Unlocking Database Architecture: The Importance of Table Design
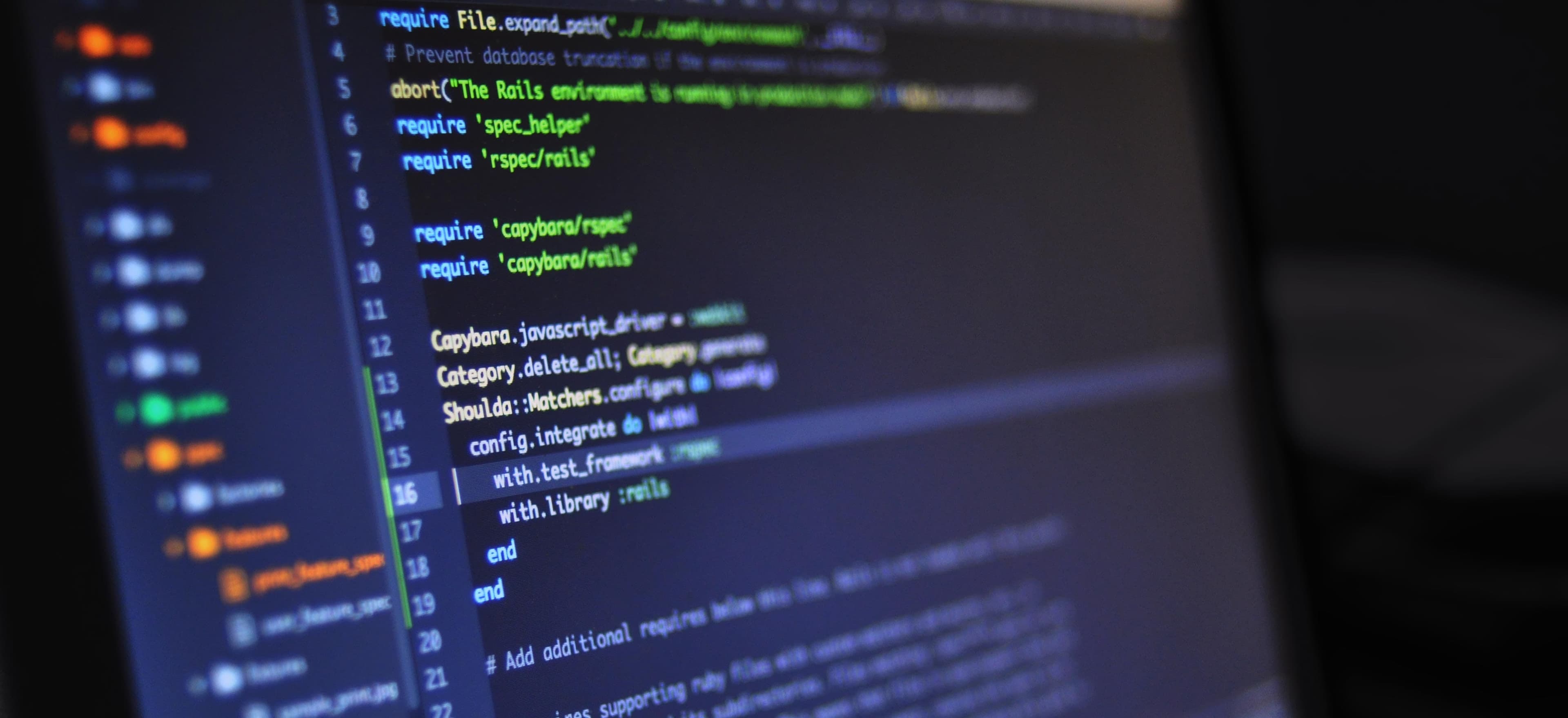
- Published on
Unlocking Database Architecture: The Importance of Table Design
Database architecture is an integral part of software development, playing a critical role in how applications manage, store, and retrieve data. Among the foundational elements of this architecture is the table design, which can directly impact both performance and scalability. In this blog post, we will dive deep into the nuances of effective database table design, its importance, best practices, and examples to illustrate these concepts.
Why Table Design Matters
Performance: A well-structured table significantly optimizes query response times. Poorly designed tables can lead to excessive loading times and slow application performance.
Scalability: Good design practices make it easier to scale your database as your application grows. You'll face fewer complications when the time comes to add new features or optimize existing ones.
Data Integrity: Proper relationships and constraints promote data integrity, ensuring that the information remains correct, accurate, and reliable.
Maintenance: An organized design simplifies database maintenance tasks. It helps developers understand the schema quickly, thereby reducing onboarding time and improving team collaboration.
Here are some key principles to consider when designing your database tables.
Principles of Effective Table Design
1. Understand Your Data Model
Before diving into table creation, take time to understand the nature of your data. This includes understanding the relationships between different data entities. Are they one-to-one, one-to-many, or many-to-many? A clear data model allows you to design better database tables.
Example:
Suppose you are designing a database for a library management system. You have entities like Books
, Authors
, and Members
. Before implementing these as tables, sketch out their relationships.
- Each book can have multiple authors (many-to-many).
- Each member can borrow multiple books (one-to-many).
Illustrating these relationships can clarify how to structure your tables.
2. Use Appropriate Data Types
Data types need to be accurately defined for each column in a table. Using the wrong type can lead to performance issues and incorrect data handling.
CREATE TABLE Books (
BookID INT PRIMARY KEY,
Title VARCHAR(255) NOT NULL,
PublishDate DATE,
Price DECIMAL(10, 2)
);
In the above snippet:
INT
is used forBookID
since it’s an identifier.VARCHAR(255)
is suitable for string values like the title, allowing for flexibility.DATE
is employed for storing dates, whileDECIMAL(10, 2)
ensures that prices are accurate.
3. Normalization
Normalization is the process of organizing your tables to minimize data redundancy. This often involves dividing a larger table into smaller ones and defining relationships between them.
Example of Normalization
Consider a scenario where you might have a table BorrowedBooks
that includes all member and book data together.
CREATE TABLE BorrowedBooks (
MemberID INT,
BookID INT,
BorrowedDate DATE,
MemberName VARCHAR(255),
BookTitle VARCHAR(255)
);
In this design, you store information redundantly. Instead, normalize it:
CREATE TABLE Members (
MemberID INT PRIMARY KEY,
MemberName VARCHAR(255)
);
CREATE TABLE Books (
BookID INT PRIMARY KEY,
BookTitle VARCHAR(255)
);
CREATE TABLE BorrowedBooks (
MemberID INT,
BookID INT,
BorrowedDate DATE,
FOREIGN KEY (MemberID) REFERENCES Members(MemberID),
FOREIGN KEY (BookID) REFERENCES Books(BookID)
);
The separation reduces redundancy and makes your data structure more efficient.
4. Define Primary and Foreign Keys
A primary key uniquely identifies each record in a table. Foreign keys create a link between two tables. The correct implementation of these keys is fundamental to ensuring data integrity and effective data relationships.
CREATE TABLE Authors (
AuthorID INT PRIMARY KEY,
Name VARCHAR(255) NOT NULL
);
CREATE TABLE Books (
BookID INT PRIMARY KEY,
Title VARCHAR(255) NOT NULL,
AuthorID INT,
FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID)
);
In this example, AuthorID
is a foreign key in Books
, linking it to the Authors
table. This clearly defines relationships, ensuring that every book is associated with a valid author.
5. Use Indexing Wisely
Indexes can dramatically improve query performance but can also consume additional disk space and slow down data modification operations. Use indexing judiciously on columns that are frequently searched against or involved in join operations.
CREATE INDEX idx_books_title ON Books(Title);
Here, an index is created for the Title
column, which can make searching and filtering by book titles quicker.
6. Plan for Future Changes
Database design is not just about current requirements; it’s also about future scalability and changes in the application. Leave room for addition without a complete redesign.
Example of Planning for Change
If you anticipate adding book genres in the future, you might want to initially create a Genres
table:
CREATE TABLE Genres (
GenreID INT PRIMARY KEY,
GenreName VARCHAR(100) NOT NULL
);
ALTER TABLE Books ADD GenreID INT,
FOREIGN KEY (GenreID) REFERENCES Genres(GenreID);
This keeps your design flexible, enabling changes or expansions down the line without significant refactoring.
Key Takeaways: Investing in Table Design Pays Off
In conclusion, effective table design is a cornerstone of robust database architecture. It impacts performance, scalability, maintenance, and data integrity. By understanding your data model, employing appropriate data types, normalizing your data, defining keys, indexing wisely, and planning for future changes, you can create a database structure that not only meets current needs but is also flexible for future modifications.
For a more in-depth exploration of relational databases and table design, visit SQL Database Basics or check out Database Normalization.
Invest the time in getting your table design right, and reap the benefits of greater efficiency and maintainability in your applications. Remember, a strong foundation in your database architecture paves the way for future success.