Common Pitfalls When Debugging Codename One Chat Apps
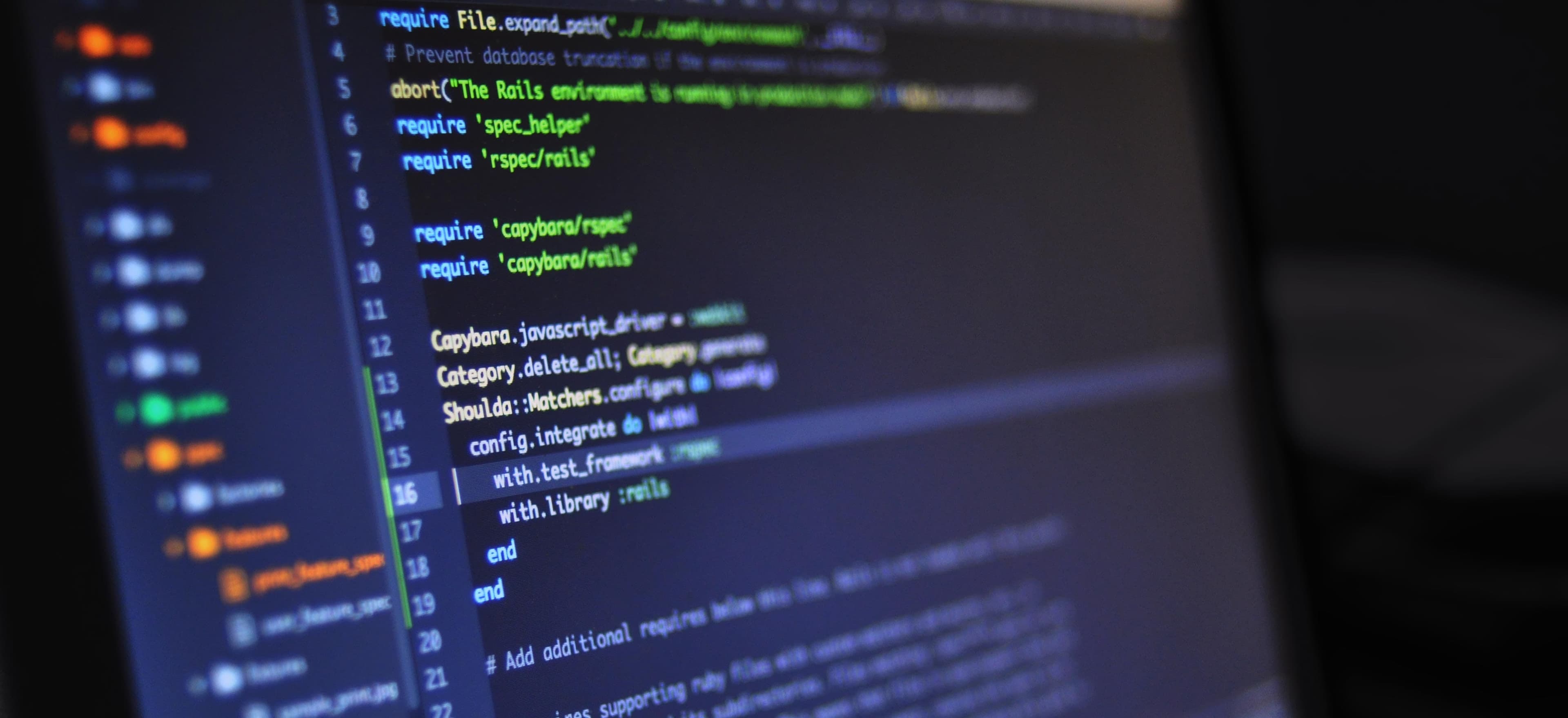
- Published on
Common Pitfalls When Debugging Codename One Chat Apps
Debugging can be as challenging as writing code itself, especially when developing chat applications using Codename One. This cross-platform framework allows developers to create mobile applications quickly, but it also introduces some complexities specific to real-time communication features. In this blog post, we’ll explore the common pitfalls you might encounter while debugging chat apps built with Codename One and provide strategies to overcome them.
Understanding Codename One
Before diving into the intricacies of debugging, let's take a moment to understand what Codename One is. Codename One is a framework for building cross-platform mobile applications that can run on iOS, Android, and various other platforms using a single codebase. It allows developers to use Java to write their apps, making it a popular choice for many.
Codename One Official Website provides extensive documentation and resources, which can be invaluable during your development journey.
Common Pitfalls in Debugging Chat Apps
1. Network Issues
Problem: Chat applications usually rely on a constant network connection. Interruptions or latency can lead to messages not being sent or received promptly, resulting in a poor user experience.
Solution: Implement proper error handling and use retry mechanisms. Utilize Codename One’s networking capabilities to check for network availability before making requests. Here’s a code snippet that checks for a network connection:
if (Display.getInstance().getCurrent().getForm().isScrollableY()) {
if (!Display.getInstance().isAlive()) {
Dialog.show("Network Error", "Please check your internet connection.", "OK", null);
} else {
// proceed with sending the message
}
}
Why? This allows the app to notify users of connectivity issues before they encounter failures, improving user experience.
2. State Management Challenges
Problem: Managing the state of chat messages (sent, received, and read) can be complex, particularly with multiple users.
Solution: Keep your state management simple and maintain a clear flow. Using a Singleton pattern for your chat manager can simplify message state management. Here’s an example:
public class ChatManager {
private static ChatManager instance;
private List<Message> messages;
private ChatManager() {
messages = new ArrayList<>();
}
public static ChatManager getInstance() {
if (instance == null) {
instance = new ChatManager();
}
return instance;
}
public void addMessage(Message message) {
messages.add(message);
// Logic to update UI components if necessary
}
public List<Message> getMessages() {
return messages;
}
}
Why? By using the Singleton pattern, you ensure that the message list is centralized, reducing errors with inconsistent states.
3. Debugging Asynchronous Code
Problem: Asynchronous operations such as network requests and callbacks can introduce timing issues or lead to unexpected behavior.
Solution: Use logging strategically. Codename One provides a logging module that can help trace issues in asynchronous calls. Consider logging important events, especially in callbacks. Here’s a brief example:
NetworkManager.getInstance().addErrorListener(new ActionListener<ErrorEvent>() {
public void actionPerformed(ErrorEvent evt) {
Log.p("Network error occurred: " + evt.getErrorMessage());
}
});
Why? This allows you to capture and troubleshoot errors effectively as they occur, which is crucial for asynchronous operations.
4. User Interface (UI) Feedback
Problem: Ineffective UI feedback can mislead users during interactions, especially in chat apps. Feedback becomes essential when sending messages.
Solution: Ensure that users receive immediate visual confirmation. When a message is sent, the user should see feedback indicating success or failure. Here's an example snippet:
Button sendButton = new Button("Send");
sendButton.addActionListener(evt -> {
sendMessage(messageTextField.getText());
Dialog.show("Message Sent", "Your message has been sent!", "OK", null);
});
Why? Providing instant feedback helps maintain user engagement and preserves trust in the application’s functionality.
5. Testing on Multiple Devices
Problem: Different devices may handle Codename One apps differently, leading to issues that only appear on specific platforms.
Solution: Always test your application on multiple devices and in various environments. Use Codename One's simulator to emulate different platforms, but also conduct real-device testing. This approach uncovers UI inconsistencies and functional discrepancies.
6. Concurrency Issues
Problem: When multiple users are interacting with the app concurrently, bugs can arise from missing synchronization.
Solution: Make use of Codename One’s built-in thread management to handle concurrency effectively. Always execute UI updates on the Event Dispatch Thread. Here’s an example:
Display.getInstance().callSerially(() -> {
// Code that updates the UI
});
Why? By ensuring UI updates are made on the correct thread, you avoid potential conflicts and crashes.
7. Message Delivery and Acknowledgments
Problem: Ensuring messages are delivered and received correctly can be tricky. Many developers overlook implementing acknowledgment mechanisms, leading to confusion about whether messages were received.
Solution: Implement a simple acknowledgment system. When a message is sent, wait for an acknowledgement response from the server before updating the local state. An example:
public void sendMessage(Message message) {
// Code to send the message to the server
sendToServer(message);
// Wait for acknowledgment
addMessageSentListener((msg) -> {
if (msg.isAck()) {
Log.p("Message delivered successfully");
}
});
}
Why? Acknowledgments provide clarity about the state of messages, improving the chat application's reliability.
Lessons Learned
Debugging chat apps in Codename One can be filled with challenges, but by being aware of these common pitfalls and implementing the suggested strategies, you can significantly improve your application's stability and user experience.
Emphasize thorough testing, effective error handling, and maintain simplicity in state management. For more advanced Codename One topics, consider exploring Codename One's Documentation for best practices that could enhance your code quality.
By addressing these issues proactively, you will not only become a more efficient developer but also deliver a better-quality product to your users. Happy coding!