Mastering Circuit Breakers in Envoy Sidecar Proxies
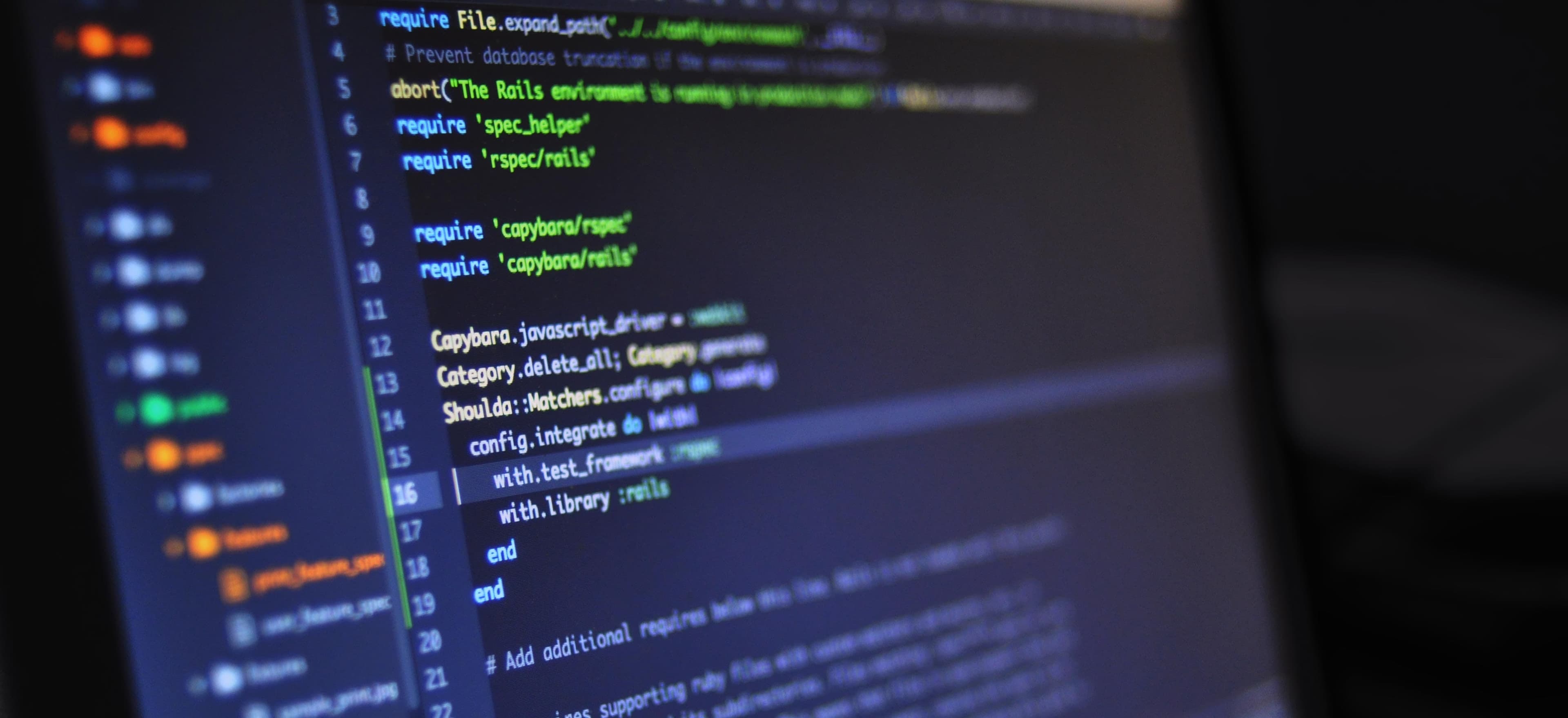
- Published on
Mastering Circuit Breakers in Envoy Sidecar Proxies
In the realm of microservices architecture, the reliability and resilience of applications are critical. One of the essential patterns to enhance these attributes is the Circuit Breaker pattern. In this post, we'll dive deep into Circuit Breakers within Envoy, a popular high-performance, open-source edge and service proxy. We will dissect how Envoy implements Circuit Breakers and how you can master their usage in your microservices.
What is Envoy?
Envoy is an open-source edge and service proxy designed for cloud-native applications. It provides advanced traffic management, load balancing, service discovery, and observability features. Envoy can be deployed as a sidecar proxy, which sits alongside your service instances, or as a standalone proxy managing access to services.
Understanding Circuit Breakers
Before exploring how Circuit Breakers are implemented in Envoy, let’s understand what a Circuit Breaker is.
In brief, a Circuit Breaker is a design pattern used in distributed systems to prevent cascading failures. It is akin to an electrical circuit breaker, which shuts off current to prevent damage. When a service is called and it fails repeatedly, the Circuit Breaker trips, preventing further attempts to call that service until it has a chance to recover. In the context of Envoy, Circuit Breakers can be configured to manage traffic to unhealthy services, thereby improving the application's reliability.
Why Use Circuit Breakers?
- Fault Tolerance: Prevents your application from calling services that are known to be down.
- Graceful Degradation: Allows your application to maintain functionality even when a service is unavailable.
- Performance Improvement: Reduces unnecessary load on services that are struggling, allowing them a chance to recover.
Implementing Circuit Breakers in Envoy
Now that we understand the purpose of Circuit Breakers, let’s dive into how to implement them in Envoy.
Basic Configuration
Envoy’s Circuit Breaker functionality is part of its configuration settings, typically found in the Route
and Cluster
settings. Below is a basic configuration example.
static_resources:
clusters:
- name: service_a
connect_timeout: 0.25s
type: STRICT_DNS
lb_policy: ROUND_ROBIN
load_assignment:
cluster_name: service_a
endpoints:
- lb_endpoints:
- endpoint:
address:
socket_address: { address: service-a.default.svc.cluster.local, port_value: 80 }
circuit_breakers:
thresholds:
- max_connections: 100
max_pending_requests: 10
max_requests: 50
max_retries: 3
Breaking Down the Configuration
connect_timeout
: Sets the timeout for establishing a connection. In this case, it is 0.25 seconds.type
: Defines how the service endpoint is resolved. Here,STRICT_DNS
means Envoy uses DNS for service discovery.lb_policy
: This defines the load balancing policy -ROUND_ROBIN
evenly distributes traffic across instances.- The
circuit_breakers
section is critical for resilience.
Each threshold specifies:
max_connections
: Limiting the maximum number of connections to the service.max_pending_requests
: The maximum requests that can be queued when connections are at capacity.max_requests
: The total max requests that Envoy will actively process at any given time.max_retries
: The maximum number of retry attempts for failed requests.
Configuring Circuit Breaker for Unhealthy Services
In an operational environment, you might want to adjust the Circuit Breaker settings dynamically based on how your services are performing.
Here's how you can achieve that using dynamic configuration:
apiVersion: v1
kind: ConfigMap
metadata:
name: envoy-config
data:
envoy.yaml: |
static_resources:
clusters:
- name: service_a
connect_timeout: 0.5s
circuit_breakers:
thresholds:
- max_connections: 10
max_pending_requests: 5
max_requests: 2
Using Health Check and Circuit Breakers Together
Envoy also allows you to configure health checks to determine if a service is available. Implementing health checks alongside Circuit Breakers can create a powerful synergy, resulting in more resilient applications.
Here is an example:
static_resources:
clusters:
- name: service_a
...
health_checks:
- timeout: 0.5s
interval: 5s
unhealthable:
threshold: 10
health_checker:
tcp_health_check: {}
Monitoring Circuit Breakers
Monitoring can provide insights into how well your Circuit Breakers are working. Envoy supports statistics and metrics via Prometheus. Here’s how you can integrate metrics to monitor your Circuit Breakers:
admin:
access_log_path: /tmp/admin_access.log
address:
socket_address: { address: 0.0.0.0, port_value: 9901 }
In your Prometheus configuration, you can add:
scrape_configs:
- job_name: envoy
static_configs:
- targets: ['localhost:9901']
By monitoring various metrics, such as cluster.service_a.circuit_breakers.active
, you can evaluate how frequently your Circuit Breaker trips.
Best Practices for Using Circuit Breakers
-
Tune Your Settings: Circuit Breaker settings should not be one-size-fits-all. Monitor the load and tune the thresholds according to your applications' traffic patterns.
-
Combine Patterns: Consider using Circuit Breakers in conjunction with Retry and Timeout patterns for a robust approach to error handling.
-
Test and Iterate: Continuously monitor the performance and adjust the Circuit Breaker configuration based on your findings.
-
Use Observability Tools: Integrate tools like Grafana and Prometheus to visualize the performance of your Circuit Breakers.
-
Educate Your Team: Ensure that your team understands Circuit Breakers and their importance to application resilience so they can implement them effectively.
Lessons Learned
Mastering Circuit Breakers in Envoy requires a solid understanding of microservices architecture, resilience patterns, and Envoy's capabilities. By configuring Circuit Breakers effectively, you can vastly improve the robustness and reliability of your services. Remember to monitor their performance, adjust configurations based on real traffic patterns, and share your knowledge with your team.
Additional Resources
- Envoy Proxy Documentation
- Circuit Breaker Pattern - Martin Fowler
- Monitoring Envoy with Prometheus
By following the guidelines discussed in this post, you will be well on your way to mastering Circuit Breakers in Envoy sidecar proxies and enhancing the resilience of your applications. Happy coding!
Checkout our other articles