Overcoming Challenges in Spring Batch Restartability
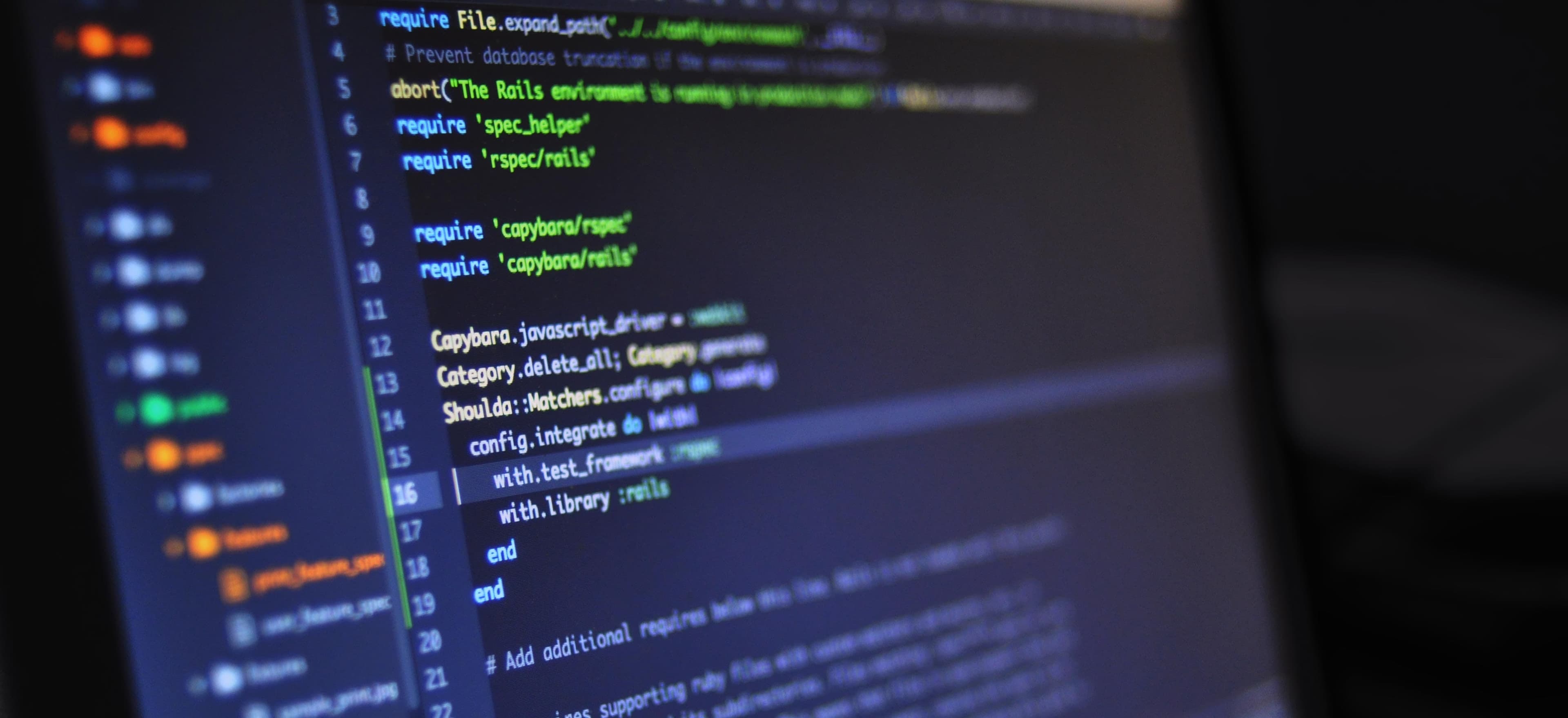
- Published on
Overcoming Challenges in Spring Batch Restartability
Spring Batch is a robust framework that provides reusable functions essential for processing large volumes of data. One powerful feature of Spring Batch is its restartability, which allows jobs to resume from the last successful execution point in case of a failure. Despite its advantages, implementing restartability in Spring Batch can present challenges. In this blog post, we will explore those challenges and provide practical solutions to overcome them.
Understanding Restartability
Restartability is a critical feature in batch processing. In a typical batch job, if an error occurs, the job can be restarted without the need to reprocess the entire dataset. Spring Batch facilitates this with Job Repository, which stores execution context information and allows developers to restart jobs.
Why is Restartability Important?
- Efficiency: Reduces processing time by avoiding re-execution of completed tasks.
- Reliability: Provides a way to recover from failures without losing the progress already made.
- User Experience: Keeps users informed that their process can resume from where it left off.
However, implementing restartability comes with its own set of challenges. Let's delve into some of these challenges and how to solve them.
Common Challenges in Restartability
1. Inconsistent State Handling
When a job fails mid-execution, the system might end up in an inconsistent state. For instance, if the job was halfway done inserting records into a database but crashed, it might leave partial data.
Solution:
Use the chunk-oriented processing in Spring Batch. This divides the data into manageable chunks that can be processed, committed, or rolled back independently.
@Bean
public Step step() {
return stepBuilderFactory.get("step")
.<InputType, OutputType>chunk(10)
.reader(itemReader())
.processor(itemProcessor())
.writer(itemWriter())
.faultTolerant()
.retryLimit(3)
.retry(SomeException.class)
.build();
}
In the above code snippet, the chunk(10)
method ensures that the job processes only 10 items at a time, minimizing the inconsistent state risk. Implementing fault tolerance with retryLimit
allows the job to attempt retries on specific exceptions.
2. Job Parameters and Execution Context
Each job execution in Spring Batch is associated with its specific job parameters and execution context. Inadequate handling of these elements can lead to confusion during restarts.
Solution:
Define parameter management appropriately. Always pass parameters needed for the job execution to the next start. Also, make use of the Execution Context to synchronize or store the state.
public JobParameters createJobParameters(String param) {
Map<String, JobParameter> parameters = new HashMap<>();
parameters.put("myParam", new JobParameter(param));
return new JobParameters(parameters);
}
This snippet details how to create job parameters to manage their states effectively. By including essential parameters, you ensure the job can accurately pick up from where it left off.
3. Data Integrity Issues
Data integrity during restarts is another critical challenge since processing could lead to duplicates or data anomalies if previous records are not correctly recorded.
Solution:
Use a processing marker or a tracking mechanism to monitor completed items:
public class ItemProcessor implements ItemProcessor<InputType, OutputType> {
@Override
public OutputType process(InputType item) throws Exception {
if (isAlreadyProcessed(item)) {
return null; // Skip processing if already done
}
// Process the item
return processedItem;
}
private boolean isAlreadyProcessed(InputType item) {
// Logic to check if the item was already processed
}
}
Here, the isAlreadyProcessed
method checks if an item has already been processed. This prevents duplication and ensures data integrity.
4. Resource Management and Cleanup
Restarting a job without a proper cleanup of resources can result in memory leaks or data corruption. Resources such as connections to databases or files need to be appropriately managed.
Solution:
Ensure the correct lifecycle management of resources. Use appropriate transactional management for database operations.
@Transactional
public void processData() {
// Logic to read, process, and write data
}
Employing the @Transactional
annotation ensures that the database operations can roll back in the event of a failure.
5. Handling External Dependencies
Jobs often rely on external systems, such as file systems or APIs, which may not always represent reliable states. This can introduce various complications.
Solution:
Introduce idempotency in your external interactions to prevent issues caused by unexpected restarts.
public void callExternalService() {
if (!isAlreadyNotified()) {
// Call the external service
notifyExternalService();
}
}
This method ensures that an external service is called only once, even if restarts occur.
Best Practices for Spring Batch Restartability
-
Thorough Testing:
- Always ensure that batch jobs undergo rigorous testing in various scenarios, including failure and recovery.
-
Proper Logging:
- Implement comprehensive logging around job steps. This helps in diagnosing issues during restarts.
-
Regular Backup:
- Maintain regular backups of your job repository to restore from disasters.
-
Monitor Job Execution:
- Use Spring Batch Admin or similar tools to monitor the health of your batch jobs.
-
Consider Data Size:
- For jobs that work with large datasets, consider chunking appropriately to prevent memory issues.
The Bottom Line
Restartability in Spring Batch is an essential feature that enhances the robustness and durability of your batch jobs. By understanding the common challenges and implementing the provided solutions, you can leverage this feature effectively.
For those looking to dive deeper into the concepts of Spring Batch and restartability, consider reading the Spring Batch Reference Documentation and exploring additional resources such as Spring Batch GitHub Repository for more practical examples.
Harness the power of Spring Batch, and ensure your applications handle failures gracefully, processing data reliably without unnecessary rework!
Checkout our other articles