Mastering EDT: Solve InvokeAndBlock Challenges in Swing
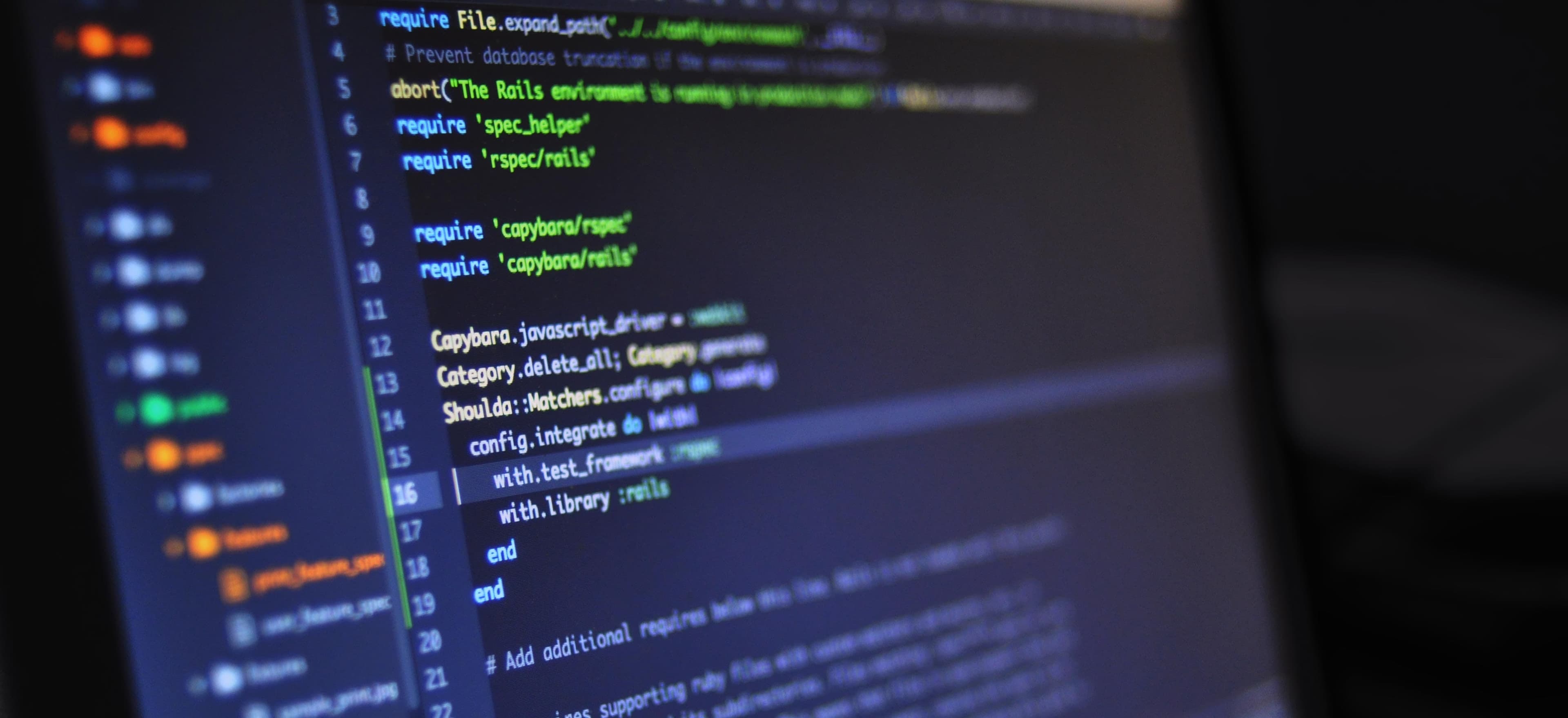
- Published on
Mastering EDT: Solving InvokeAndBlock Challenges in Swing
Java Swing is a powerful toolkit for building graphical user interfaces (GUIs). However, managing the complexity of GUI applications can be daunting, particularly when it comes to threading. One key area where developers frequently encounter difficulties is the Event Dispatch Thread (EDT). In this blog post, we will delve into the nuances of the EDT, specifically focusing on the invokeAndBlock
dilemma within Swing applications.
Understanding the Event Dispatch Thread (EDT)
The Event Dispatch Thread is crucial in Swing applications. All GUI-related tasks such as painting components, handling events, or updating the user interface must occur on this thread to ensure thread safety. If you try to manipulate Swing components from a non-EDT thread, you risk creating unstable applications, leading to unpredictable behavior.
What is invokeAndBlock
?
invokeAndBlock
is not a standard Java Swing method but serves a distinct purpose in some libraries or custom implementations. In essence, it simulates a blocking call to the EDT, allowing a developer to execute GUI updates or event handling in a synchronous manner. This can be especially useful when performing long-running tasks that need to communicate with the GUI during or after completion.
However, using invokeAndBlock
can lead to deadlocks if not applied carefully, especially if the code block is executed on the EDT itself or if it waits on resources locked by the EDT.
Why is Thread Safety Important?
Thread safety ensures that data remains consistent in concurrent operations. Swing enforces this through the using of the EDT for all GUI-related tasks. When multiple threads attempt to access and modify GUI components concurrently, it can lead to corrupt your application's state, resulting in crashes or visually inconsistent UIs.
Example: Updating the UI from a Background Thread
Consider a scenario where you are fetching data from an API using a background thread. The aim is to update a label on the GUI once the data is received. For this, you can use the SwingUtilities.invokeLater()
method, which ensures that the update happens on the EDT.
Here’s a code snippet illustrating this:
import javax.swing.*;
import java.awt.*;
public class BackgroundTaskExample {
private JLabel label;
public BackgroundTaskExample() {
JFrame frame = new JFrame("Background Task Example");
label = new JLabel("Waiting for data...");
frame.add(label, BorderLayout.CENTER);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
fetchData();
}
private void fetchData() {
new Thread(() -> {
String data = fetchDataFromAPI(); // Long-running operation.
SwingUtilities.invokeLater(() -> updateLabel(data)); // Update UI on EDT.
}).start();
}
private String fetchDataFromAPI() {
try {
Thread.sleep(2000); // Simulating network delay.
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Data received from API!";
}
private void updateLabel(String data) {
label.setText(data); // GUI update on EDT.
}
public static void main(String[] args) {
SwingUtilities.invokeLater(BackgroundTaskExample::new);
}
}
Commentary on the Example
-
Decoupling UI from the Data Layer: The application fetches data in a background thread, ensuring the UI remains responsive.
-
Safe UI Updates: The
SwingUtilities.invokeLater()
method safely schedulesupdateLabel(data)
for execution on the EDT, thus preventing potential threading issues. -
Separation of Concerns: By separating data-fetching logic from UI updates, maintenance becomes more manageable, fostering cleaner code.
Challenges with invokeAndBlock
As previously mentioned, while invokeAndBlock
might appear to simplify UI interactions, it can have pitfalls, particularly regarding responsiveness and deadlock scenarios. The following points elucidate these challenges:
1. Susceptibility to Deadlocks
Deadlocks occur when two threads block each other from proceeding. If one thread is waiting for a resource held by another, and that second thread is waiting for the first, you have a classic deadlock scenario. Using invokeAndBlock
on the EDT can potentially exacerbate this problem.
2. Poor UI Responsiveness
When long-running tasks are invoked on the EDT, the GUI becomes unresponsive for the duration of the task. Users may interpret this as software hanging, which can lead to a poor user experience.
Example: Bad Practice with invokeAndBlock
Supposing you invoke a blocking call directly on the EDT, consider the following contrived example:
private void invokeAndBlockExample() {
// This method should never be on the EDT!
invokeAndBlock(() -> {
// Simulating a long-running operation
Thread.sleep(5000); // Blocking the EDT
});
}
Why This is Harmful
- UI Freeze: The GUI will be unresponsive for 5 seconds, leading to a frustrating user experience.
- Risk of Deadlocks: If other operations are also trying to access resources held by this method while it is executing, a deadlock can easily occur.
Best Practices for Handling EDT
To navigate the complexities surrounding the EDT, consider the following tried-and-true techniques:
Use Background Workers
For more extensive tasks, consider using SwingWorker
. It provides built-in support for background processing and safely handling updates to the UI.
private class MyWorker extends SwingWorker<String, Void> {
@Override
protected String doInBackground() {
// Long running Task
return fetchDataFromAPI();
}
@Override
protected void done() {
try {
String result = get(); // Retrieves the result from doInBackground
updateLabel(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Rely on Timer Tasks
If periodic tasks need to occur, consider using javax.swing.Timer
, which executes an action on the EDT, thereby simplifying timing operations without blocking the thread.
Always Delegate GUI Updates to the EDT
Whenever a task involves updating the GUI, remember to delegate it back to the EDT using methods such as invokeLater()
or a scheduled SwingWorker
.
Bringing It All Together
Mastering the Event Dispatch Thread is vital for any Swing developer. It ensures your applications are responsive, deadlock-free, and behave predictably in multi-threaded environments. Utilizing concepts like background threading, utilizing tools such as SwingWorker
, and comprehensively understanding the implications of invokeAndBlock
will significantly improve your Swing applications.
For further reading on developing robust applications with Swing, check out Oracle's Swing Tutorial and Effective Java by Joshua Bloch. Both resources offer foundational knowledge beneficial to both novices and experienced developers seeking to refine their skills.
By adhering to these best practices, you will not only enhance your projects' performance but also provide a better experience for the end-user. Happy coding!
Checkout our other articles