Common Challenges in Configuring Spring Batch on WildFly
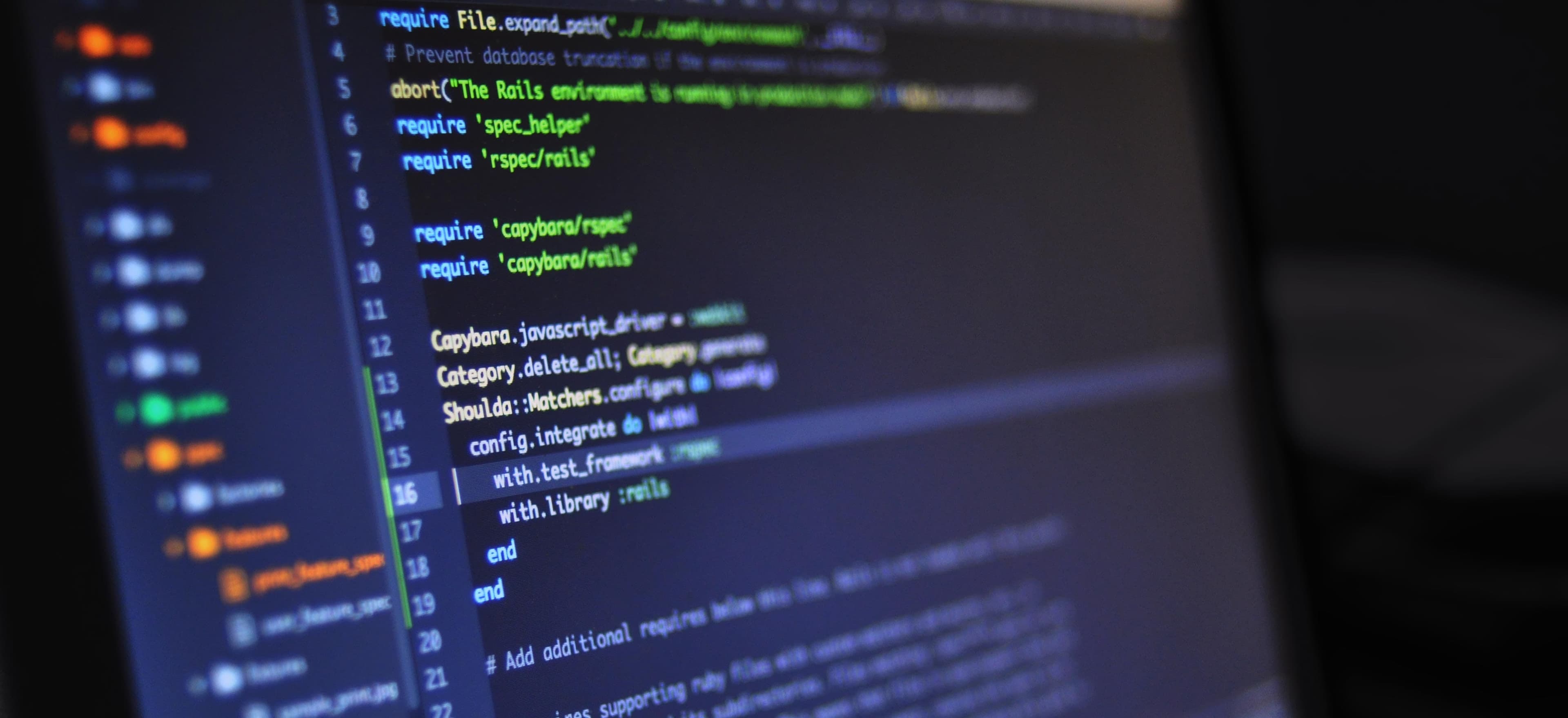
- Published on
Common Challenges in Configuring Spring Batch on WildFly
Spring Batch is a powerful framework designed for batch processing in Java applications. It supports high-volume processing through efficient batch jobs and provides features like transaction management, chunk processing, and skip logic. Deploying Spring Batch in a robust application server like WildFly can be rewarding. However, it does come with its own set of challenges. In this blog post, we'll explore some common issues when configuring Spring Batch on WildFly and provide some guidelines and best practices for overcoming them.
Understanding Spring Batch and WildFly
Spring Batch is part of the Spring Framework, which focuses on the development of batch processing applications. It offers reusable processing components, enabling developers to set up robust batch jobs seamlessly.
WildFly, on the other hand, is a popular open-source application server, originally known as JBoss AS. It provides a lightweight, flexible architecture suitable for deploying Java EE applications.
Integrating the two requires careful configuration to avoid common pitfalls.
Common Challenges
1. Dependency Management
Spring Batch depends on various Spring framework libraries, which can lead to classloading conflicts within WildFly's environment.
Solution
To manage dependencies cleanly, you should package your application as a standalone JAR with all relevant dependencies bundled using a build tool like Maven or Gradle. You can use the Shade plugin for Maven or the Shadow plugin for Gradle. This ensures that the application runs with all its required libraries, avoiding version conflicts.
Here's an example of how you might set up a Maven pom.xml
:
<dependency>
<groupId>org.springframework.batch</groupId>
<artifactId>spring-batch-core</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-batch</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>3.0.0</version>
</dependency>
Using specific versions ensures that your application remains stable.
2. Transaction Management
Spring Batch relies heavily on transactions. WildFly provides its own transaction manager, which can complicate matters when integrating with Spring's transaction management.
Solution
To properly configure transaction management, make sure to define your transaction manager. Here’s how to set it up in your Spring Batch configuration:
@Configuration
@EnableBatchProcessing
public class BatchConfiguration {
@Bean
public PlatformTransactionManager transactionManager(EntityManagerFactory entityManagerFactory) {
return new JpaTransactionManager(entityManagerFactory);
}
}
This code example demonstrates that you can align Spring's transaction management with JPA's EntityManager setup.
3. Setting Up Data Sources
Configuring the data source properly is critical. If based on JPA, it needs to be defined correctly in standalone.xml
or through microprofile-config properties.
Solution
In WildFly, data sources are typically defined in the standalone.xml
file. Here's an example of how to define a datasource:
<datasource jndi-name="java:jboss/datasources/MyDS" pool-name="MyDS">
<connection-url>jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE</connection-url>
<driver>h2</driver>
<security>
<user-name>sa</user-name>
<password>sa</password>
</security>
</datasource>
To access this data source within your Spring Batch configuration, you can use:
@Bean
public DataSource dataSource() {
return new JndiDataSourceLookup().getDataSource("java:jboss/datasources/MyDS");
}
4. Configuration Conflicts
Configuring Spring Batch in a Java EE application server might lead to conflicts, particularly with the default configurations in Spring.
Solution
To avoid configuration conflicts, you can define your custom configurations explicitly using the Spring <context:annotation-config>
or <context:component-scan>
.
<context:annotation-config />
<context:component-scan base-package="com.example.batch" />
With this setup, only the Spring components necessary for your application will be scanned, reducing possible conflicts.
5. Classloading Issues
Classloading can lead to issues where Spring cannot find the necessary classes due to WildFly's deployment structure.
Solution
To address classloading issues, you can adjust the jboss-deployment-structure.xml
. Here's a way to set this up:
<jboss-deployment-structure>
<deployment>
<dependencies>
<module name="javax.api" export="true"/>
<module name="javax.transaction.api" export="true"/>
<module name="org.springframework" export="true"/>
<module name="org.hibernate" export="true"/>
</dependencies>
</deployment>
</jboss-deployment-structure>
This helps WildFly to locate the required classes by defining explicit module dependencies.
6. Performance Tuning
When running batch jobs, performance can be a common challenge, especially under high loads.
Solution
Utilize the chunk-oriented processing that Spring Batch provides. In your Job configuration, you can set the chunk size, which determines how many items are processed per transaction. A well-defined chunk size can significantly improve performance.
@Bean
public Job job(JobBuilderFactory jobBuilderFactory, Step step) {
return jobBuilderFactory.get("job")
.incrementer(new RunIdIncrementer())
.flow(step)
.end()
.build();
}
@Bean
public Step step(StepBuilderFactory stepBuilderFactory) {
return stepBuilderFactory.get("step")
.<InputType, OutputType>chunk(10) // Process 10 records at a time
.reader(reader())
.processor(processor())
.writer(writer())
.build();
}
Summary
Configuring Spring Batch on WildFly can be complex due to numerous factors, including dependency management, transaction handling, classloading issues, and performance optimization. By following a structured approach and using best practices as outlined above, you can effectively overcome these challenges.
For a deeper examination of Spring Batch, you may check out the official Spring documentation here, and for details about WildFly configuration, refer to the documentation here.
By being aware of these challenges and employing the solutions described, you can smoothly deploy a Spring Batch application on WildFly, ensuring robust and efficient batch processing capabilities. Happy coding!
Checkout our other articles