Common Java Cryptography Mistakes to Avoid
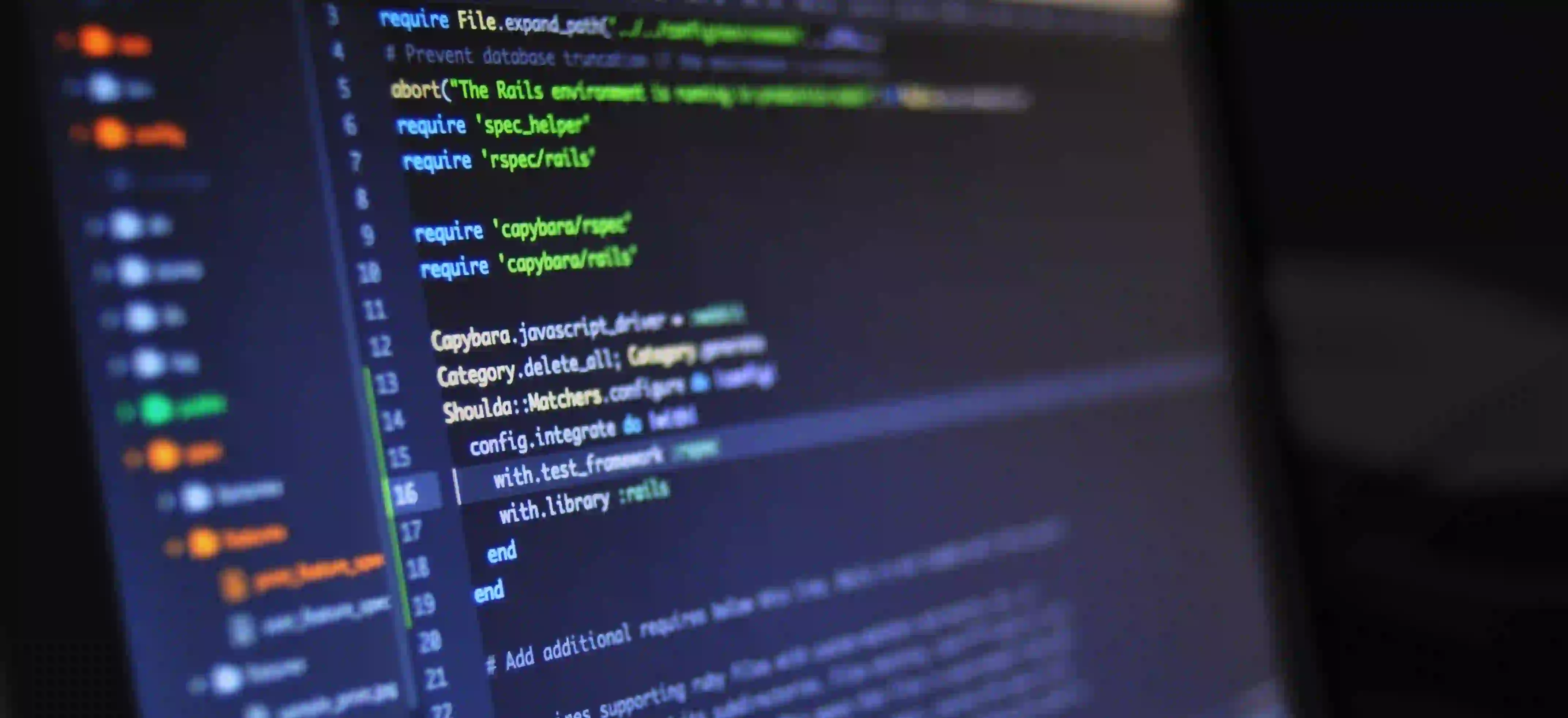
Common Java Cryptography Mistakes to Avoid
Cryptography is a critical component in the arsenal of any modern software developer. It ensures data integrity, confidentiality, and authenticity. In Java, the Secure Sockets Layer (SSL) and Java Cryptography Architecture (JCA) provide developers the tools to implement secure communications and data protection. However, misuse of these tools can lead to vulnerabilities. This blog post will explore common Java cryptography mistakes and how to avoid them.
Table of Contents
- Understanding Cryptography
- Common Mistakes
- Failing to Use Strong Algorithms
- Poor Key Management
- Incorrect Initialization Vector (IV) Usage
- Insecure Random Number Generators
- Best Practices in Java Cryptography
- Further Reading and Resources
- Conclusion
Understanding Cryptography
Before diving into specific mistakes, it is important to understand what cryptography does. At its core, cryptography transforms data into a secure format. It can be classified into two main types:
- Symmetric Key Cryptography: Uses the same key for encryption and decryption.
- Asymmetric Key Cryptography: Utilizes a public key for encryption and a private key for decryption.
Java provides a rich set of cryptographic libraries to achieve secure communications and data storage, such as the javax.crypto
package and the java.security
package.
Common Mistakes
1. Failing to Use Strong Algorithms
Using outdated or weak algorithms can expose your data to security breaches. For example, DES (Data Encryption Standard) is now considered insecure due to its short key length.
Correct Approach: Use modern algorithms such as AES (Advanced Encryption Standard) which provides better security.
Here’s a simple example using AES encryption in Java:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class AESEncryption {
public byte[] encryptData(byte[] data) throws Exception {
// Generate a key for AES
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256); // specify key size
SecretKey secretKey = keyGen.generateKey();
// Create AES Cipher instance
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Encrypt data
return cipher.doFinal(data);
}
}
Why this Works
- The key size of 256 bits makes AES secure against current brute-force attacks.
- Proper usage of
Cipher.getInstance("AES")
ensures that we’re leveraging a well-vetted algorithm.
2. Poor Key Management
A common oversight is the lack of robust key management. Hardcoding keys in the source code or not rotating them regularly can lead to severe security risks. If an attacker gains access to the key, they can decrypt sensitive data.
Correct Approach: Utilize a secure key storage system such as a Key Management Service (KMS) or environment variables for sensitive keys.
Example of Reading a Key from Environment Variables
public class KeyStore {
public SecretKey getSecretKey() throws Exception {
String keyString = System.getenv("MY_SECRET_KEY");
byte[] keyBytes = keyString.getBytes();
return new SecretKeySpec(keyBytes, "AES");
}
}
Why this Works
- Environment variables allow for dynamic key management without hardcoding sensitive information.
- Externalizing the keys minimizes exposure in logs or in the source code.
3. Incorrect Initialization Vector (IV) Usage
An Initialization Vector (IV) is vital in block cipher encryption modes like CBC (Cipher Block Chaining). Using a static IV can lead to predictable patterns. Every encryption operation should have a unique IV to maintain security.
Correct Approach: Generate a new IV for each encryption operation and store it alongside the ciphertext.
Example of Proper IV Handling in AES
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
public class AESWithIV {
public byte[] encrypt(byte[] data, SecretKey secretKey) throws Exception {
// Generate a random IV
byte[] iv = new byte[16];
new SecureRandom().nextBytes(iv);
IvParameterSpec ivParams = new IvParameterSpec(iv);
// Create cipher
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey, ivParams);
// Encrypt data
byte[] encryptedData = cipher.doFinal(data);
// Combine IV and encrypted data
byte[] combined = new byte[iv.length + encryptedData.length];
System.arraycopy(iv, 0, combined, 0, iv.length);
System.arraycopy(encryptedData, 0, combined, iv.length, encryptedData.length);
return combined;
}
}
Why this Works
- A new random IV protects against replay attacks by ensuring each encryption is unique.
- Combining the IV with the encrypted data allows the decryption function to easily access the IV.
4. Insecure Random Number Generators
Using insecure random number generators can lead to vulnerabilities, particularly in the generation of cryptographic keys and IVs. The default Java Random
class does not provide adequate cryptographic security.
Correct Approach: Use SecureRandom
for all cryptographic purposes.
Example of Using SecureRandom for Key Generation
import java.security.SecureRandom;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class SecureKeyGenerator {
public SecretKey generateKey() throws Exception {
SecureRandom secureRandom = new SecureRandom();
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256, secureRandom); // Ensures secure key generation
return keyGen.generateKey();
}
}
Why this Works
SecureRandom
is designed to produce unpredictable values suitable for cryptography.- Proper key generation further enhances the security of your application.
Best Practices in Java Cryptography
- Use well-established libraries: Libraries such as BouncyCastle or Java's built-in JCA are preferred for implementing cryptographic algorithms.
- Regularly update dependencies: Ensure libraries and IDEs are up-to-date to mitigate any known vulnerabilities.
- Conduct security audits: Periodically review your codebase for any possible vulnerabilities related to cryptography.
- Educate Developers: Foster a culture of security awareness among team members on the importance of using appropriate cryptographic practices.
Further Reading and Resources
- Java Cryptography Architecture
- Cryptography Best Practices
Lessons Learned
Navigating Java cryptography can be daunting, but awareness of common mistakes can prevent vulnerabilities. By using strong algorithms, secure key management, dynamic IVs, and robust random number generators, you will enhance the overall security posture of your applications.
Implementing these principles will not only protect your system but also instill confidence in your users. Remember, cryptography is not just about implementing features; it's about securing trust.
With careful attention to the issues outlined above, you can avoid the pitfalls and leverage Java's cryptographic capabilities effectively. Stay curious, code securely!
Feel free to enhance your understanding of cryptographic practices by exploring additional resources and keeping up with the latest trends in security.