfinding algorithm works, seeing how the enemies would navigate through the grid.
Integrating Pathfinding into Your Game
Once you have the A* pathfinding and visualization set up, integrating it into your tower defense game is systematic:
- Implement Enemy AI: Each enemy should call the
findPath
method to determine its route to the player's base. - Update Enemy Goals: Continuously update the enemy's path as towers are built, possibly recalculating their path in real-time.
- Handle Obstacles: Represent towers as obstacles in your grid to ensure enemies do not walk through them.
Additional Resources
Here are some resources for further learning on pathfinding and game development:
- A* Pathfinding Project
- JavaFX Official Documentation
- Game Development using Java
Final Thoughts
Mastering AI pathfinding, particularly the A* algorithm, is a cornerstone of developing intelligent and engaging tower defense games using JavaFX. By understanding how the algorithm operates and integrating it within your game's architecture, you can create dynamic gameplay experiences that keep players engaged.
In pursuit of game development, never stop exploring new ways to improve your AI and game mechanics. With constant updates and community engagement, you’ll find boundless opportunities to enhance your development skills. Happy coding!