How to Overcome Spring MVC Challenges for Atom Feeds
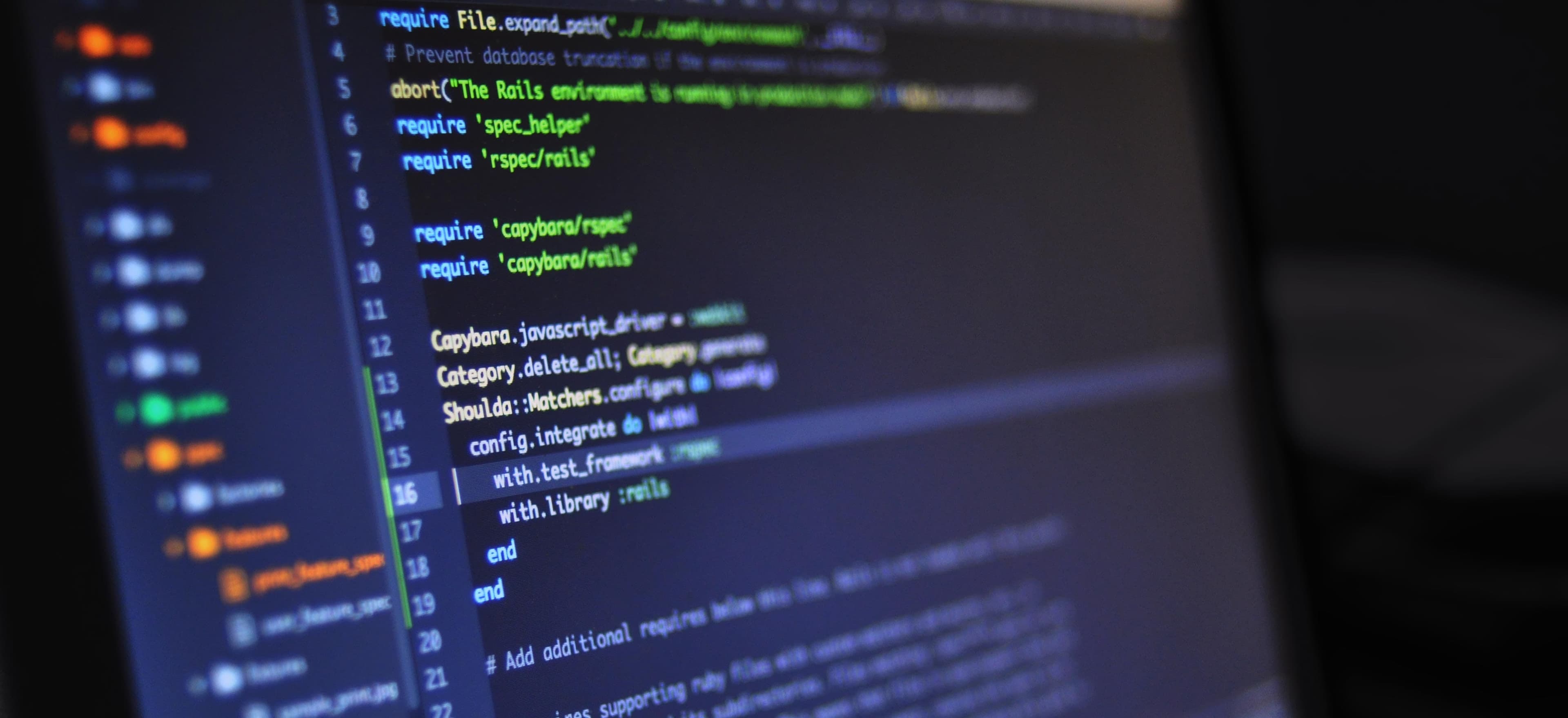
- Published on
How to Overcome Spring MVC Challenges for Atom Feeds
Atom feeds provide a structured way to distribute web content, and they can be quite beneficial for web applications requiring syndication. While Spring MVC is a powerful framework for building web applications in Java, working with Atom feeds can present unique challenges. In this blog post, we will explore these challenges, along with effective strategies to overcome them.
What is Atom Feed?
An Atom feed is an XML-based format that allows users to subscribe to updates from web applications. Similar to RSS, Atom feeds can contain metadata about items, such as titles, links, and publication dates, wrapped in a single XML document.
Advantages of Atom Feeds:
- Standardization: Atom is a standardized format for syndication, making integration with various platforms easier.
- Rich Data: Atom supports various content types and can include additional metadata, enhancing the user experience.
- Well-Structured: Atom’s XML structure allows for clarity and ease of parsing.
Challenges of Implementing Atom Feeds in Spring MVC
Spring MVC offers several conveniences for building web applications, but when it comes to creating Atom feeds, developers often encounter specific challenges. Here are the main ones:
1. Content Negotiation
When your application supports multiple formats (like JSON, XML, etc.), you need effective content negotiation. Spring MVC includes built-in support for content negotiation, but configuring it properly for Atom feeds can be tricky.
2. Serialization of Complex Objects
Converting complex Java objects into the Atom XML format requires careful serialization. Failure to properly serialize could lead to incomplete or incorrect feeds.
3. Handling Conditional GET Requests
Optimizing endpoint performance through caching and handling conditional GET requests properly is crucial. This involves understanding the ETag
and Last-Modified
headers.
4. Understanding Atom Syndication Protocol
Familiarity with the Atom Syndication Protocol is essential. Developers need to ensure they correctly adhere to its specifications when developing feeds.
Overcoming Spring MVC Challenges for Atom Feeds
Let’s dive into some strategies for overcoming these challenges.
1. Implementing Content Negotiation
To effectively support Atom feeds alongside other formats, you can configure Spring MVC's content negotiation feature. By specifying the acceptable media types, you can control how the server responds.
Here’s an example of how to configure content negotiation in your WebMvcConfigurer
:
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void configureContentNegotiation(ContentNegotiationConfigurer configurer) {
configurer.favorPathExtension(false)
.favorParameter(true)
.parameterName("mediaType")
.ignoreAcceptHeader(false)
.useRegisteredExtensionsOnly(false)
.defaultContentType(MediaType.APPLICATION_XML);
}
}
Why this Matters:
By configuring content negotiation this way, you create a robust mechanism that can seamlessly serve different types of outputs based on client preferences. This ensures users receive data in their preferred format while reducing the need for changing application logic.
2. Serializing Complex Objects
Using JAXB (Java Architecture for XML Binding), you can simplify the process of converting Java objects to XML.
Here's how you might define a Java class for an Atom entry:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "entry")
public class AtomEntry {
private String title;
private String link;
private String content;
@XmlElement
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@XmlElement
public String getLink() {
return link;
}
public void setLink(String link) {
this.link = link;
}
@XmlElement
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
Why this Matters:
Using JAXB allows you to focus on your Java objects rather than the complexities of XML formatting. This approach leads to cleaner code and less room for serialization errors.
3. Implementing Conditional GET Requests
To make your Atom feed endpoints more efficient, implement handling for ETag
and Last-Modified
headers. You would want to check if the client already has the most recent version of the feed.
Here's a simple example:
@RequestMapping(value = "/atom", method = RequestMethod.GET)
public ResponseEntity<AtomFeed> getAtomFeed(@RequestHeader(value = "If-None-Match", required = false) String eTag) {
AtomFeed feed = yourFeedService.generateFeed(); // Generate fresh feed
String currentETag = generateETag(feed);
if (currentETag.equals(eTag)) {
return ResponseEntity.status(HttpStatus.NOT_MODIFIED).body(null);
}
return ResponseEntity.ok()
.eTag(currentETag)
.body(feed);
}
Why this Matters:
Implementing conditional requests not only improves your API's performance but also saves bandwidth, as it prevents unnecessary data transfers.
4. Understanding Atom Syndication Protocol
Finally, familiarizing yourself with the Atom Syndication Protocol is essential for creating compliant feeds. Resources like RFC 4287 provide in-depth information about the specifications and should be consulted during implementation.
Bringing It All Togethers
In summary, while integrating Atom feeds into your Spring MVC application comes with its own set of challenges, understanding how to navigate these hurdles can lead to efficient and well-structured feeds. By properly configuring content negotiation, leveraging libraries like JAXB for serialization, implementing efficient request handling for caching, and thoroughly understanding the Atom Syndication Protocol, you can create a robust solution that meets both your and your users’ needs.
With this foundational knowledge, you are now well-equipped to handle Atom feeds in your Spring MVC applications. Moreover, these practices will contribute to cleaner, more maintainable code and ultimately enhance the user experience.
As you delve deeper into Spring MVC, consider exploring more Spring Documentation for additional functionalities that can further boost your applications. Happy coding!
Checkout our other articles