Overcoming Latency Issues in Clustered Idempotent Consumers
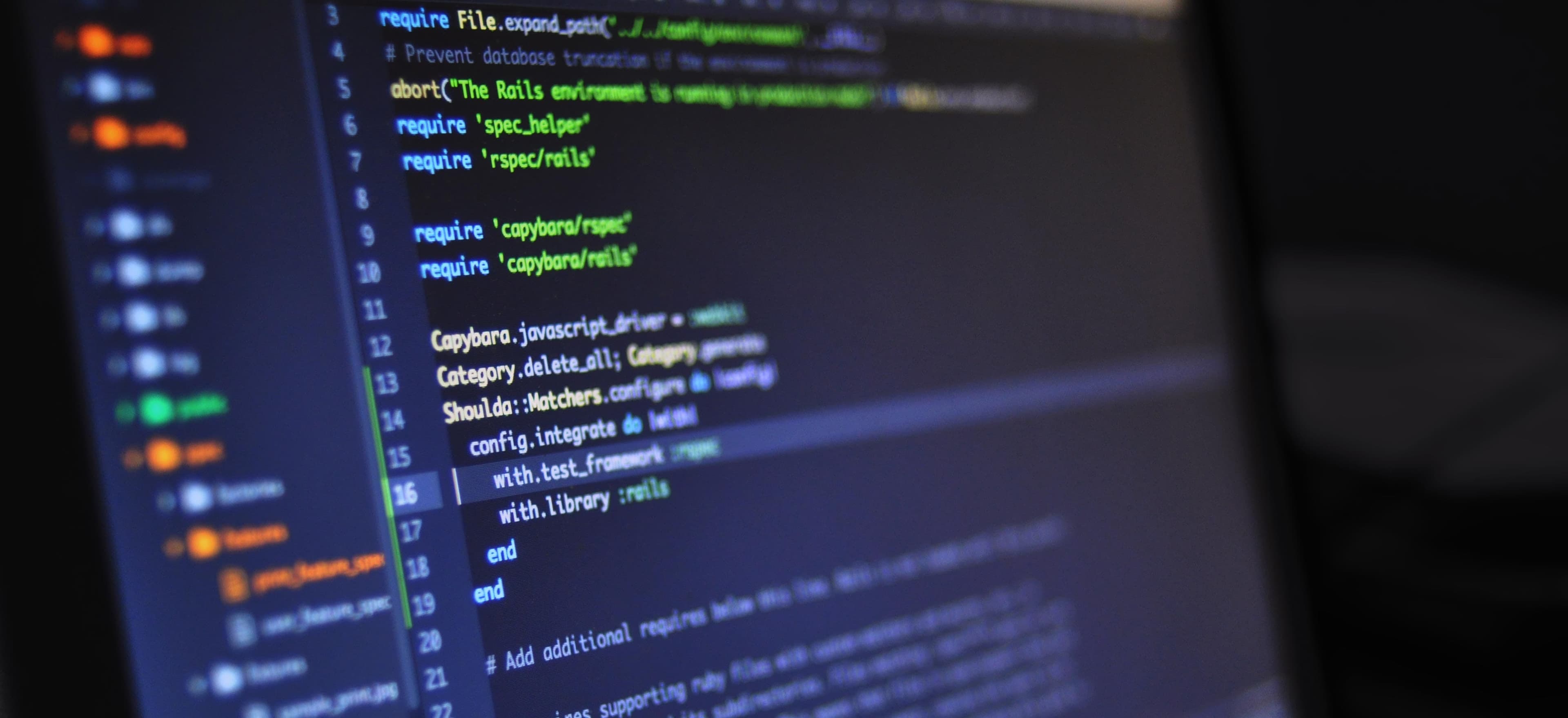
- Published on
Overcoming Latency Issues in Clustered Idempotent Consumers
In modern distributed systems, the concept of idempotency is crucial for ensuring reliable and consistent operations. Specifically in message-driven architectures, where consumers may be clustered for load balancing, maintaining idempotency can become challenging, especially in the face of latency issues. This blog post delves into the intricacies of clustered idempotent consumers, explores common latency challenges, and discusses effective strategies to mitigate these issues.
Understanding Idempotency
Idempotency is a property that ensures an operation can be performed multiple times without changing the outcome beyond the initial application. For example, if you send a request to process a payment, doing it once should yield the same result as doing it multiple times – no additional charges should be incurred.
Why Idempotency Matters in Distributed Systems
In distributed environments, messages may be lost or duplicated due to network issues, making it critical to ensure that consumers can handle repeated messages without adverse effects. Idempotency helps ensure that systems remain reliable even when users face unexpected outages or interruptions.
The Latency Challenge
When dealing with clustered idempotent consumers, latency can pose serious threats to system performance and user experience. Common latency issues include:
- Network Delays: The time taken for messages to travel across the network can introduce noticeable delays.
- Load Distribution: An uneven distribution of tasks among consumers can lead to bottlenecks.
- Database Performance: Slow database access can obstruct quick response times, impacting overall consumer readiness.
Measuring Latency
To address latency issues effectively, we need to measure them. Simple performance testing can help pin down bottlenecks and compliance to service level agreements (SLAs). Tools such as Apache JMeter or Gatling can be useful here.
Strategies to Overcome Latency Issues
Here are several strategies to minimize latency in clustered idempotent consumers:
1. Optimize Message Broker Configuration
Choosing the right message broker can significantly affect your system's performance. For instance, using Apache Kafka or RabbitMQ with appropriate configurations can enhance message throughput and reduce latency.
- For Kafka, consider tweaking the
batch.size
andlinger.ms
parameters to optimize how messages are sent to consumers.
Here is a simple configuration snippet:
# Kafka Producer Configuration
batch.size=16384
linger.ms=5
Why This Matters
Adjusting these parameters allows your consumers to process messages more efficiently, allowing for higher throughput without sacrificing latency.
2. Implement Asynchronous Processing
Synchronous processing can slow down operations, particularly in high-latency scenarios. By employing asynchronous processing, consumers can handle messages independently and return responses more quickly.
Example
Imagine you have a service that makes external API calls. Instead of waiting for a response synchronously, you can perform this task asynchronously:
import java.util.concurrent.CompletableFuture;
public class ExternalServiceCaller {
public CompletableFuture<String> callApiAsync(String endpoint) {
return CompletableFuture.supplyAsync(() -> {
// Simulate an API call that takes time
return makeHttpRequest(endpoint);
});
}
private String makeHttpRequest(String endpoint) {
// Simulated delay
try {
Thread.sleep(2000); // Simulate network delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Response from " + endpoint;
}
}
Why This Matters
By using asynchronous processing, you free up consumer resources and significantly reduce response times.
3. Adopt Client-side Throttling
Throttling is an effective mechanism to manage load and mitigate latency issues. Implementing client-side throttling appropriately can prevent overwhelming your consumers with too many simultaneous requests.
Example
You might utilize a semaphore to limit the number of concurrent requests:
import java.util.concurrent.Semaphore;
public class ThrottledConsumer {
private final Semaphore semaphore;
public ThrottledConsumer(int limit) {
this.semaphore = new Semaphore(limit);
}
public void consume() {
try {
semaphore.acquire();
// Process your message
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
semaphore.release();
}
}
}
Why This Matters
With throttling, you can control the flow of messages and ensure that your consumers are not overwhelmed, thus maintaining a steady processing rhythm.
4. Utilize Caching
Caching solutions can significantly reduce the time taken to retrieve frequently accessed data, thereby lowering latency. By utilizing in-memory caching with solutions like Redis or Memcached, your application can quickly access the necessary information without hitting the database repeatedly.
Example
import redis.clients.jedis.Jedis;
public class CacheService {
private Jedis jedis;
public CacheService() {
jedis = new Jedis("localhost");
}
public String getCachedData(String key) {
return jedis.get(key);
}
public void cacheData(String key, String value) {
jedis.set(key, value);
}
}
Why This Matters
Using caching minimizes database calls, improving response times for consumers by quickly serving up needed data.
5. Monitor and Scale Dynamically
Finally, monitoring the performance of your clustered consumers is crucial. Tools like Prometheus or Grafana can assist in visualizing data and making informed decisions about scaling your consumers based on usage patterns.
Learn more about monitoring tools here.
A Final Look
Latency issues in clustered idempotent consumers can disrupt business processes and degrade user experiences. However, by understanding these latency challenges and applying optimized strategies—including asynchronous processing, caching, throttling, and effective monitoring—you can enhance your system's performance and maintain idempotency across your architecture.
Is your system experiencing latency issues? Consider implementing these strategies to transform your consumer architecture into a robust and efficient framework. The road to a latency-free, idempotent consumer may not be straightforward, but the rewards are well worth it.
For further reading on distributed systems and idempotency, check out this detailed guide on building reliable systems.
Additional Resources
Feel free to share your thoughts or questions on managing latency in distributed systems!