Balancing Code Quality and Security: A Practical Guide
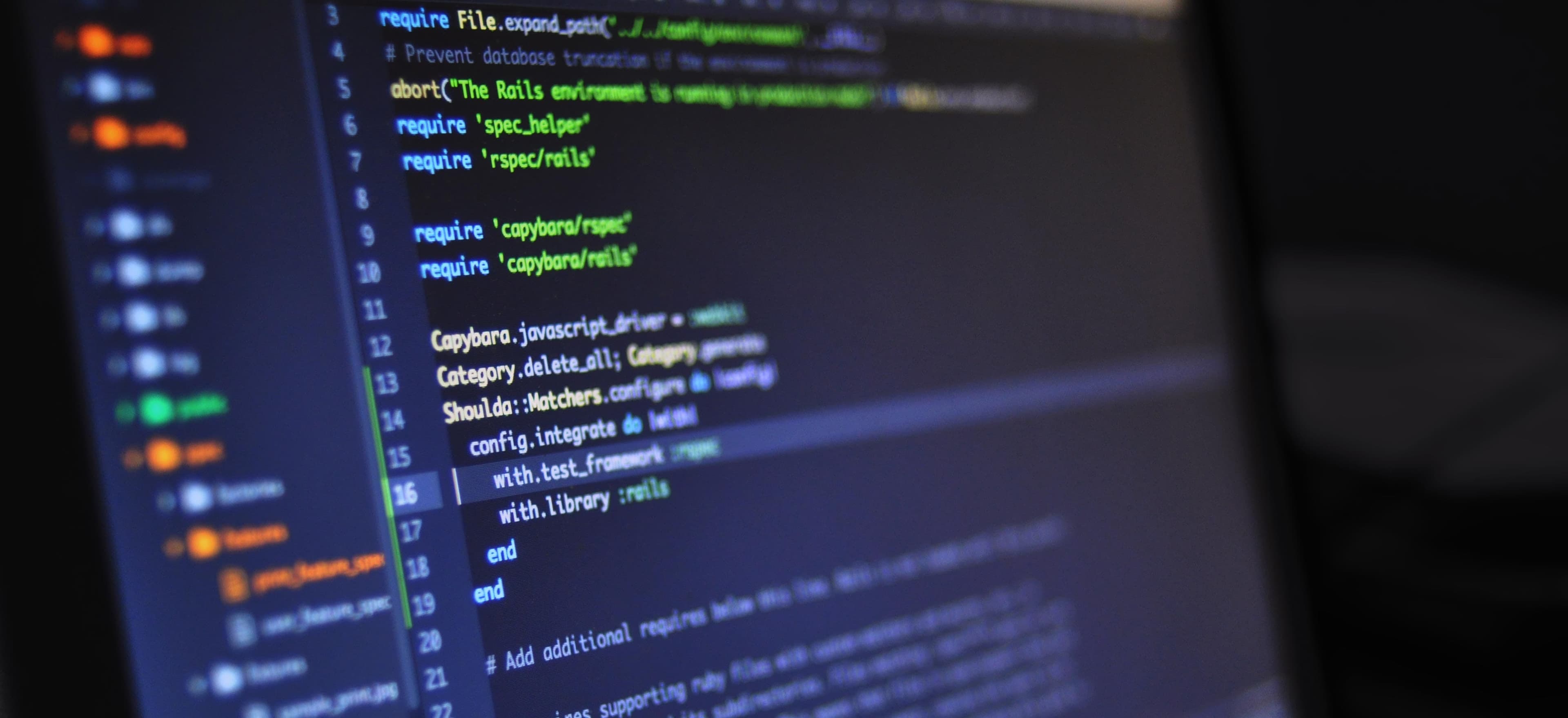
- Published on
Balancing Code Quality and Security: A Practical Guide
In today's software development landscape, the importance of both code quality and security cannot be overstated. As applications become increasingly complex and interwoven with sensitive data, the need for robust, secure code is paramount. This blog post explores strategies for balancing code quality and security and provides practical insights for developers looking to ensure that both aspects are adequately addressed.
What Is Code Quality?
Code quality refers to the attributes of code that make it easier to read, maintain, and understand. Good code quality is characterized by:
- Clarity: The code is easy to read and understand.
- Maintainability: The code can be easily updated and modified.
- Performance: The code runs efficiently under various conditions.
- Testability: The code can be easily tested to ensure it functions as intended.
Why Security Matters
Security, on the other hand, focuses on protecting an application from malicious attacks and vulnerabilities. Poorly written code can lead to security flaws that expose sensitive information or allow unauthorized access. Security practices include:
- Input validation: Ensuring that all user-supplied input is validated before processing.
- Authentication and authorization: Implementing strict access controls.
- Encryption: Protecting sensitive data both in transit and at rest.
The Intersection of Code Quality and Security
At first glance, code quality and security might seem like separate concerns. However, there are numerous overlaps. High-quality code tends to be more secure because:
- It is easier to understand, making it simpler to spot vulnerabilities.
- It is easier to maintain, allowing for faster updates to security patches.
- It often adheres to best practices that reduce the risk of introducing security flaws.
Strategies for Balancing Code Quality and Security
1. Embrace Coding Standards
Establishing coding standards is essential for maintaining both code quality and security. Standards should enforce best practices in coding, such as naming conventions, code organization, and documentation. By adhering to these standards, all team members can work towards a common goal, resulting in cleaner and more secure code.
Code Example: Java Naming Conventions
public class UserAccount {
private String username;
private String password;
public UserAccount(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
The UserAccount
class uses camelCase for variable names and PascalCase for the class name, adhering to Java naming conventions. Following these conventions contributes to better readability, making the code easier to review for potential security issues.
2. Conduct Code Reviews
Regular code reviews are crucial in identifying potential vulnerabilities and ensuring that coding standards are being followed. During code reviews, developers can discuss the logic behind the code, and reviewers can look out for security issues and errors. A robust review process encourages collaboration and shared knowledge among team members.
3. Use Static Code Analysis Tools
Static code analysis tools examine source code before it is run. These tools help identify potential security vulnerabilities, code smells, and deviations from coding standards. By integrating these tools into your Continuous Integration/Continuous Deployment (CI/CD) pipelines, you can automate the detection of issues early in the development process.
Example: SonarQube Configuration
To set up SonarQube for static analysis, you would typically include it as part of your build process:
<plugin>
<groupId>org.sonarsource.scanner.maven</groupId>
<artifactId>sonar-maven-plugin</artifactId>
<version>3.9.0.2155</version>
</plugin>
By running SonarQube as part of your build, you can get a report on code quality and security vulnerabilities. This allows developers to address issues before release.
4. Prioritize Security Training
Developers must be equipped with the knowledge of secure coding practices. Regular training sessions for your team on security topics like the OWASP Top Ten can reinforce critical security concepts. Understanding common vulnerabilities will promote better coding practices, contributing to both code quality and security.
5. Implement Unit and Integration Testing
Testing is a pivotal part of software development. Unit tests validate the functionality of small parts of the code, while integration tests ensure that components work together as expected. Testing should also include security-focused tests.
Code Example: Unit Testing in JUnit
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserAccountTest {
@Test
public void testGetUsername() {
UserAccount user = new UserAccount("testUser", "password123");
assertEquals("testUser", user.getUsername());
}
@Test
public void testGetPassword() {
UserAccount user = new UserAccount("testUser", "password123");
assertEquals("password123", user.getPassword());
}
}
These unit tests ensure that the UserAccount
class functions as intended. Regular testing confirms both the integrity and security of the application.
6. Adopt a Shift-Left Approach
Shifting left means incorporating security practices earlier in the software development lifecycle (SDLC). This proactive approach helps catch security issues during the early stages of development rather than after deployment. By integrating security into the design and coding phases, you can minimize technical debt related to security vulnerabilities.
The Last Word
Balancing code quality and security is an ongoing process that requires attention, effort, and collaboration among development teams. By establishing coding standards, prioritizing security training, utilizing static analysis tools, and implementing comprehensive testing strategies, you can create a culture that values both quality and security. More than just a technical requirement, achieving this balance is essential in building reliable, secure applications that inspire confidence among users and stakeholders.
For more resources on coding standards and security practices, explore:
- The importance of code quality
- OWASP Security Resources
- Effective Java by Joshua Bloch
By taking these steps and integrating security into the core of your development processes, you will foster an environment where quality and security coexist harmoniously, ultimately leading to more resilient software applications.