Troubleshooting Common Issues in Spring Workflow Engines
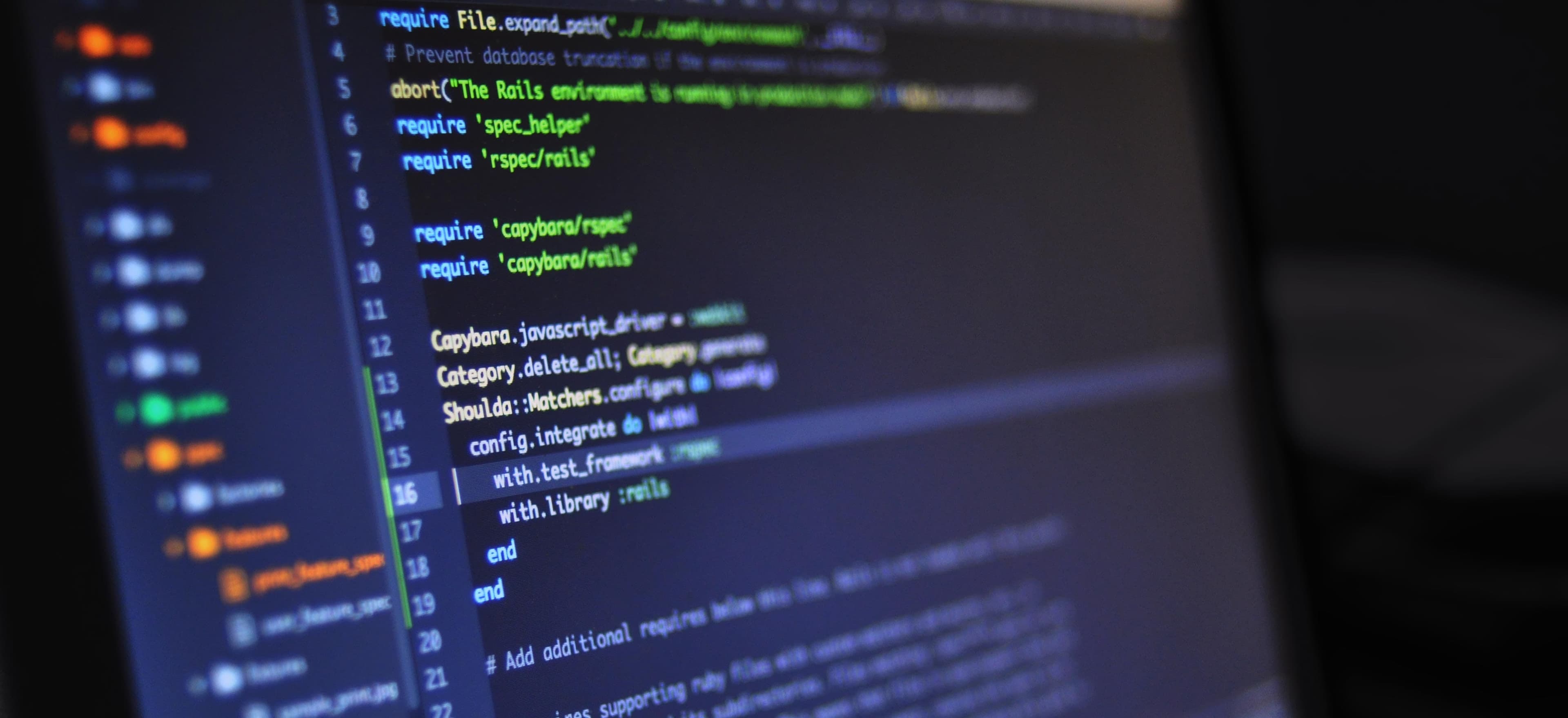
- Published on
Troubleshooting Common Issues in Spring Workflow Engines
Workflow engines are critical components in many modern enterprise systems, automating complex business processes and improving operational efficiency. When using Spring-based workflow engines such as Spring Cloud Data Flow or Spring Web Flow, you may encounter several common issues. This blog post aims to help you troubleshoot these issues effectively.
Understanding Spring Workflow Engines
Spring workflow engines are designed to handle the orchestration of processes, allowing for greater manageability and scalability. Understanding the core concepts behind these engines can significantly aid in troubleshooting.
- Workflows: Sequences of tasks or actions that define an end-to-end process.
- Tasks: Stand-alone pieces of work within the workflow.
- Events: Triggers that initiate task execution (e.g., user inputs, scheduled times).
For a more in-depth understanding of Spring Web Flow, refer to the Spring Web Flow documentation.
Common Issues in Spring Workflow Engines
1. Application Context Issues
One of the most prevalent issues developers face is application context-related problems, often when beans are not properly defined or injected. Misconfigurations can lead to NoSuchBeanDefinitionException
, among other errors.
Example Scenario
You have defined a service bean but forgot to annotate it with @Service
.
@Service
public class MyService {
public void performAction() {
// Implementation here
}
}
Troubleshooting Steps
- Check Annotations: Ensure all beans are properly annotated.
- Correct Packages: Verify that component scanning is set up to include your packages.
- Review Configuration: Double-check your XML or Java configuration files.
2. Task Execution Failures
Sometimes tasks fail to execute due to various reasons, including configuration errors, resource unavailability, or exceptions thrown during execution.
Example Scenario
You have a task that connects to a database, but the database URL is incorrect.
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/invalid_db");
dataSource.setUsername("user");
dataSource.setPassword("password");
return dataSource;
}
Troubleshooting Steps
- Log Exception Stack Traces: Examine the logs for any root cause exceptions related to the task.
- Verify Configuration Values: Ensure that connection details such as the URL, username, and password are correct.
- Check Dependencies: Ensure all required libraries (e.g., JDBC drivers) are included in your build.
3. Workflow State Management
State management issues can result in workflows not progressing as expected. This may happen if there are missing transitions or state definitions.
Example Scenario
You might forget to specify the transitions between states in your workflow definition.
<flow xmlns="http://www.springframework.org/schema/webflow"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/webflow
http://www.springframework.org/schema/webflow/spring-webflow.xsd">
<view-state id="start" view="startView">
<transition on="next" to="end"/>
</view-state>
<end-state id="end" view="endView"/>
</flow>
Troubleshooting Steps
- Review State Configuration: Ensure all states and transitions are correctly defined.
- Test Workflow Path: Manually trigger transitions to verify that the workflow progresses.
- Use Debugging Tools: Leverage debugging tools to monitor state changes in the application.
4. Performance Issues
Workflow engines can sometimes experience performance bottlenecks, especially under heavy loads. This can derail the entire business process.
Example Scenario
Improperly configured thread pools can lead to insufficient resources for task execution.
@Bean
public Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(200);
executor.setThreadNamePrefix("task-");
executor.initialize();
return executor;
}
Troubleshooting Steps
- Monitor Resource Usage: Use tools like VisualVM or JConsole to track CPU and memory consumption.
- Adjust Thread Pool Settings: Tune the thread pool according to the expected load.
- Review Database Connections: Ensure the database can handle the number of concurrent connections required by the application.
5. Integration Issues
When integrating with various systems or services, you may run into communication issues or data format mismatches.
Example Scenario
An HTTP client might misconfigure the request or not handle timeouts well.
@Bean
public RestTemplate restTemplate() {
RestTemplate restTemplate = new RestTemplate();
restTemplate.setRequestFactory(new SimpleClientHttpRequestFactory());
return restTemplate;
}
Troubleshooting Steps
- Validate API Endpoints: Ensure the defined API endpoints are accessible and respond as expected.
- Inspect Request and Response: Use tools like Postman or Fiddler to verify requests and responses.
- Check Timeouts: Make sure your request timeouts are reasonable and not prematurely terminating requests.
Additional Resources
- For more about error handling in Spring workflows, check this guide on Spring Boot Error Handling.
- Dive into asynchronous workflows with the Spring WebFlow Asynchronous Processing.
Final Thoughts
Troubleshooting common issues in Spring workflow engines requires a balanced approach of understanding the system's behavior and applying systematic investigation techniques. By carefully inspecting your application context, task execution, state management, performance, and integration points, you can quickly identify and resolve issues.
Moreover, staying updated with Spring's best practices and developing a good debugging strategy will significantly enhance your efficiency in managing workflow engines. Have any other common issues come up during your development journey? Share your thoughts and experiences in the comments below!