Translating C to Java: Common Pitfalls to Avoid
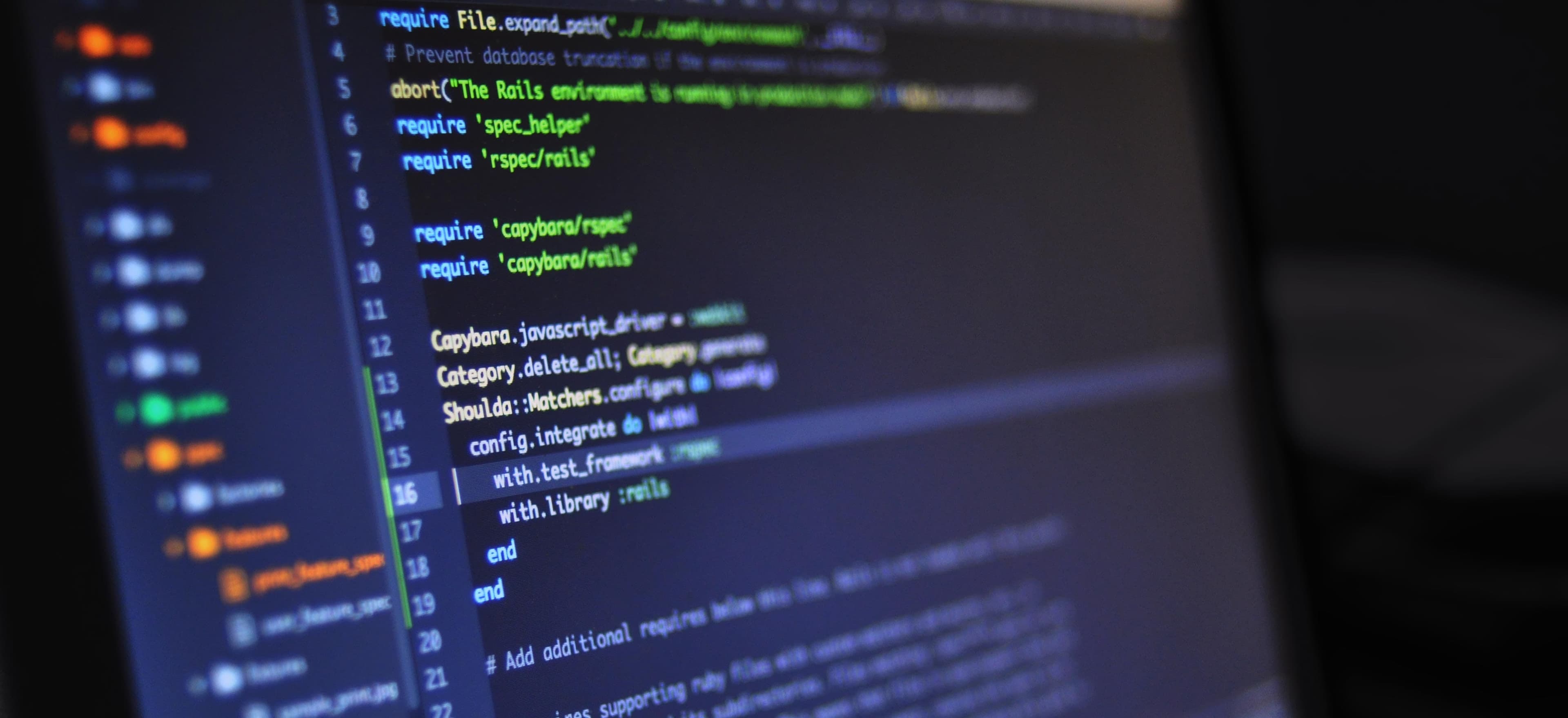
- Published on
Translating C to Java: Common Pitfalls to Avoid
Translating code from C to Java can be a valuable skill, especially for developers seeking to leverage Java's rich ecosystem and its resource management capabilities. However, the two languages differ significantly in structure, design philosophy, and memory management. This blog post will focus on common pitfalls when translating C code to Java, helping you navigate the complexities of this process successfully.
Understanding the Fundamental Differences
Before diving into the specifics of pitfalls, it's essential to understand the fundamental differences between C and Java. Here are a few core distinctions:
- Memory Management: C uses manual memory management (malloc/free), while Java employs automatic garbage collection.
- Pointers vs References: C uses pointers to manipulate memory directly, whereas Java uses references, abstracting memory management.
- Data Types and Structures: C has primitive types and user-defined structures, whereas Java has classes and interfaces for object-oriented programming.
With these differences in mind, let's look at specific pitfalls.
Pitfall 1: Misunderstanding Memory Management
In C, developers are responsible for allocating and freeing memory, which can lead to memory leaks or dangling pointers if not handled properly. In contrast, Java's garbage collection eases this burden by automatically reclaiming memory that is no longer in use.
C Code Example
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr = (int*)malloc(10 * sizeof(int));
// Use arr
free(arr); // Remember to free the memory
return 0;
}
Java Equivalent
public class Main {
public static void main(String[] args) {
int[] arr = new int[10]; // Automatic memory management
// Use arr
}
}
Commentary
The critical aspect here is recognizing that there is no need to manually free memory in Java. However, be careful with large objects; they can still lead to out-of-memory errors if not controlled.
Pitfall 2: Using Primitive Types Inappropriately
C heavily relies on primitive data types, which can lead to cluttered code when translating to Java. While Java offers similar primitive types (such as int and float), it also provides wrapper classes (like Integer and Float).
C Code Example
#include <stdio.h>
int main() {
double value = 32.5;
printf("Value is: %f\n", value);
return 0;
}
Java Equivalent
public class Main {
public static void main(String[] args) {
double value = 32.5; // Java primitive
System.out.println("Value is: " + value);
}
}
Commentary
Here, the value remains a primitive type in Java. However, in cases where you require nullability or want to leverage collections, prefer wrapper classes:
Integer integerValue = null; // Nullable wrapper
Pitfall 3: Poor Exception Handling
In C, error handling often revolves around return codes. Java enhances error handling with exceptions, making it cleaner and more robust.
C Code Example
#include <stdio.h>
int divide(int a, int b) {
if (b == 0) {
return -1; // error code for division by zero
}
return a / b;
}
int main() {
int result = divide(10, 0);
if (result == -1) {
printf("Error: Division by zero.\n");
} else {
printf("Result is: %d\n", result);
}
return 0;
}
Java Equivalent
public class Main {
public static int divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("Division by zero.");
}
return a / b;
}
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result is: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Commentary
Using exceptions improves clarity and separates error handling from business logic. Always use try-catch blocks in Java where applicable to manage exceptional cases efficiently.
Pitfall 4: Confusing Interface and Implementation
In C, files can have functions defined in one file (header files) and implementations in another. In Java, the interface and implementation are often confined in the same class or in defined interfaces.
C Code Example
// header.h
void func();
// implementation.c
#include "header.h"
void func() {
// some logic
}
Java Equivalent
public interface MyInterface {
void func();
}
public class MyClass implements MyInterface {
@Override
public void func() {
// some logic
}
}
Commentary
In Java, it's considered good practice to use interfaces to define behavior, allowing for easier code reuse and testing. Make sure to take advantage of this feature for cleaner architecture.
Pitfall 5: Neglecting String Handling Differences
String handling in C can be risky due to null-terminated arrays, while Java provides a robust String
class that makes string manipulation straightforward and safer.
C Code Example
#include <stdio.h>
#include <string.h>
int main() {
char str[20] = "Hello";
strcat(str, " World");
printf("%s\n", str);
return 0;
}
Java Equivalent
public class Main {
public static void main(String[] args) {
String str = "Hello";
str += " World"; // String concatenation in Java
System.out.println(str);
}
}
Commentary
Java's String
class is immutable, meaning each time you modify a string, you're creating a new object. For more performance-sensitive scenarios, consider using the StringBuilder
class:
StringBuilder sb = new StringBuilder("Hello");
sb.append(" World");
System.out.println(sb.toString());
Wrapping Up
Translating C to Java is not without its challenges. By understanding these common pitfalls, developers can make the transition smoother and more efficient. Remember to embrace Java's object-oriented features, leverage its robust exception handling, and respect memory management differences to produce clean and efficient code.
For additional context on Java programming or object-oriented concepts, consider visiting Oracle’s Java Tutorials or GeeksforGeeks on Java.
By staying aware of these challenges, you'll be well-equipped to translate your C code into elegant Java solutions. Stay curious and code well!
Checkout our other articles